效果展示
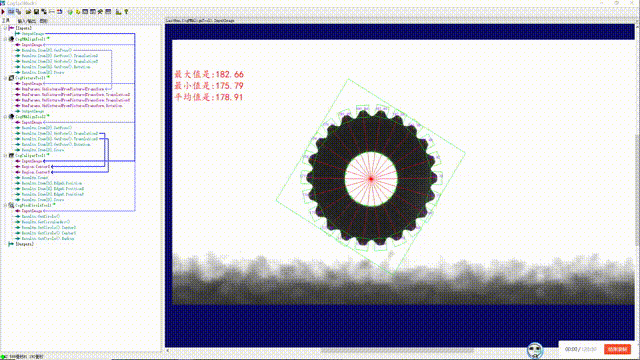
一、题目要求
求出最大值,最小值,平均值
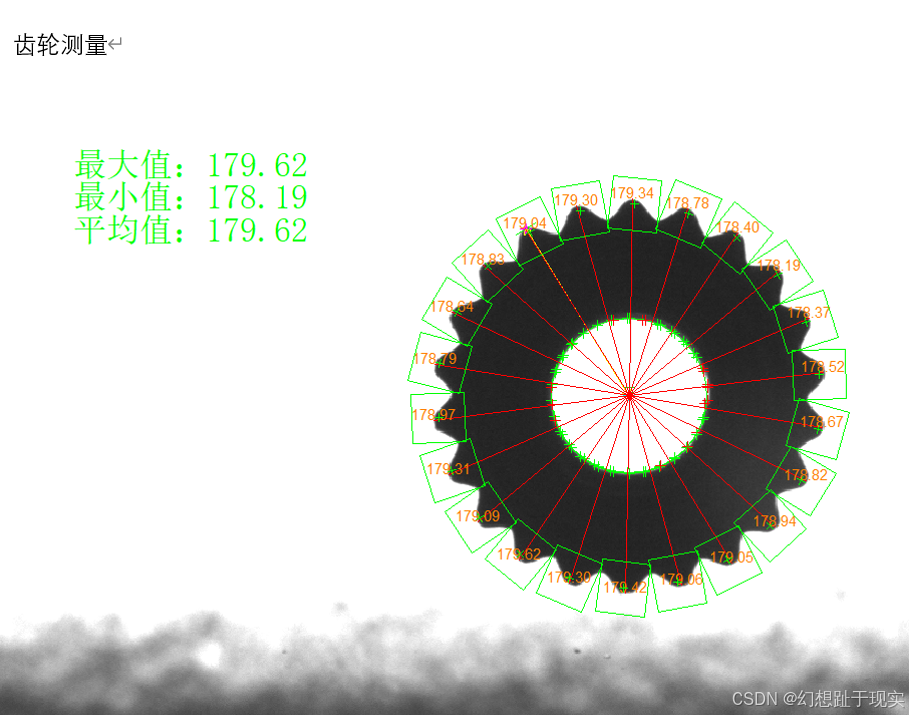
二、分析
1.首先要进行模板匹配
2.划清匹配范围
3.匹配小三角的模板匹配
4.卡尺
5.用找圆工具
工具
1.CogPMAlignTool
2.CogCaliperTool
3.CogFindCircleTool
4.CogFixtureTool
三、模板匹配工具
1.搜索区域
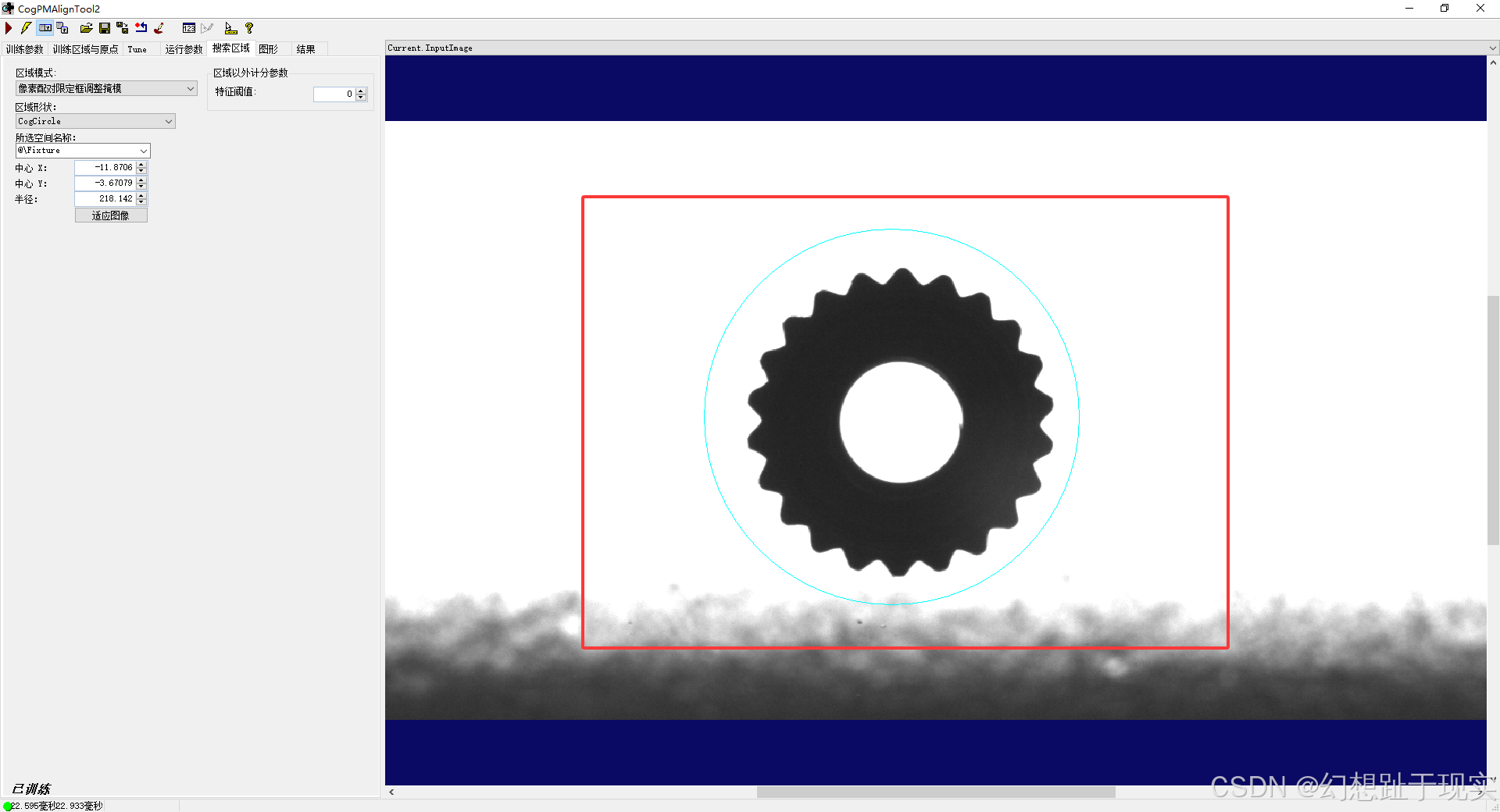
2.齿轮匹配
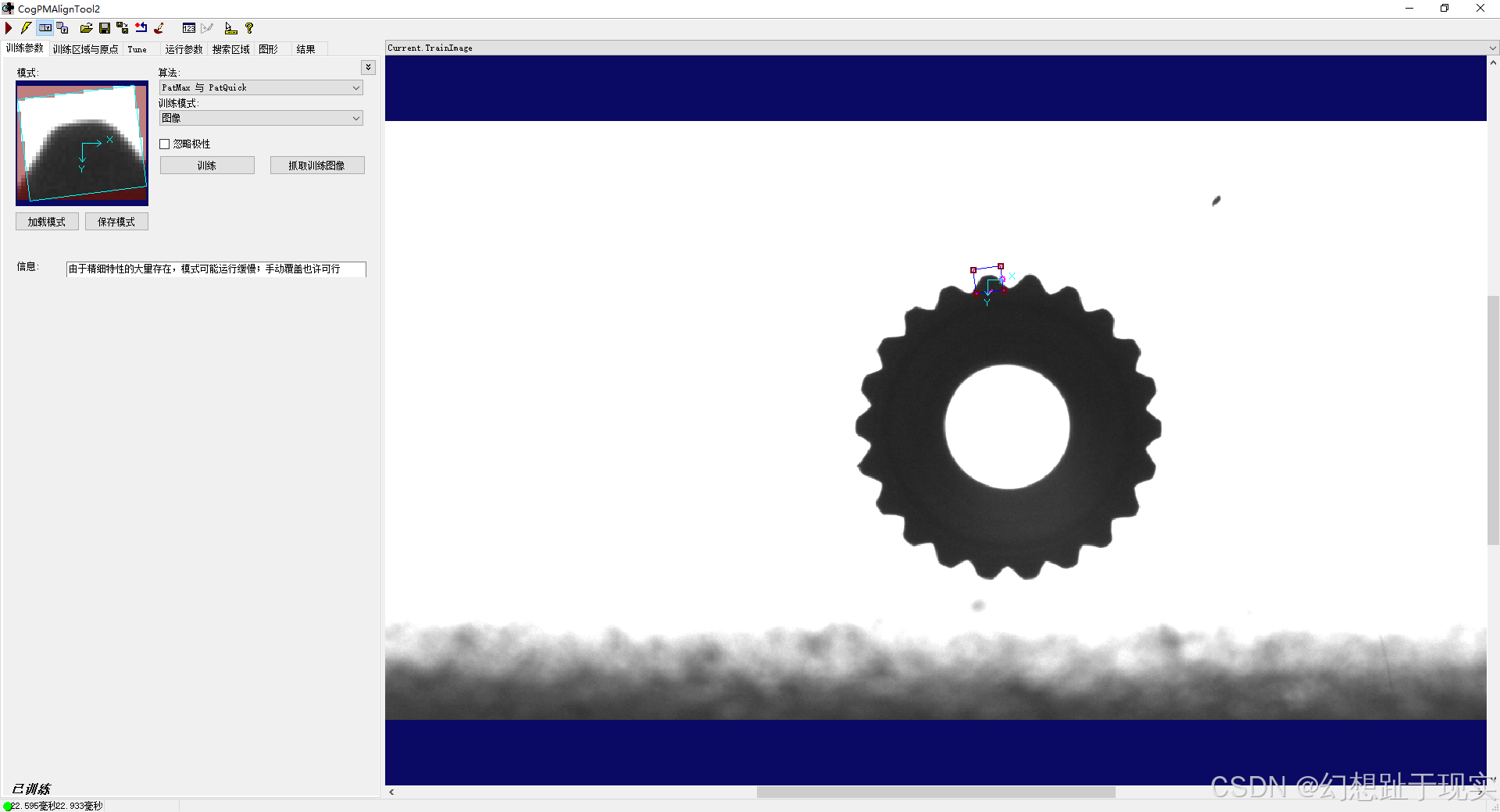
3.设置参数
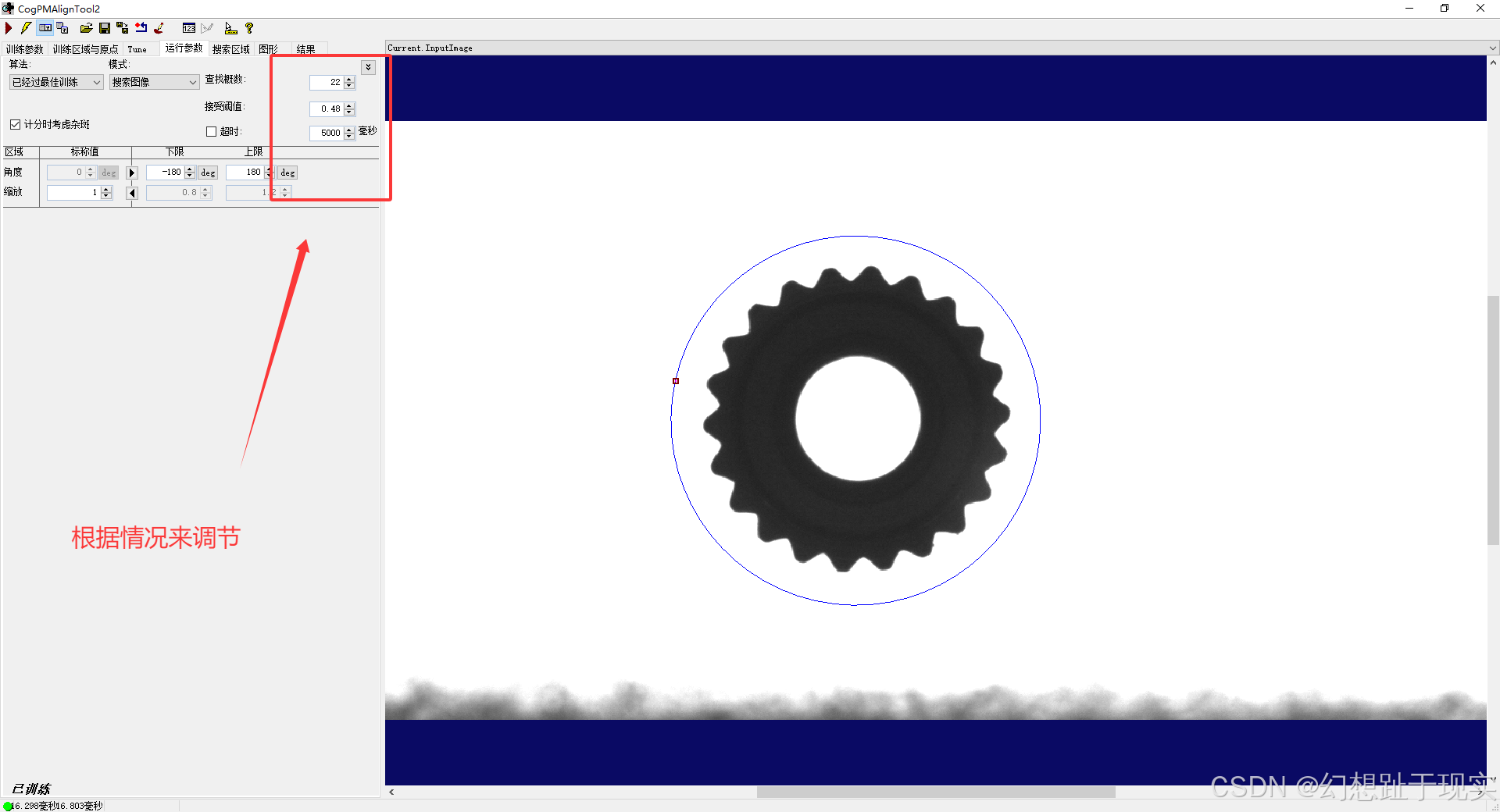
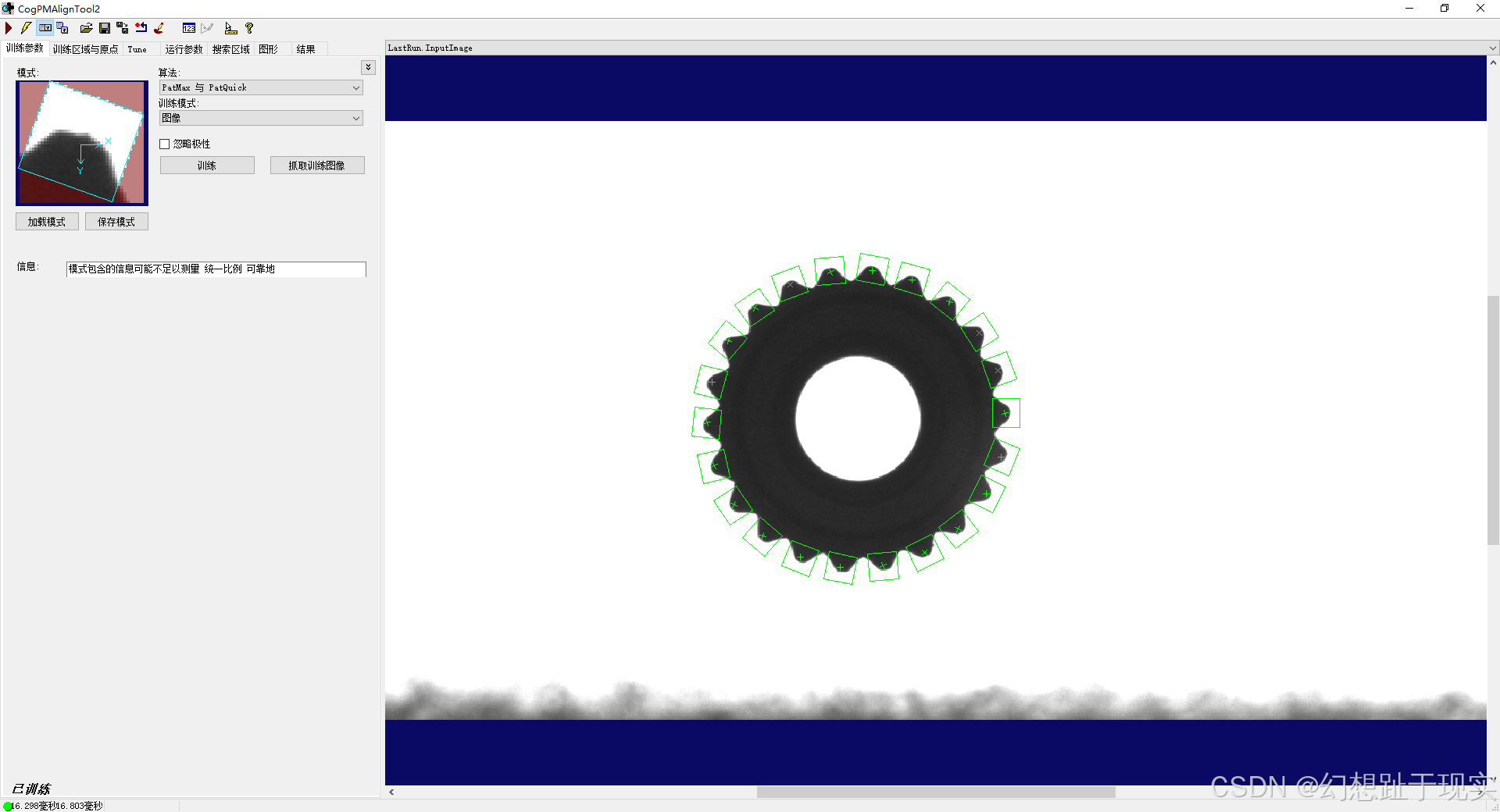
4.卡尺设置
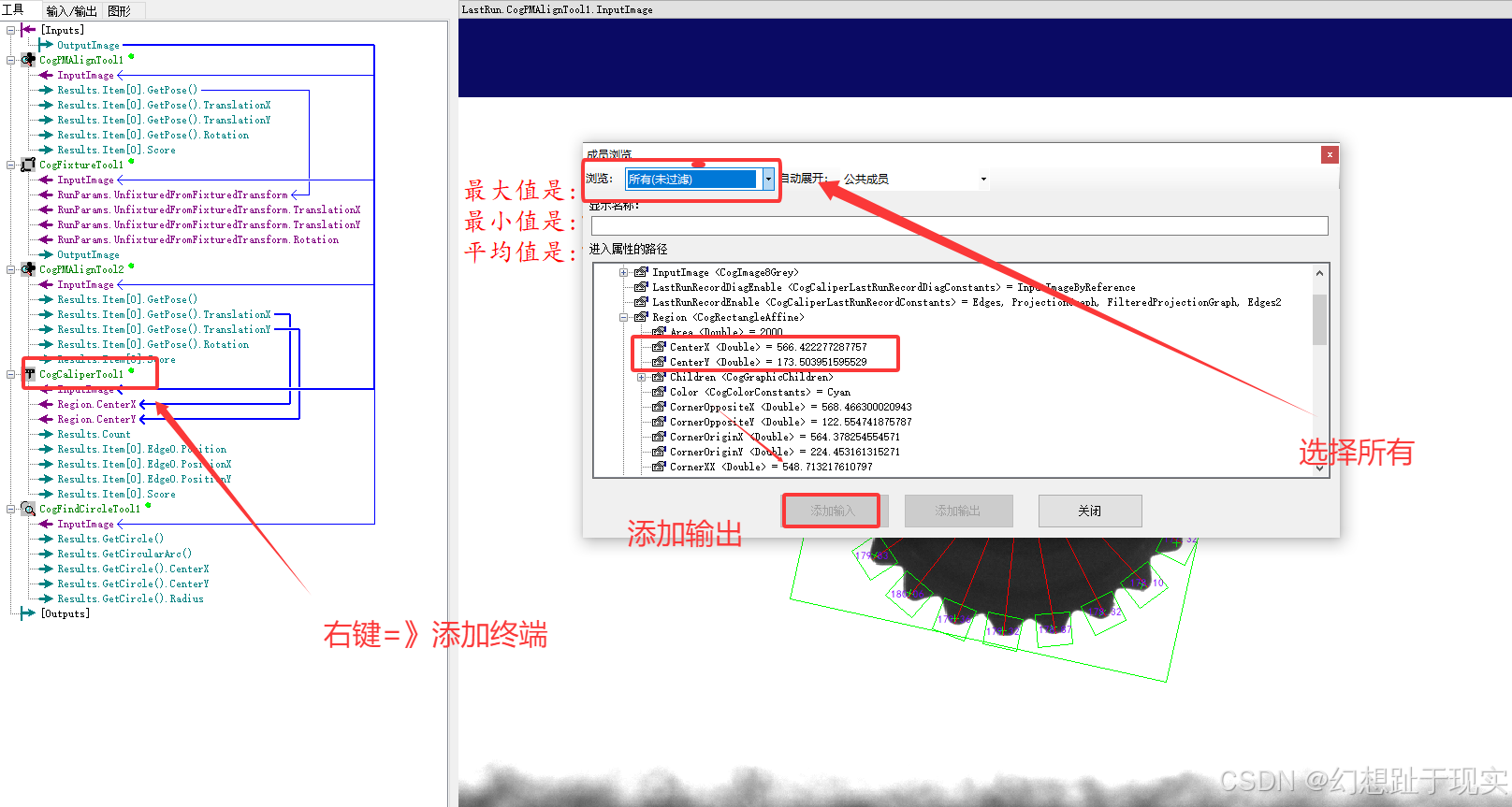
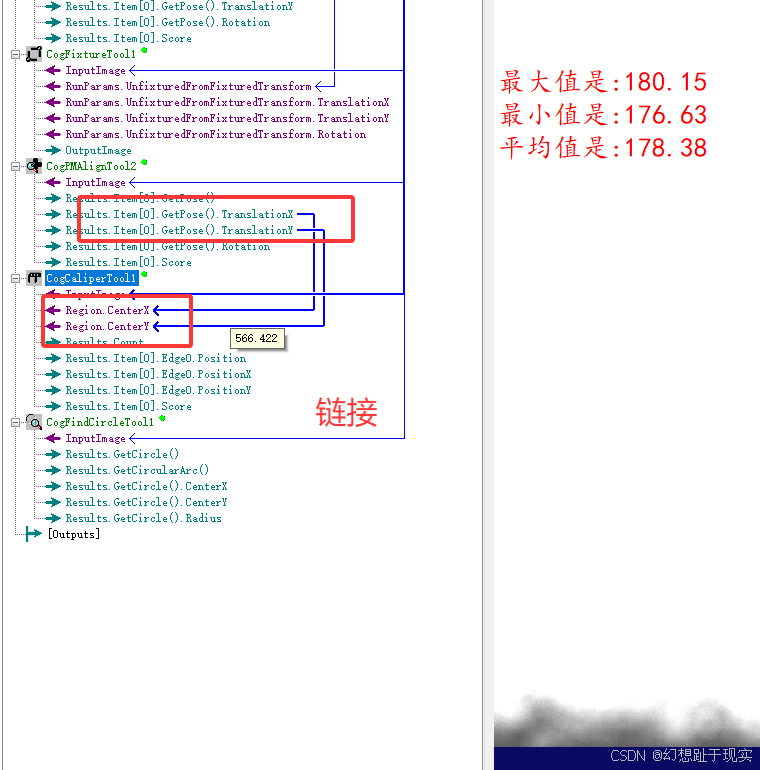
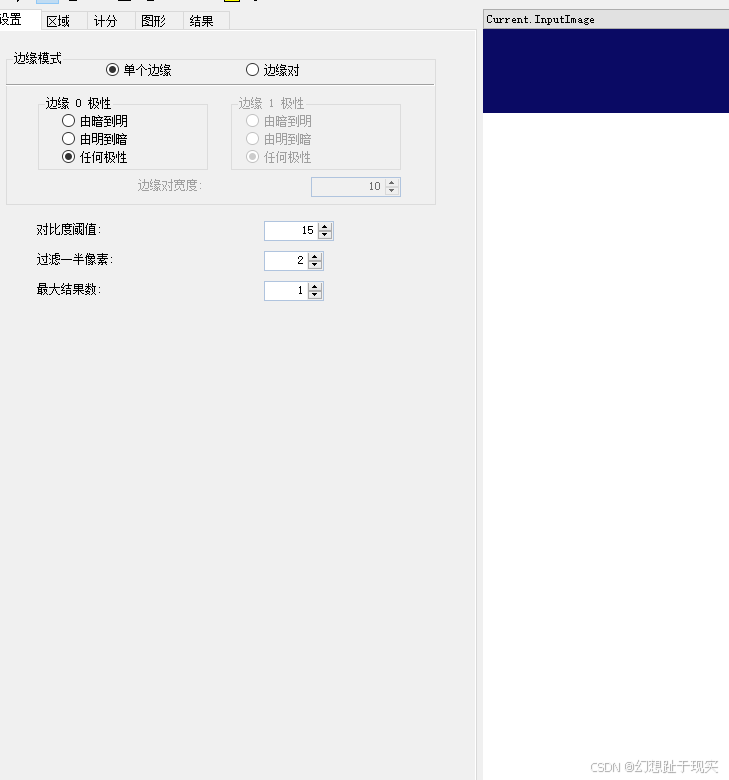
5.找圆工具
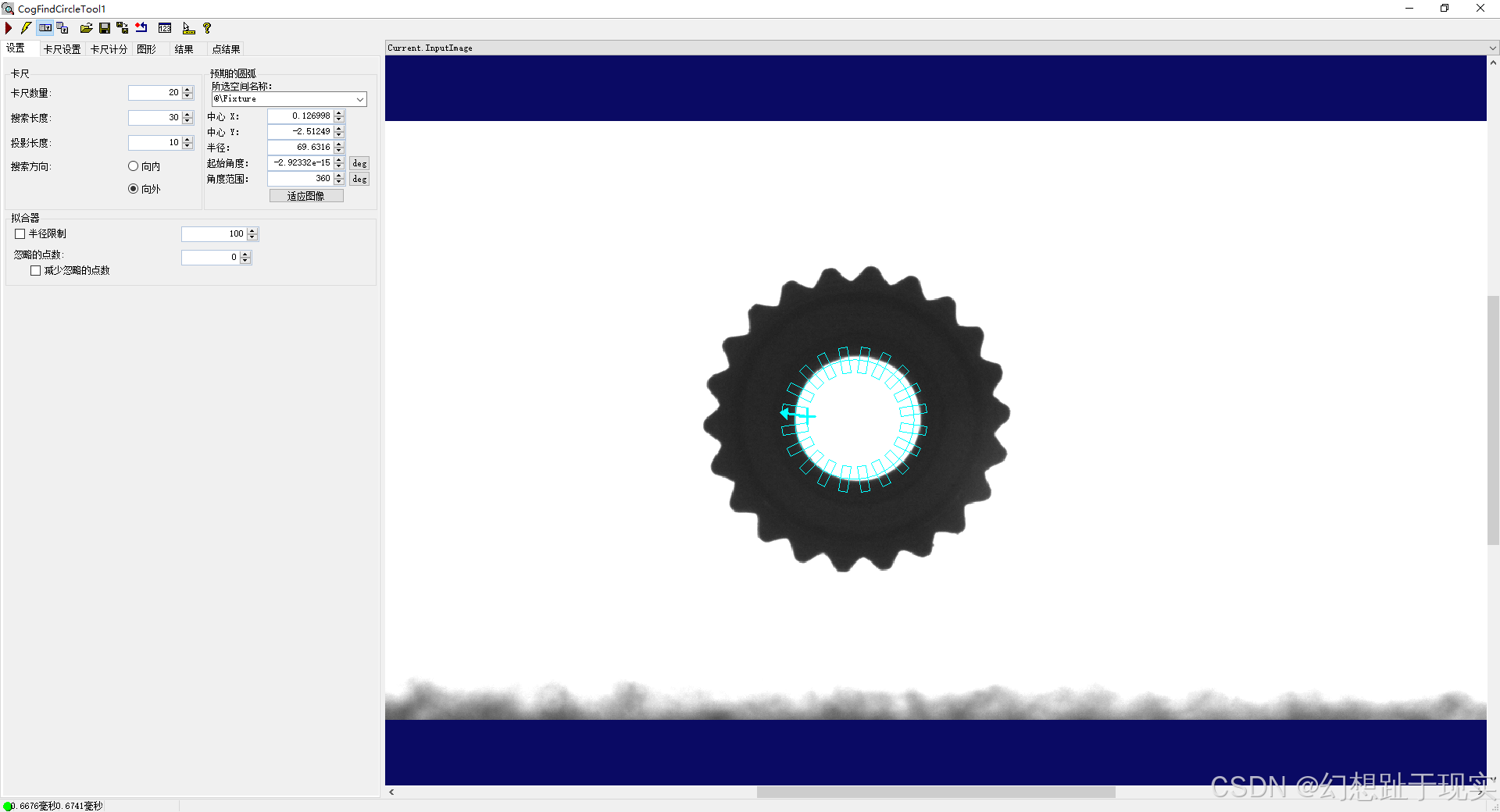
四、代码分析
1.声明集合,文字显示工具,线段
CogGraphicCollection dt = new CogGraphicCollection();CogGraphicLabel label;CogLineSegment line;
2.将工具进行实例化
dt.Clear();CogPMAlignTool pma = mToolBlock.Tools["CogPMAlignTool2"]as CogPMAlignTool;CogCaliperTool caliper = mToolBlock.Tools["CogCaliperTool1"]as CogCaliperTool;CogFindCircleTool find = mToolBlock.Tools["CogFindCircleTool1"]as CogFindCircleTool;
3.解决实例化问题
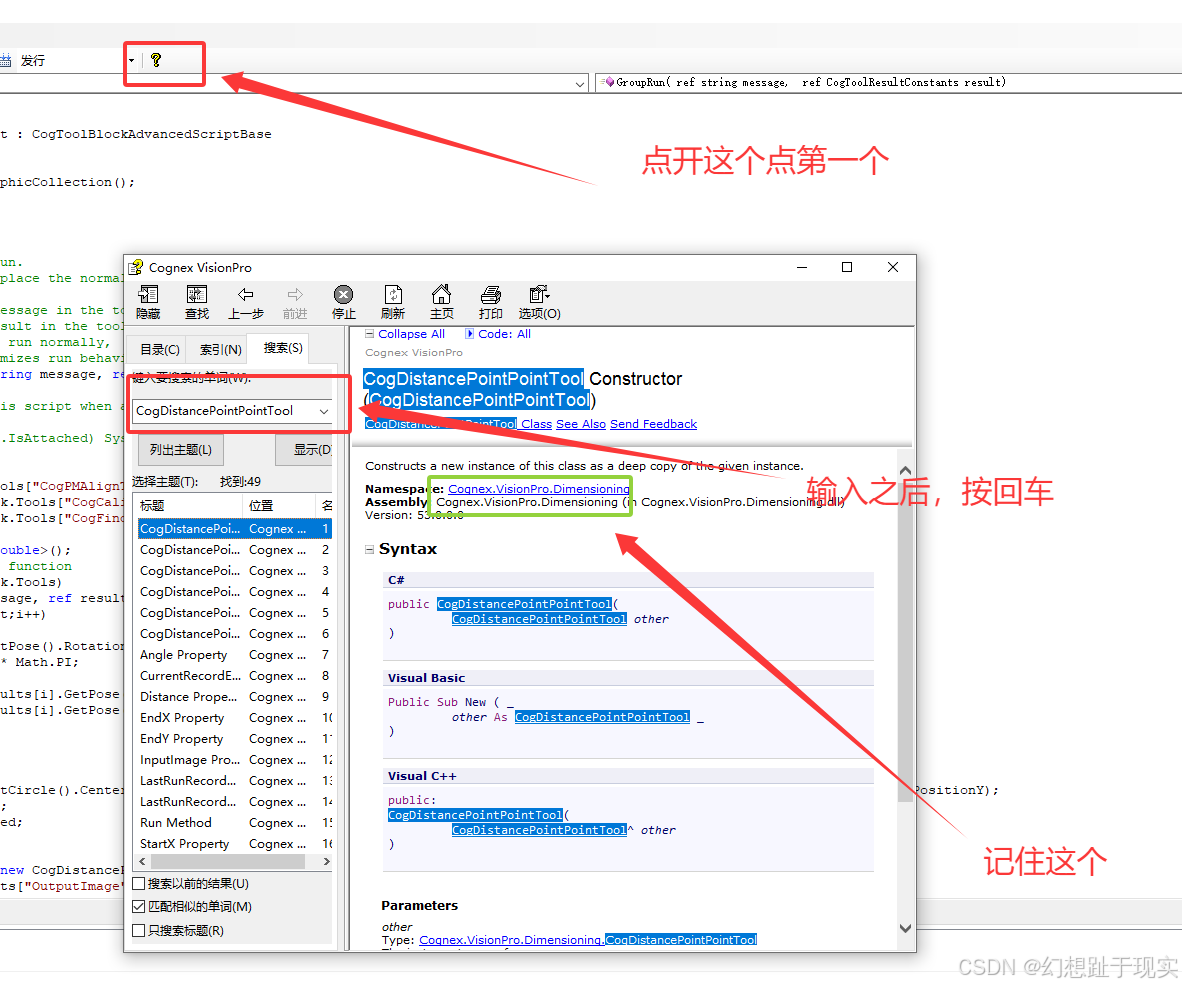
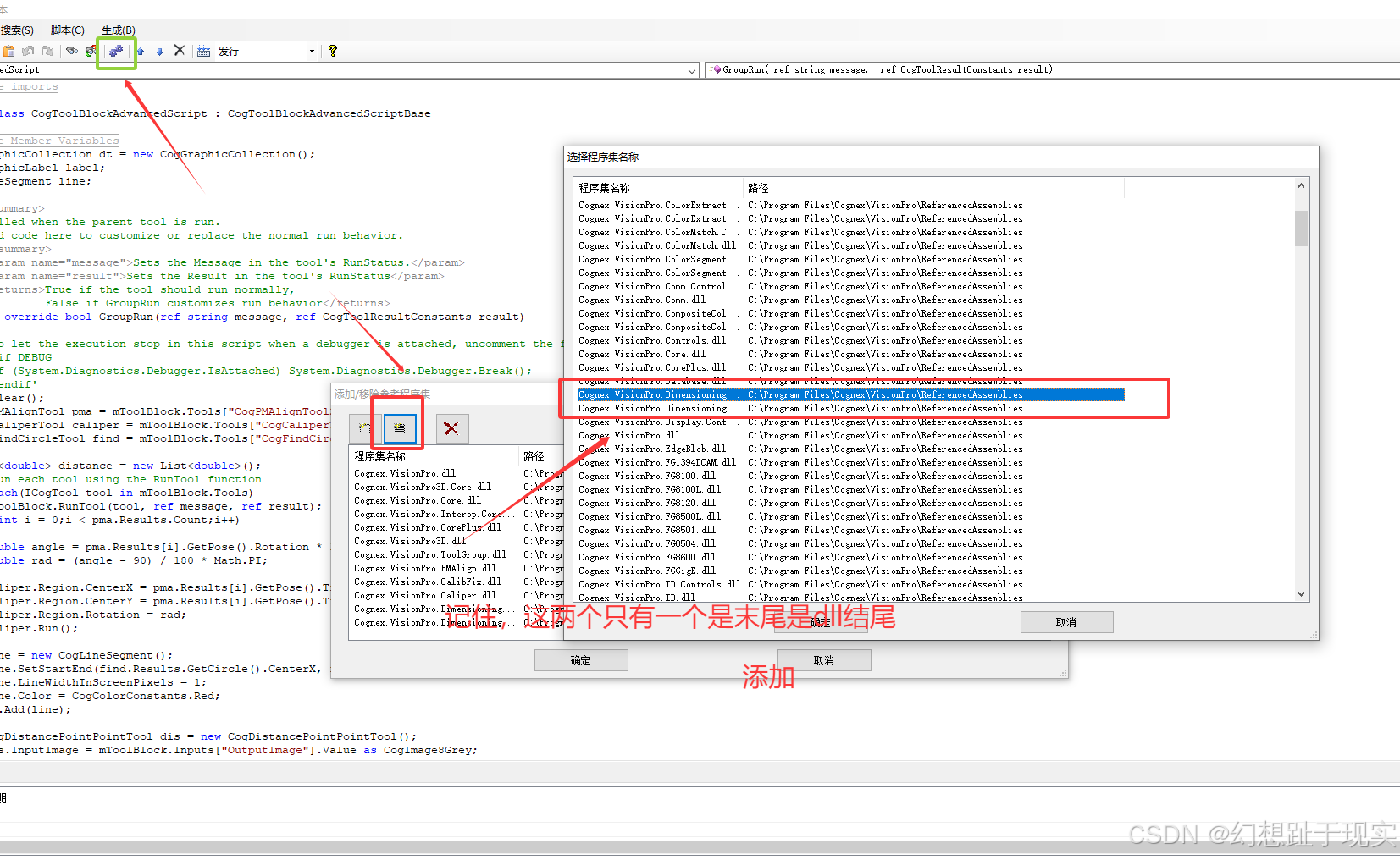
