java coin介绍
在计算机上安装Java 7 SDK
安装Eclipse Indigo 3.7.1
您需要寻找适合您操作系统的捆绑软件。
在Eclipse工作区中,您需要在运行时中定义已安装的Java 7 JDK。 在工作台中,转到窗口>首选项> Java>已安装的JRE,然后添加Java 7主目录。
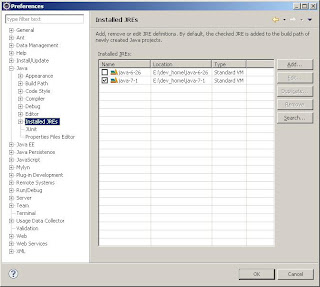
接下来,您需要在Java>编译器中将编译器级别设置为1.7。
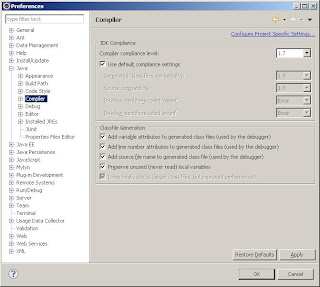
改进的文字
文字是固定值的源代码表示。
“在Java SE 7和更高版本中,数字文字中数字之间的任何位置都可以出现任何数量的下划线字符(_)。 此功能使您可以将数字文字中的数字组分开,这可以提高代码的可读性。” (来自Java教程 )
public class LiteralsExample { public static void main(String[] args) { System.out.println("With underscores: "); long creditCardNumber = 1234_5678_9012_3456L; long bytes = 0b11010010_01101001_10010100_10010010; System.out.println(creditCardNumber); System.out.println(bytes); System.out.println("Without underscores: "); creditCardNumber = 1234567890123456L; bytes = 0b11010010011010011001010010010010; System.out.println(creditCardNumber); System.out.println(bytes); }
}
注意文字中的下划线(例如1234_5678_9012_3456L)。 结果写入控制台:
With underscores:
1234567890123456
-764832622
Without underscores:
1234567890123456
-764832622
如您所见,下划线对值没有影响。 它们只是用来使代码更具可读性。
SafeVarargs
在JDK 7之前的版本中,调用某些varargs库方法时始终会收到未经检查的警告。 如果没有新的@SafeVarargs
批注,此示例将创建未经检查的警告。
public class SafeVarargsExample { @SafeVarargs static void m(List<string>... stringLists) { Object[] array = stringLists; List<integer> tmpList = Arrays.asList(42); array[0] = tmpList; // compiles without warnings String s = stringLists[0].get(0); // ClassCastException at runtime } public static void main(String[] args) { m(new ArrayList<string>()); } }</string></integer></string>
第3行中的新注释无助于在运行时解决烦人的ClassCastException
。 而且,它只能应用于静态方法和最终方法。 因此,我相信这不会有太大帮助。 Java的未来版本将对不安全的代码产生编译时错误,例如上面的示例中的代码。
钻石
在Java 6中,需要耐心地创建地图列表。 看这个例子:
public class DiamondJava6Example {public static void main(String[] args) {List<Map<Date, String>> listOfMaps = new ArrayList<Map<Date, String>>(); // type information twice!HashMap<Date, String> aMap = new HashMap<Date, String>(); // type information twiceaMap.put(new Date(), "Hello");listOfMaps.add(aMap);System.out.println(listOfMaps);}
}
正如你可以在3和4行转让的右侧看到你需要重复的类型信息的listOfMaps
变量还有的aMap
变量。 在Java 7中,这不再是必需的:
public class DiamondJava7Example {public static void main(String[] args) {List<Map<Date, String>> listOfMaps = new ArrayList<>(); // type information once!HashMap<Date, String> aMap = new HashMap<>(); // type information once!aMap.put(new Date(), "Hello");listOfMaps.add(aMap);System.out.println(listOfMaps);}
}
多渔获
在Java 7中,不需要为每个单个异常都包含catch子句,可以在一个子句中捕获多个异常。 您记得这样的代码:
public class HandleExceptionsJava6Example { public static void main(String[] args) { Class string; try { string = Class.forName("java.lang.String"); string.getMethod("length").invoke("test"); } catch (ClassNotFoundException e) { // do something } catch (IllegalAccessException e) { // do the same !! } catch (IllegalArgumentException e) { // do the same !! } catch (InvocationTargetException e) { // yeah, well, again: do the same! } catch (NoSuchMethodException e) { // ... } catch (SecurityException e) { // ... } }
}
从Java 7开始,您可以像这样编写它,这使我们的生活更加轻松:
public class HandleExceptionsJava7ExampleMultiCatch { public static void main(String[] args) { try { Class string = Class.forName("java.lang.String"); string.getMethod("length").invoke("test"); } catch (ClassNotFoundException | IllegalAccessException | IllegalArgumentException | InvocationTargetException | NoSuchMethodException | SecurityException e) { // do something, and only write it once!!! } }
}
switch语句中的字符串
由于Java 7可以在switch子句中使用字符串变量。 这是一个例子:
public class StringInSwitch { public void printMonth(String month) { switch (month) { case "April": case "June": case "September": case "November": case "January": case "March": case "May": case "July": case "August": case "December": default: System.out.println("done!"); } }
}
资源试穿
此功能确实有助于减少意外的运行时执行。 在Java 7中,可以使用所谓的try-with-resource子句,该子句在发生异常时自动关闭所有打开的资源。 看例子:
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream; public class TryWithResourceExample { public static void main(String[] args) throws FileNotFoundException { // Java 7 try-with-resource String file1 = "TryWithResourceFile.out"; try (OutputStream out = new FileOutputStream(file1)) { out.write("Some silly file content ...".getBytes()); ":-p".charAt(3); } catch (StringIndexOutOfBoundsException | IOException e) { System.out.println("Exception on operating file " + file1 + ": " + e.getMessage()); } // Java 6 style String file2 = "WithoutTryWithResource.out"; OutputStream out = new FileOutputStream(file2); try { out.write("Some silly file content ...".getBytes()); ":-p".charAt(3); } catch (StringIndexOutOfBoundsException | IOException e) { System.out.println("Exception on operating file " + file2 + ": " + e.getMessage()); } // Let's try to operate on the resources File f1 = new File(file1); if (f1.delete()) System.out.println("Successfully deleted: " + file1); else System.out.println("Problems deleting: " + file1); File f2 = new File(file2); if (f2.delete()) System.out.println("Successfully deleted: " + file2); else System.out.println("Problems deleting: " + file2); }
}
在第14行中,try-with-resource子句用于打开我们要操作的文件。 然后,第16行生成一个运行时异常。 请注意,我没有明确关闭资源。 当您使用try-with-resource时,这是自动完成的。 当您使用第21-30行中显示的Java 6等效项时,*不是*。
该代码会将以下结果写入控制台:
Exception on operating file TryWithResourceFile.out: String index out of range: 3
Exception on operating file WithoutTryWithResource.out: String index out of range: 3
Successfully deleted: TryWithResourceFile.out
Problems deleting: WithoutTryWithResource.out
就项目硬币而言就是这样。 在我眼中非常有用的东西。
参考:来自我们JCG合作伙伴 Niklas的“ Java 7:代码示例中的项目代币”。
相关文章 :
- Java 7功能概述
- 在Java 7中处理文件
- 具有Java 7中自动资源管理功能的GC
- Java 7:尝试资源
- Java SE 7、8、9 –推动Java前进
翻译自: https://www.javacodegeeks.com/2012/01/java-7-project-coin-in-code-examples.html
java coin介绍