import * as THREE from "three"
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls'const width = window.innerWidth;
const height = window.innerHeight;
const scene = new THREE.Scene();
scene.background = new THREE.Color('#65762c');
const geometry = new THREE.SphereGeometry(50,50,50)
const material = new THREE.MeshLambertMaterial({color:0x00ffff,
})
const mesh = new THREE.Mesh(geometry,material);
scene.add(mesh)
const dirLight2 = new THREE.DirectionalLight(0xFFFFFF, 1);
dirLight2.position.set(100,100,100);
scene.add(dirLight2);
const ambient = new THREE.AmbientLight(0xffffff, 1)
scene.add(ambient)
const camera = new THREE.PerspectiveCamera(45,width/height,1,1000)
camera.position.set(100,200,250);
camera.lookAt(0,0,0)
const renderer = new THREE.WebGLRenderer();
renderer.setSize(width,height);
document.body.appendChild(renderer.domElement);
function render(){renderer.render(scene,camera);requestAnimationFrame(render);
}render();
const controls = new OrbitControls(camera, renderer.domElement);
controls.addEventListener('change',function(){renderer.render(scene,camera);
})
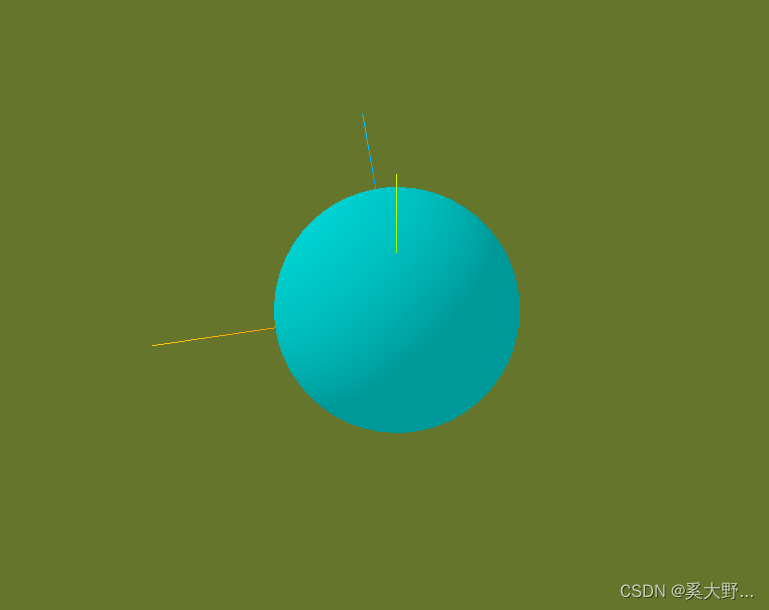