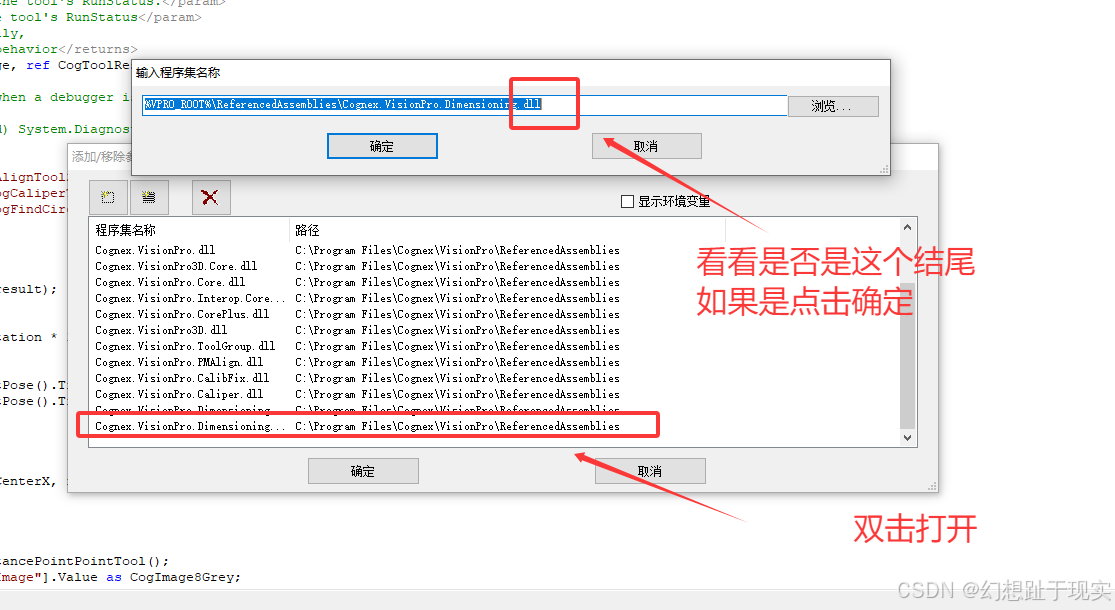
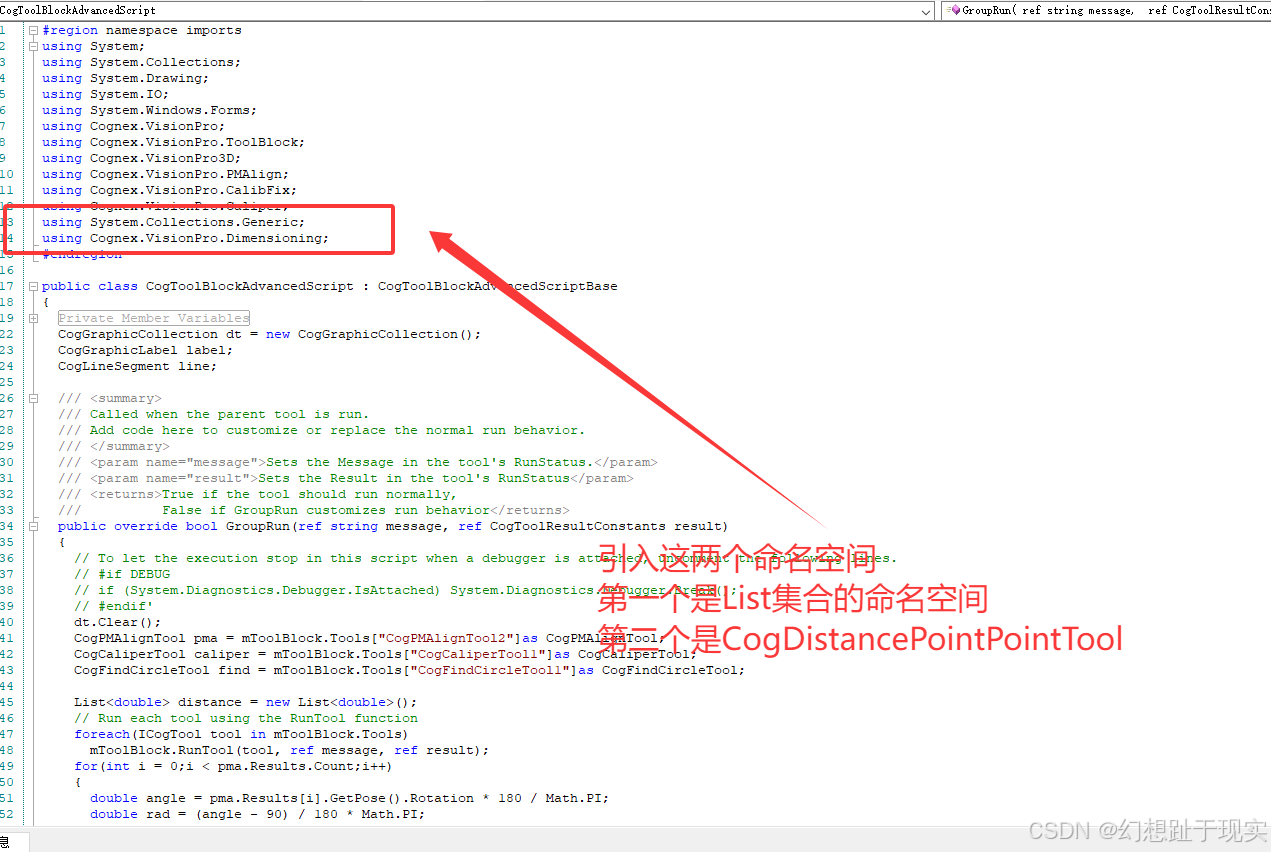
4.声明List 弧度转角度(角度转弧度)遍历卡尺数量
公式: 弧度=角度/180*Math.PI
角度=弧度*180/MathPI
List<double> distance = new List<double>();for(int i = 0;i < pma.Results.Count;i++){double angle = pma.Results[i].GetPose().Rotation * 180 / Math.PI;double rad = (angle - 90) / 180 * Math.PI;caliper.Region.CenterX = pma.Results[i].GetPose().TranslationX;caliper.Region.CenterY = pma.Results[i].GetPose().TranslationY;caliper.Region.Rotation = rad;caliper.Run();
5.卡尺找到的地方声明线段
line = new CogLineSegment();line.SetStartEnd(find.Results.GetCircle().CenterX, find.Results.GetCircle().CenterY, caliper.Results[0].Edge0.PositionX, caliper.Results[0].Edge0.PositionY);line.LineWidthInScreenPixels = 1;line.Color = CogColorConstants.Red;dt.Add(line);
6.实例化点到点的距离工具 链接
CogDistancePointPointTool dis = new CogDistancePointPointTool();dis.InputImage = mToolBlock.Inputs["OutputImage"].Value as CogImage8Grey;dis.StartX = find.Results.GetCircle().CenterX;dis.StartY = find.Results.GetCircle().CenterY;dis.EndX = caliper.Results[0].Edge0.PositionX;dis.EndY = caliper.Results[0].Edge0.PositionY;dis.Run();distance.Add(dis.Distance);
