一、作业

代码:
#include <iostream>using namespace std;using datatype = int; //类型重命名
#define MAX 2 //宏定义
//结构体定义
struct Sqlist
{
private:datatype *data; //顺序表数组int size = 0; //数组大小int len = 0; //数组实际长度
public://初始化函数void init(int s){size = s; //当前数组的最大容量data = new datatype(size); //在堆区申请一个顺序表容量为size}//判空函数bool empty();//判满函数bool full();//添加数据函数bool add(datatype e);//当前顺序表实际长度int length();//任意位置插入bool insert_pos(int pos,datatype e);//任意位置删除bool delete_pos(int pos);//访问容器中任意一个元素 atdatatype &at(int index){return data[index-1];}//展示数组所有内容void output(){cout<<"顺序表中数组如下:"<<endl;for(int i=0;i<len;i++){cout<<data[i]<<" ";}cout<<endl;}//二倍扩容void expend();
};
/*****************定义结构体内公共函数******************/
int Sqlist::length()
{return len;
}
bool Sqlist::empty()
{int llen = length();if(llen==0){cout<<"顺序表为空"<<endl;return 1;}return 0;
}
bool Sqlist::full()
{int llen = length();if(llen==size){cout<<"顺序表已满"<<endl;return 1;}return 0;
}
bool Sqlist::add(datatype e)
{//判满if(full()==1){expend();}//加入数据data[len] = e;len++;return 1;
}
