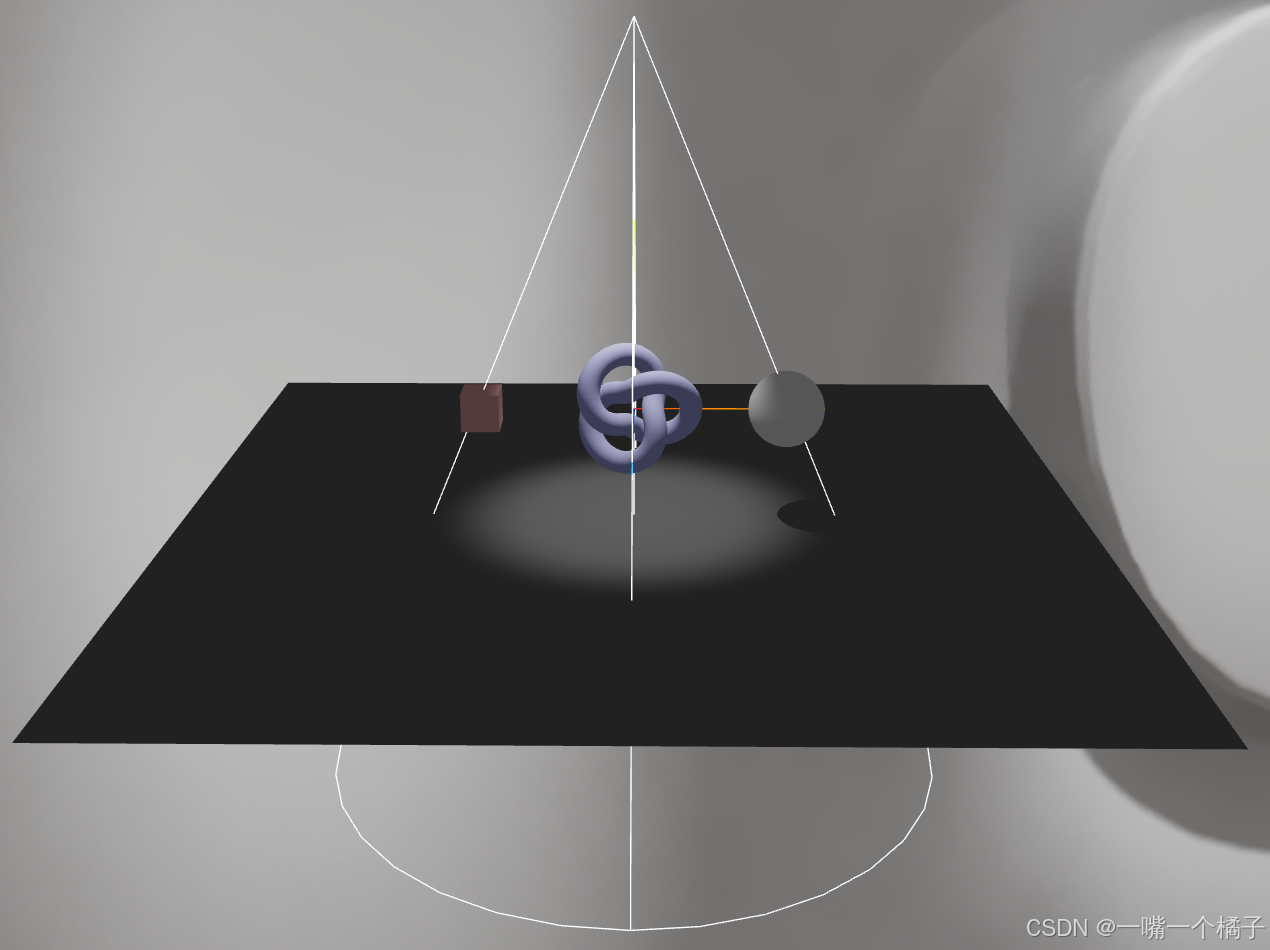
import * as THREE from 'three'
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls'
import { RGBELoader } from 'three/examples/jsm/loaders/RGBELoader.js'
import { GUI } from 'three/examples/jsm/libs/lil-gui.module.min.js'const scence = new THREE.Scene()const camera = new THREE.PerspectiveCamera(45, window.innerWidth / window.innerHeight, 0.1, 1000)
camera.position.set(15, 2.4, 0.4)
camera.lookAt(0, 0, 0)const renderer = new THREE.WebGLRenderer({antialias: true
})
renderer.setSize(window.innerWidth, window.innerHeight) renderer.shadowMap.enabled = true `【灯光与阴影 - 设置渲染器允许投射阴影】`renderer.outputColorSpace = THREE.SRGBColorSpace
renderer.toneMapping = THREE.ACESFilmicToneMapping
renderer.toneMappingExposure = 1
document.body.appendChild(renderer.domElement)
const axesHelper = new THREE.AxesHelper(5)
scence.add(axesHelper)const controls = new OrbitControls(camera, renderer.domElement)
controls.enableDamping = true
controls.dampingFactor = 0.05
controls.addEventListener('change', () => {renderer.render(scence, camera)
})
function render() {controls.update()renderer.render(scence, camera) requestAnimationFrame(render)
}
render()
const rgbeLoader = new RGBELoader()
rgbeLoader.load('../public/assets/texture/Video_Copilot-Back Light_0007_4k.hdr', envMap => {envMap.mapping = THREE.EquirectangularReflectionMappingscence.background = envMap