7.放置每个角的长度位置
label = new CogGraphicLabel();label.Font = new Font("楷体", 8);label.Color = CogColorConstants.Purple;label.SetXYText(caliper.Results[0].Edge0.PositionX, caliper.Results[0].Edge0.PositionY, dis.Distance.ToString("F2"));dt.Add(label);
五、找出最大,最小,平均值
double Max = 0;double Small = distance[0];double total = 0;double average;for(int i = 0;i < distance.Count;i++){if(distance[i] > Max){Max = distance[i];}if(distance[i] < Small){Small = distance[i];}total += distance[i];}average = total / distance.Count;CogGraphicLabel label1 = new CogGraphicLabel();CogGraphicLabel label2 = new CogGraphicLabel();CogGraphicLabel label3 = new CogGraphicLabel();label1.SetXYText(100, 100, "最大值是:" + Max.ToString("F2"));label2.SetXYText(100, 130, "最小值是:" + Small.ToString("F2"));label3.SetXYText(100, 160, "平均值是:" + average.ToString("F2"));label1.Color = CogColorConstants.Red;label1.Font = new Font("楷体", 20);label2.Color = CogColorConstants.Red;label2.Font = new Font("楷体", 20);label3.Color = CogColorConstants.Red;label3.Font = new Font("楷体", 20);dt.Add(label1);dt.Add(label2);dt.Add(label3);
