组合式API
setup选项
执行时机:比beforeCreate早
不能使用this
数据和函数需要return才能应用
标准写法
<script>export default {setup() {const message = "vue32"const logMessage = () => {console.log(message)}return {message,logMessage}}console.log('beforeCreate')}}
</script>
简化版(语法糖)
<script setup>const message = "vue3"const logMessage = () => {console.log(message)}
</script>
reactive(对象类型)
作用:接受应该对象类型的数据,返回一个响应式的对象
<script setup>import {reactive} from 'vue'const state = reactive({count: 100})const setCount = () => {state.count++}
</script><template><div><div>{{state.count}}</div><button @click="setCount">+1</button></div>
</template>
ref (简单类型和对象类型都行)
作用:接受一个对象类型或简单类型的数据,返回一个响应式的对象
在脚本中需要通过.value,在template中不用
<script setup>import {ref} from 'vue'const count = ref(0)const setCount = () => {count.value++}
</script><template><div><div>{{count}}</div><button @click="setCount">+1</button></div>
</template>
computed
<script setup>import {computed,ref} from 'vue'const list = ref([1, 2, 3, 4, 5, 6, 7, 8])const computedList = computed(() => {return list.value.filter(item => item > 2)})const addfn = () => {list.value.push(12)}
</script><template><div><div>{{list}}</div><div>{{computedList}}</div><button @click="addfn" type="button">修改</button></div>
</template>
watch
监视事件
<script setup>import {ref,watch} from 'vue'const count = ref(0)const nickname = ref('张三')const changeCount = () => {count.value++}const changeName = () => {nickname.value = 'lisi'}// 一个对象watch(count,(newValue,oldValue)=>{console.log(newValue,oldValue)})// 多个对象watch([count,nickname],(newValue,oldValue)=>{console.log(newValue[0],oldValue[0])})
</script><template><div><div>{{count}}</div><button @click="changeCount" type="button">修改</button><div>{{nickname}}</div><button @click="changeName" type="button">修改</button></div>
</template>
mounted
渲染完成后执行,操作DOM
created
初始化渲染请求
父子组件
父传子
子组件
<template><div class='son'>son of api-{{car}}</div>
</template><script>const props = defineProps({car: String})
</script><style scoped>.son {border: 1px solid #000;padding: 30px;}
</style>
父组件
<script setup>// 局部注册import SonCom from'@/components/son-com.vue'
</script><template><div><SonCom car="1"></SonCom></div>
</template>
子传父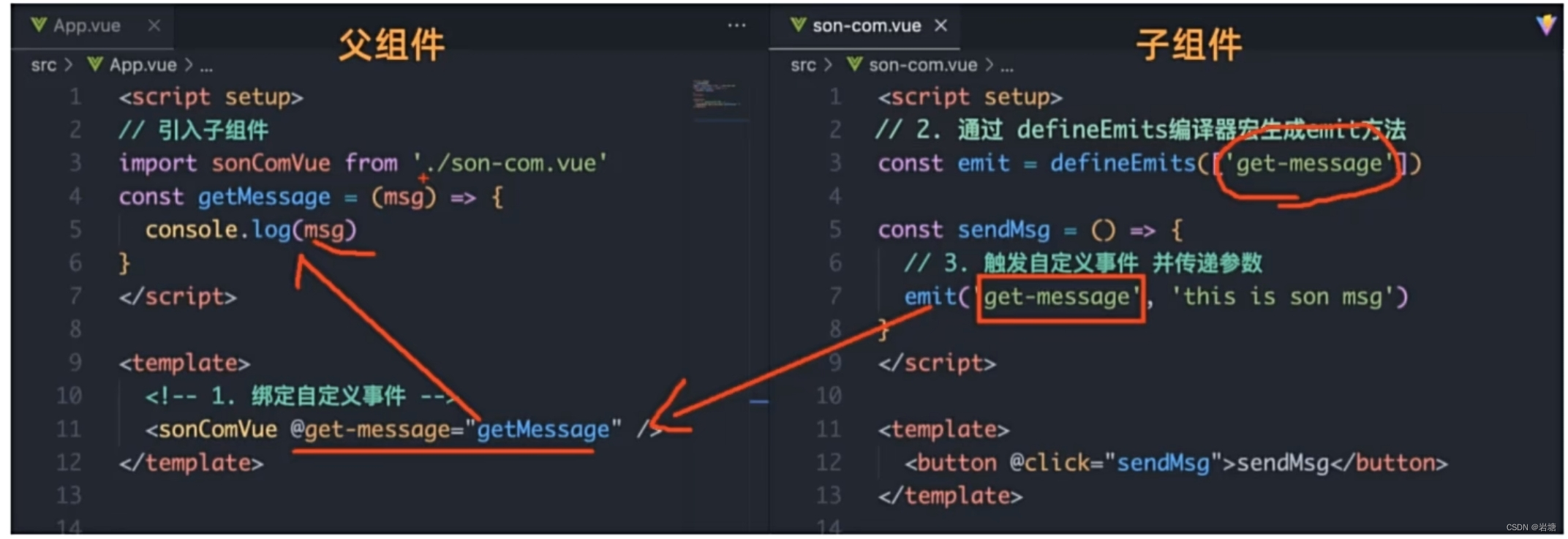
defineExpose(子传父)
父组件
<script setup>
import { ref, onMounted } from 'vue'
import testCom from './components/test-com.vue'
const input = ref(null)
onMounted(() => {})
const clickFn = () => {input.value.focus()
}
const testRef = ref(null)
const getCom = () => {console.log(testRef.value.name)testRef.value.sayHi()
}</script><template><div><input type="text" ref="input" /><button @click="clickFn">聚焦</button><testCom ref="testRef"></testCom><button @click="getCom">获取组件</button></div>
</template><style scoped></style>
子组件
<script setup>
const name = "test";
const sayHi = () => {console.log("hi");
}
defineExpose({name,sayHi,
})
</script>
<template><div>test demo-{{ name }}</div>
</template>
外部组件
axios
类似ajax,用于后台传值
echarts
画图,一般在mounted函数中实现