目录
- react18 之 06 之 useReducer 结合 useContext使用 (实现全局状态管理、复杂组件交互逻辑、多个组件需要共享和更新某个状态)
- useReducer 结合 useContext使用 实现全局共享
- RootCom.jsx
- A.jsx
- ASon.jsx
- B.jsx
- C.jsx
- 执行效果
react18 之 06 之 useReducer 结合 useContext使用 (实现全局状态管理、复杂组件交互逻辑、多个组件需要共享和更新某个状态)
- 作用
-
- 全局状态管理:使用 useReducer 结合 useContext 来实现全局状态管理,避免了 props 的层层传递。
-
- 复杂的组件交互逻辑:当组件之间的交互逻辑较为复杂,包含多个状态和操作时,使用 useReducer 结合 useContext 可以更好地组织和管理这些状态和操作,使代码更清晰易维护。
-
- 状态共享和更新:当你需要在多个组件中共享某个状态,并且这些组件可能需要对该状态进行更新时,可以使用 useReducer 结合 useContext 来提供状态和更新函数,让组件可以访问和更新共享的状态。
useReducer 结合 useContext使用 实现全局共享
RootCom.jsx
import React, { useReducer } from 'react';
import A from "./A";
import B from "./B";
import C from "./C";
import { GlobalContext } from './globalContext';
const initState = {count:1
}
function reducer(state,action) {switch(action.type){case 'add': {return {...state,count: state.count + action.count}}case 'sub': {return {...state,count: state.count - action.count}}case 'mul': {return {...state,count: state.count * action.count}}default: {throw Error('Unknown action: ' + action.type);}}
}
export default function Rootcom() {const [state, dispatch] = useReducer(reducer, initState);const { count } = statereturn (<GlobalContext.Provider value={{state,dispatch}} ><div><div>root组件 count - {count}</div><A></A><B></B><C></C></div></GlobalContext.Provider>)
}
A.jsx
import React, { useContext } from 'react';
import ASon from "./ASon";
import { GlobalContext } from './globalContext';
export default function A(props) {const { state,dispatch } = useContext(GlobalContext)function addCount(){dispatch({type:'add',count:2})}return (<div style={{marginTop:'10px'}}>A count - {state.count} <button onClick={addCount}>A组件count+2</button><ASon></ASon></div>)
}
ASon.jsx
import React, { useContext } from 'react';
import { GlobalContext } from './globalContext';
export default function ASon(props) {const { state,dispatch } = useContext(GlobalContext)function addCount(){dispatch({type:'add',count:1})}return (<div style={{marginTop:'10px'}}>ASon count - {state.count} <button onClick={addCount}>ASon组件count+1</button></div>)
}
B.jsx
import React, { useContext } from 'react';
import { GlobalContext } from './globalContext';
export default function B(props) {const { state,dispatch } = useContext(GlobalContext)function subCount(){dispatch({type:'sub',count:1})}return (<div style={{marginTop:'10px'}}>B count-{state.count} <button onClick={subCount}>B组件count-1</button></div>)
}
C.jsx
import React, { useContext } from 'react';
import { GlobalContext } from './globalContext';
export default function C() {const { state,dispatch } = useContext(GlobalContext)function cmulCount(){dispatch({type:'mul',count: 2})}return (<div style={{marginTop:'10px'}}>C count - {state.count} <button onClick={cmulCount}>C组件count * 2</button></div>)
}
执行效果
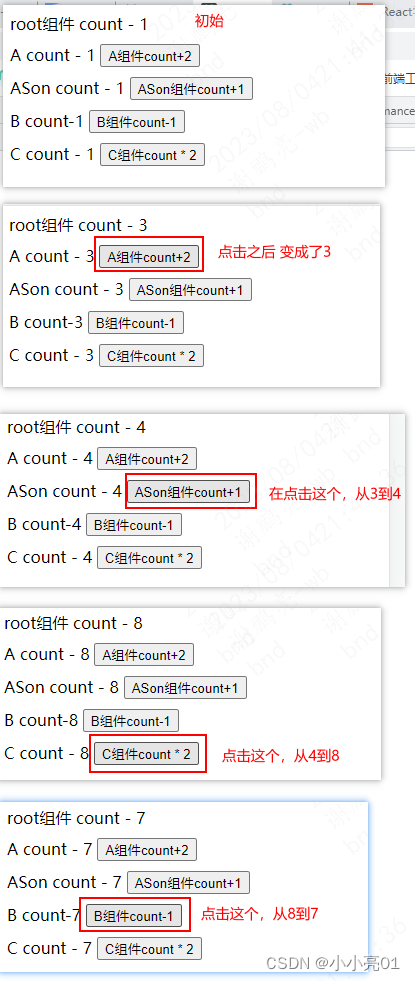