前言
新手刚开始学习 TypeScript 时,往往会对 type 和 interface 的使用场景和方式感到困惑。因此,本文旨在总结 type 和 interface 的概念和用法。
一、概念
-
type:类型别名
-
概念:允许为一个或多个数据类型(例如 string、number 等)创建一个别名。
-
举例:
type dataType = number
(为单个类型创建别名)type dataType = number | string | tuple
-
-
interface:接口
- 概念:定义参数或方法的数据类型。
二、两者的异同
1. 相同点
type 和 interface 都可用于描述对象或函数。
typescriptCopy code
// Interface
interface User {name: string;age: number;
}interface SetUser {(name: string, age: number): void;
}// Type
type User = {name: string;age: number;
}type SetUser = (name: string, age: number) => void;
2. 异同点
- 类型别名可用于联合类型、元组类型、基本类型(原始值),而 interface 不支持。
typescriptCopy code
type PartialPointX = { x: number };
type PartialPointY = { y: number };type PartialPoint = PartialPointX | PartialPointY;
type Data = [PartialPointX, PartialPointY];
type Name = Number;let div = document.createElement('div');
type B = typeof div;
- Interface 可多次定义并被视为合并所有声明成员,而 type 不支持。
typescriptCopy code
interface Point {x: number;
}
interface Point {y: number;
}
const point: Point = { x: 1, y: 2 };
- Type 可以使用
in
关键字生成映射类型,但 interface 不支持。
typescriptCopy code
type Keys = 'firstname' | 'surname';type DudeType = {[key in Keys]: string;
};
const test: DudeType = {firstname: 'Pawel',surname: 'Grzybek',
};
- 默认导出方式不同。
typescriptCopy code
export default interface Config {name: string;
}type Config2 = { name: string };
export default Config2;
- Type 支持其他高级操作,如联合类型、映射类型、泛型、元组等。
typescriptCopy code
type StringOrNumber = string | number;
type Text = string | { text: string };
type NameLookup = Dictionary<string, Person>;
type Callback<T> = (data: T) => void;
type Pair<T> = [T, T];
type Coordinates = Pair<number>;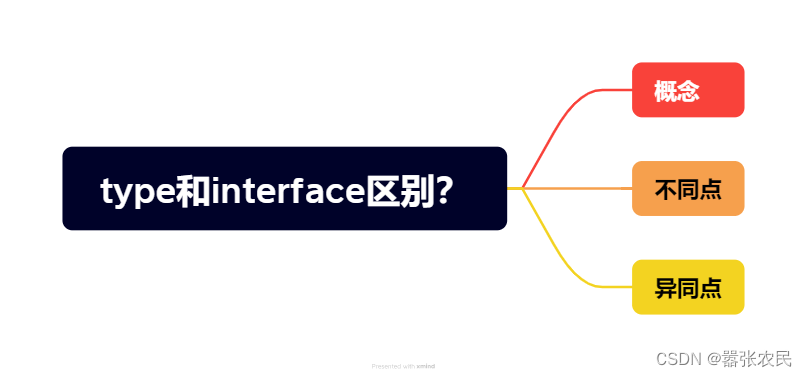type Tree<T> = T | { left: Tree<T>, right: Tree<T> };
总结
通常情况下,如果能用 interface 实现,就使用 interface;如果不能,再考虑使用 type。