六、实现在图片工具图上
foreach(ICogGraphic s in dt){mToolBlock.AddGraphicToRunRecord(s, lastRecord, "CogPMAlignTool1.InputImage", "");}
七、愚公搬代码
#region namespace imports
using System;
using System.Collections;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using Cognex.VisionPro;
using Cognex.VisionPro.ToolBlock;
using Cognex.VisionPro3D;
using Cognex.VisionPro.PMAlign;
using Cognex.VisionPro.CalibFix;
using Cognex.VisionPro.Caliper;
using System.Collections.Generic;
using Cognex.VisionPro.Dimensioning;
#endregionpublic class CogToolBlockAdvancedScript : CogToolBlockAdvancedScriptBase
{#region Private Member Variablesprivate Cognex.VisionPro.ToolBlock.CogToolBlock mToolBlock;#endregionCogGraphicCollection dt = new CogGraphicCollection();CogGraphicLabel label;CogLineSegment line;/// <summary>/// Called when the parent tool is run./// Add code here to customize or replace the normal run behavior./// </summary>/// <param name="message">Sets the Message in the tool's RunStatus.</param>/// <param name="result">Sets the Result in the tool's RunStatus</param>/// <returns>True if the tool should run normally,/// False if GroupRun customizes run behavior</returns>public override bool GroupRun(ref string message, ref CogToolResultConstants result){// To let the execution stop in this script when a debugger is attached, uncomment the following lines.// #if DEBUG// if (System.Diagnostics.Debugger.IsAttached) System.Diagnostics.Debugger.Break();// #endif'dt.Clear();CogPMAlignTool pma = mToolBlock.Tools["CogPMAlignTool2"]as CogPMAlignTool;CogCaliperTool caliper = mToolBlock.Tools["CogCaliperTool1"]as CogCaliperTool;CogFindCircleTool find = mToolBlock.Tools["CogFindCircleTool1"]as CogFindCircleTool;List<double> distance = new List<double>();// Run each tool using the RunTool functionforeach(ICogTool tool in mToolBlock.Tools)mToolBlock.RunTool(tool, ref message, ref result);for(int i = 0;i < pma.Results.Count;i++){double angle = pma.Results[i].GetPose().Rotation * 180 / Math.PI;double