bool Sqlist::insert_pos(int pos, datatype e)
{//加入数据if(add(e)==1){//把数据放入指定位置for(int i=len-1;i>=pos;i--){data[i+1] = data[i];}data[pos] = data[len];cout<<"数据添加成功"<<endl;return 1;}cout<<"添加失败"<<endl;return 0;
}
bool Sqlist::delete_pos(int pos)
{//判空if(empty()==1){cout<<"顺序表数组已空不能再删除了"<<endl;return 0;}//删除指定位置数据for(int i=pos+1;i<len;i++){data[i-1] = data[i];}len--;cout<<"数据删除成功"<<endl;return 1;
}void Sqlist::expend()
{//判满if(full()==0){return;}//初始化一个新堆区空间int new_size = 2*size;init(new_size);cout<<"扩容成功"<<endl;
}/*******************主程序*************************/
int main()
{Sqlist s1; //定义一个结构体变量s1.init(MAX); //初始化//插入数据while(1){int pos;datatype e;cout<<"请输入要放入的数据和位置"<<endl;cin>>e>>pos;//退出插入条件if(pos == -1){break;}s1.insert_pos(pos,e);}//删除数据while(1){int pos;cout<<"请输入要删除的数据位置"<<endl;cin>>pos;//退出删除条件if(pos == -1){break;}s1.delete_pos(pos);}//展示顺序表内容s1.output();return 0;
}
运行结果:
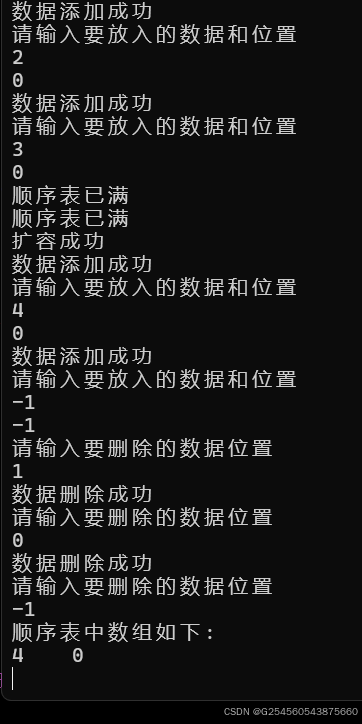
二、思维导图
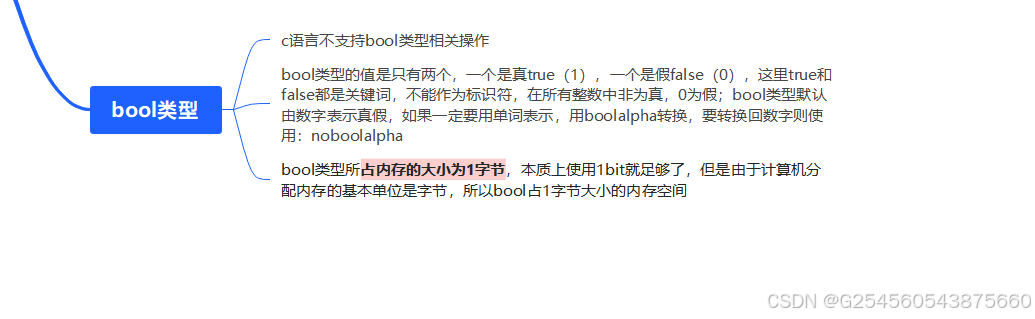
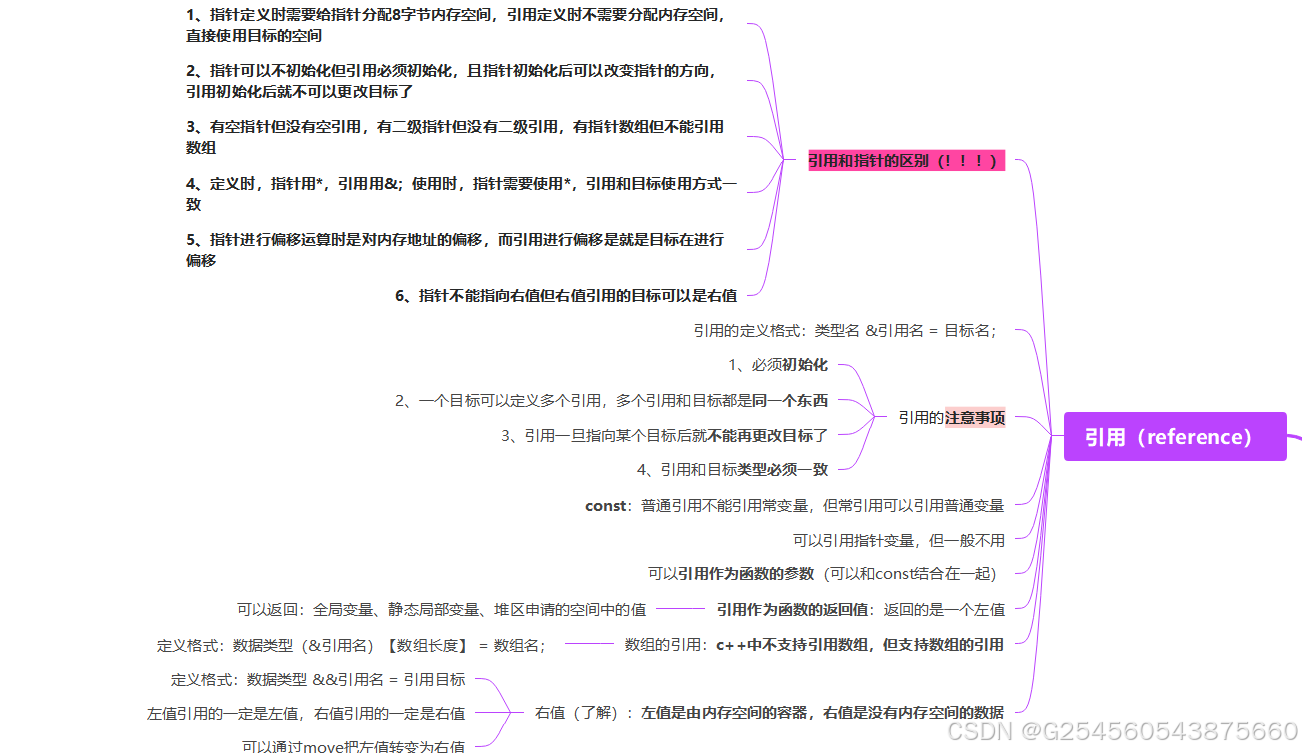
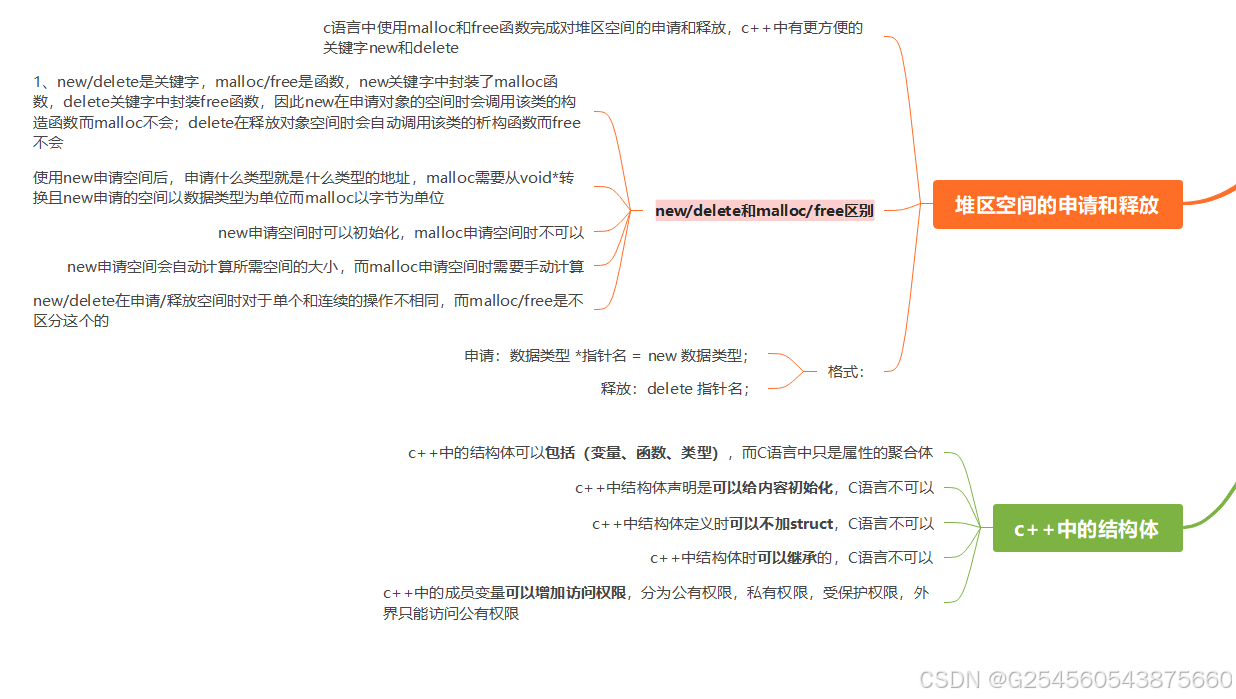