})'创建 - 环形结'const geometry = new THREE.TorusKnotGeometry(1, 0.3, 100, 16)const material = new THREE.MeshPhysicalMaterial({color: 0xccccff})const torusKnot = new THREE.Mesh(geometry, material)scence.add(torusKnot)'创建 - 球'const sphereGeometry = new THREE.SphereGeometry(1, 32, 32)const material_1 = new THREE.MeshPhysicalMaterial({color: 0xffffff})const sphere = new THREE.Mesh(sphereGeometry, material_1)sphere.position.set(4, 0, 0)scence.add(sphere)sphere.receiveShadow = truesphere.castShadow = true'创建 - 立方体'const boxGeometry = new THREE.BoxGeometry(1, 1, 1)const material_2 = new THREE.MeshPhysicalMaterial({color: 0xffcccc})const box = new THREE.Mesh(boxGeometry, material_2)box.position.set(-4, 0, 0)scence.add(box)'创建 - 平面'const planeGeometry = new THREE.PlaneGeometry(24, 24, 1, 1)const planeMaterial = new THREE.MeshPhysicalMaterial({color: 0x999999})const plane = new THREE.Mesh(planeGeometry, planeMaterial)plane.rotation.x = -Math.PI / 2plane.position.set(0, -3, 0)scence.add(plane)plane.receiveShadow = trueplane.castShadow = true'添加环境光'
let ambientLight = new THREE.AmbientLight(0xffffff, 0.1)
scence.add(ambientLight)`添加聚光灯`(手电筒那种锥形的光)const spotLight = new THREE.SpotLight(0xffffff, 2)spotLight.position.set(0, 10, 0) spotLight.target.position.set(0, 0, 0) spotLight.castShadow = true scence.add(spotLight)`锥形张开的角度`spotLight.angle = Math.PI / 8`光照射的距离(手电筒打光,不可能无限远不是)`spotLight.distance = 20`光照射,边缘部分的衰减`spotLight.penumbra = 0.5`光线强度的衰减程度,值越大,边缘部分就更加没光了`spotLight.decay = 2'添加聚光灯辅助器'let spotLightHelper = new THREE.SpotLightHelper(spotLight)scence.add(spotLightHelper)spotLight.shadow.mapSize.width = 2048spotLight.shadow.mapSize.height = 2048