rad = (angle - 90) / 180 * Math.PI;caliper.Region.CenterX = pma.Results[i].GetPose().TranslationX;caliper.Region.CenterY = pma.Results[i].GetPose().TranslationY;caliper.Region.Rotation = rad;caliper.Run();line = new CogLineSegment();line.SetStartEnd(find.Results.GetCircle().CenterX, find.Results.GetCircle().CenterY, caliper.Results[0].Edge0.PositionX, caliper.Results[0].Edge0.PositionY);line.LineWidthInScreenPixels = 1;line.Color = CogColorConstants.Red;dt.Add(line);CogDistancePointPointTool dis = new CogDistancePointPointTool();dis.InputImage = mToolBlock.Inputs["OutputImage"].Value as CogImage8Grey;dis.StartX = find.Results.GetCircle().CenterX;dis.StartY = find.Results.GetCircle().CenterY;dis.EndX = caliper.Results[0].Edge0.PositionX;dis.EndY = caliper.Results[0].Edge0.PositionY;dis.Run();distance.Add(dis.Distance);label = new CogGraphicLabel();label.Font = new Font("楷体", 8);label.Color = CogColorConstants.Purple;label.SetXYText(caliper.Results[0].Edge0.PositionX, caliper.Results[0].Edge0.PositionY, dis.Distance.ToString("F2"));dt.Add(label);}double