Knife4j(生成接口文档)
使用swagger你只需要按照它的规范去定义接口及接口相关的信息,就可以做到生成接口文档,以及在线接口调试页面。官网:https://swagger.io/
Knife4j是为Java MVC框架集成Swagger生成Api文档的增强解决方案。
使用方式
1、导入 knife4j的maven坐标
<dependency>
<groupld>com.github.xiaoymin</groupld>
<artifactld>knife4j-spring-boot-starter</artifactld>
<version>3.0.2</version>
</dependency>
2、在配置类中加入 knife4j相关配置
@Beanpublic Docket docket() {log.info("准备生成接口文档");ApiInfo apiInfo = new ApiInfoBuilder().title("xxx项目接口文档")//生成标题.version("2.0")//版本.description("xxx项目接口文档")//描述信息.build();Docket docket = new Docket(DocumentationType.SWAGGER_2).apiInfo(apiInfo)//生成接口文档的信息.select()//指定生成接口需要扫描的包.apis(RequestHandlerSelectors.basePackage("com.sky.controller")).paths(PathSelectors.any()).build();return docket;}
3、设置静态资源映射,否则接口文档页面无法访问
protected void addResourceHandlers(ResourceHandlerRegistry registry) {log.info("开始设置静态资源映");registry.addResourceHandler("/doc.html").addResourceLocations("classpath:/META-INF/resources/");registry.addResourceHandler("/webjars/**").addResourceLocations("classpath:/META-INF/resources/webjars/");}
通过localhost:8080/doc.html就可以访问了
静态资源未放在static资源包下
可以创建一个配置类
WebMvcConfig类:
package com.example.reggie.Config;import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport;@Slf4j
@Configuration
public class WebMvcConfig extends WebMvcConfigurationSupport {/*** Description:设置静态资源映射* date: 2024-07-16 21:50** @author: 努力敲代码的小火龙*/@Overrideprotected void addResourceHandlers(ResourceHandlerRegistry registry) {log.info("开始进行静态资源映射、、、");registry.addResourceHandler("/backend/**").addResourceLocations("classpath:/backend/");registry.addResourceHandler("/front/**").addResourceLocations("classpath:/front/");}
}
这样就可以完成静态资源映射
公共字段自动填充
这四个字段就属于公共字段,为表中插入数据时就要向这些公共字段赋值
代码冗余、不便于后期维护
那我们该如何实现公共字段自动填充呢?
1、自定义注解 AutoFil,用于标识需要进行公共字段自动填充的方法
枚举:
自定义注解:
package com.sky.annotation;import com.sky.enumeration.OperationType;import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;@Target(value = ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface AutoFill {//数据库操作类型OperationType value();
}
2、自定义切面类 AutoFilAspect,统一拦截加入了 AutoFi 注解的方法,通过反射为公共字段赋值
自定义切面类 AutoFilAspect:
package com.sky.Aspect;import com.sky.annotation.AutoFill;
import com.sky.constant.AutoFillConstant;
import com.sky.context.BaseContext;
import com.sky.enumeration.OperationType;
import lombok.extern.slf4j.Slf4j;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.Signature;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;import java.lang.reflect.Method;
import java.time.LocalDateTime;@Aspect
@Component
@Slf4j