Max = 0;double Small = distance[0];double total = 0;double average;for(int i = 0;i < distance.Count;i++){if(distance[i] > Max){Max = distance[i];}if(distance[i] < Small){Small = distance[i];}total += distance[i];}average = total / distance.Count;CogGraphicLabel label1 = new CogGraphicLabel();CogGraphicLabel label2 = new CogGraphicLabel();CogGraphicLabel label3 = new CogGraphicLabel();label1.SetXYText(100, 100, "最大值是:" + Max.ToString("F2"));label2.SetXYText(100, 130, "最小值是:" + Small.ToString("F2"));label3.SetXYText(100, 160, "平均值是:" + average.ToString("F2"));label1.Color = CogColorConstants.Red;label1.Font = new Font("楷体", 20);label2.Color = CogColorConstants.Red;label2.Font = new Font("楷体", 20);label3.Color = CogColorConstants.Red;label3.Font = new Font("楷体", 20);dt.Add(label1);dt.Add(label2);dt.Add(label3);return false;}#region When the Current Run Record is Created/// <summary>/// Called when the current record may have changed and is being reconstructed/// </summary>/// <param name="currentRecord">/// The new currentRecord is available to be initialized or customized.</param>public override void ModifyCurrentRunRecord(Cognex.VisionPro.ICogRecord currentRecord){}#endregion#region When the Last Run Record is Created/// <summary>/// Called when the last run record may have changed and is being reconstructed/// </summary>/// <param name="lastRecord">/// The new last run record is available to be initialized or customized.</param>public override void ModifyLastRunRecord(Cognex.VisionPro.ICogRecord lastRecord){foreach(ICogGraphic s in dt){mToolBlock.AddGraphicToRunRecord(s, lastRecord, "CogPMAlignTool1.InputImage", "");}}#endregion#region When the Script is Initialized/// <summary>/// Perform any initialization required by your script here/// </summary>/// <param name="host">The host tool</param>public override void Initialize(Cognex.VisionPro.ToolGroup.CogToolGroup host){// DO NOT REMOVE - Call the base class implementation first - DO NOT REMOVEbase.Initialize(host);// Store a local copy of the script hostthis.mToolBlock = ((Cognex.VisionPro.ToolBlock.CogToolBlock)(host));}#endregion}