public class AutoFillAspect {/*** Description: 切入点* date: 2024-07-18 13:38** @author: 努力敲代码的小火龙*/@Pointcut("execution(* com.sky.mapper.*.*(..)) && @annotation(com.sky.annotation.AutoFill)")public void autoFillPointCut() {};/*** Description:前置通知* date: 2024-07-18 13:42** @author: 努力敲代码的小火龙*/@Before("autoFillPointCut()")public void autoFill(JoinPoint joinPoint) throws NoSuchMethodException {log.info("开始进行公共字段自动填充...");//获取到当前被拦截的方法上的数据库操作类型MethodSignature signature = (MethodSignature) joinPoint.getSignature();//方法签名对象AutoFill annotation = signature.getMethod().getAnnotation(AutoFill.class);//获得方法上的注解对象OperationType value = annotation.value();//获得数库操作类型//获取到当前被拦截的方法的参数--实体对象Object[] args = joinPoint.getArgs();if (args == null || args.length == 0) {return;}Object entity = args[0];//准备赋值的数据LocalDateTime now = LocalDateTime.now();Long currentId = BaseContext.getCurrentId();//根据当前不同的操作类型,为对应的属性通过反射来赋值if (value == OperationType.INSERT) {try {Method setUpdateTime =entity.getClass().getDeclaredMethod(AutoFillConstant.SET_UPDATE_USER, LocalDateTime.class);Method setUpdateUser = entity.getClass().getDeclaredMethod(AutoFillConstant.SET_UPDATE_USER, Long.class);Method setCreateUser = entity.getClass().getDeclaredMethod(AutoFillConstant.SET_CREATE_USER, Long.class);Method setCreateTime = entity.getClass().getDeclaredMethod(AutoFillConstant.SET_CREATE_TIME, LocalDateTime.class);//通过反射为对象属性赋值setCreateTime.invoke(entity,now);setCreateUser.invoke(entity,currentId);setUpdateUser.invoke(entity,currentId);setUpdateTime.invoke(entity,now);} catch (Exception e) {e.printStackTrace();}} else if (value==OperationType.UPDATE) {try {Method setUpdateTime =entity.getClass().getDeclaredMethod(AutoFillConstant.SET_UPDATE_USER, LocalDateTime.class);Method setUpdateUser = entity.getClass().getDeclaredMethod(AutoFillConstant.SET_UPDATE_USER, Long.class);setUpdateUser.invoke(entity,currentId);setUpdateTime.invoke(entity,now);} catch (Exception e) {e.printStackTrace();}}}
}
3、在 Mapper 的方法上加入 AutoFil 注解
Spring Cache
Spring Cache 是一个框架,实现了基于注解的缓存功能,只需要简单地加一个注解,就能实现缓存功能。
Spring Cache 提供了一层抽象,底层可以切换不同的缓存实现,例如:EHCache、Caffeine、Redis
导入依赖
pom.xml
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-cache</artifactId></dependency>
常用注解:
@EnableCaching 开启缓存注解功能,通常加在启动类上
@Cacheable 在方法执行前先查询缓存中是否有数据,如果有数据则直接返回缓存数据;如果没有缓存数据,调用方法并将方法返回值放到缓存中
@CachePut 将方法的返回值放到缓存中
@CacheEvict 将一条或多条数据从缓存中删除
@EnableCaching 开启缓存注解功能,通常加在启动类上
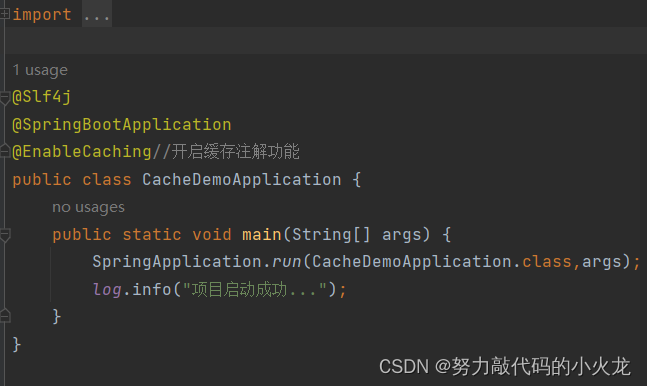
@Cacheable 在方法执行前先查询缓存中是否有数据,如果有数据则直接返回缓存数据;如果没有缓存数据,调用方法并将方法返回值放到缓存中
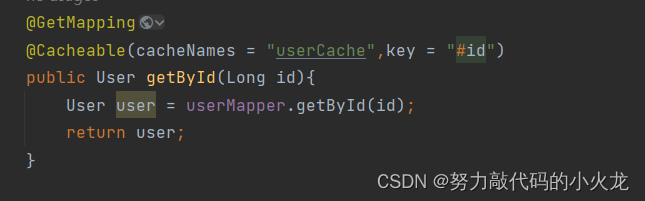
@CachePut 将方法的返回值放到缓存中
这个id是从下面的返回值取到的
这个是取第一个参数的id
这个也是取第一个参数的id
下面是上面的这个的语法
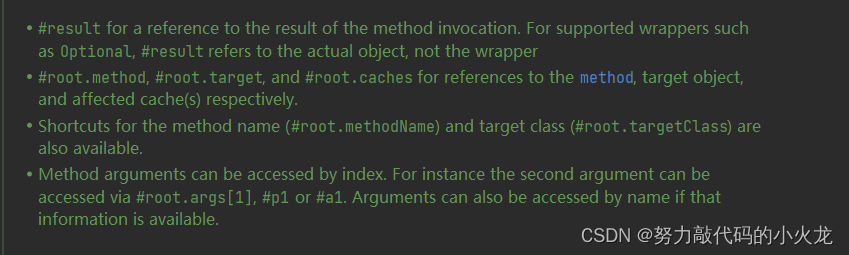
@CacheEvict 将一条或多条数据从缓存中删除
删除一条数据
删除多条数据
Spring Task
Spring Task 是Spring框架提供的任务调度工具,可以按照约定的时间自动执行某个代码逻辑。
定位:定时任务框架
作用:定时自动执行某段Java代码
cron表达式
cron表达式其实就是一个字符串,通过cron表达式可以定义任务触发的时间
构成规则:分为6或7个域,由空格分隔开,每个域代表一个含义
每个域的含义分别为:秒、分钟、小时、日、月、周、年(可选)
可以用编辑器直接生成cron表达式
Spring Task使用步骤
导入maven坐标spring-context
启动类添加注解 @EnableScheduling开启任务调度
自定义定时任务类