1. File类
1.1 File的介绍
File是java.io.包下的类, File类的对象,用于代表当前操作系统的文件(可以是文件、或文件夹)。
注意:File类只能对文件本身进行操作,不能读写文件里面存储的数据。
1.2 File类的构造方法
构造方法 | 说明 |
---|
File(String pathName) | 通过给定的路径字符串构造对应的File对象 |
File(String parent,String child) | 通过给定的父路径字符串和子路径构造对应的File对象 |
File(File parent,String child) | 通过父路径的File文件和子路径构造对应的File对象 |
1.3 File类创建的方法
方法名 | 说明 |
---|
createNewFile() | 如果当前File对象描述的文件不存在则创建此文件并且返回true。如果文件已经存在返回flase。如果创建的文件的路径中有不存在的目录则创建失败并且出现异常 |
mkdir() | 如果当前File对象描述的目录不存在则创建目录并且返回true。如果目录存返回flase。如果创建的目录的父目录不存在则创建失败。不能创建多级目录 |
mkdirs() | 如果当前File对象描述的目录不存在则创建目录并且返回true。可以创建多级目录 |
public class Demo1 {public static void main(String[] args) throws IOException {File file1 = new File("E://a.txt");boolean b = file1.createNewFile();System.out.println(b);}
}
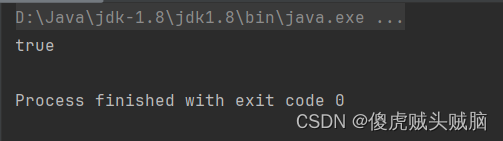
public static void main(String[] args) {//需求:键盘输入一个路径,创建此路径的所有内容//E://aa/bb/c.txtFile file = new File("E://aa/bb/c.txt");boolean newFile = file.mkdirs();System.out.println(newFile);}
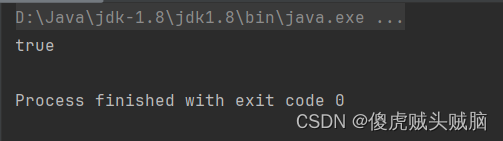
1.4 File删除方法
public static void main(String[] args) throws IOException {File file1 = new File("E://a.txt");boolean b = file1.delete();System.out.println(b);}
1.5 File判断方法
方法名 | 描述 |
---|
exists() | 判断是否存在 |
isDirectory() | 判断是否是文件夹 |
isFile() | 判断是否是文件 |
1.6 File的获取方法
方法名 | 描述 |
---|
getAbsolutePath() | 获取文件的绝对路径 |
getName() | 获取文件名 |
listFiles() | 获取当前路径下所有的子目录和子文件的File数组(只能获取当前目录下) |
2. IO流
IO:输入/输出(Input/Output)
流:流是一种抽象的概念,是对数据传输的一种总称。数据在设备之间传输就称之为流
2.1 IO流分类
2.2 字节输出流
字节流的超类:
InputStream:输入
OutputStream:输出
但是两个类是抽象类,直接实例化使用不方便。
方法名 | 描述 |
---|
write(int b) | 将数字对应的字符写入到文件输出流。一次写入一个字节的数据 |
write(byte[] b) | 将b.length长度的字节从指定字节数组中写入到文件输出流。 一次写一个数组的数组 |
write(byte[] b,int off ,int len) | 将len长度的字节从指定数组(b)开始,从偏移量off开始写入到文件输出流。一次写入len个长度字节 |
close() | 关闭流 |
2.3 字节输入流
方法名 | 描述 |
---|
read() | 每次读取一个字节 |
read(byte[] b) | 每次读取一个数组,并且读取的长度 |
read(byte[] b,int off ,int len) | 每次读取一个数组 |
public static void main(String[] args) throws IOException {FileInputStream fis = new FileInputStream("E://a.txt");int len;while((len=fis.read())!=-1){System.out.println((char)len);}fis.close();
}
2.4 案例
public static void main(String[] args) {//需求: 完成文件复制//E://a/a.txt//E://b/a.txtFile file1 = new File("E://a/a.txt");File file2 = new File("E://b/a.txt");//每次读的容量byte[] roby = new byte[1024];int len;try {//输入流读FileInputStream fileInput = new FileInputStream(file1);//输出流写FileOutputStream fileOutput = new FileOutputStream(file2);while ((len = fileInput.read(roby)) != 1) {fileOutput.write(roby, 0, len);}fileInput.close();fileOutput.close();} catch (FileNotFoundException e) {throw new RuntimeException(e);} catch (IOException e) {throw new RuntimeException(e);}}
2.5 字节缓冲流
BufferedInputStream:输入字节缓冲流
BufferedOutputStream:输出字节缓冲流
相比较字节流来说,存在了一个缓冲池可以提高效率
public static void main(String[] args) throws IOException {BufferedInputStream buffInput = new BufferedInputStream(new FileInputStream("D://a.txt"));BufferedOutputStream buffOutput = new BufferedOutputStream(new FileOutputStream("D://b.txt"));byte[] roBy = new byte[1024];int len;while((len=buffInput.read(roBy))!=-1){buffOutput.write(roBy,0,len);}buffInput.close();buffOutput.close();}
3. 字符流
3.1 字符流的超类
- Writer:所有输出字符流的超类
- Reader:所有输入字符流的超类
3.2 FileReader(文件字符输入流)
- 作用:以内存为基准,可以把文件中的数据以字符的形式读入到内存中去。
构造方法 | 描述 |
---|
FileReader(String filePath) | 根据指定文件路径实例化FileReader对象 |
FileReader(File file) | 根据指定文件对象实例化FileReader对象 |
方法名 | 描述 |
---|
reader() | 一次读取一个字符,如果发现没有数据可读会返回-1. |
reader(char [] ch) | 一次读取一个字符数组 |
reader(char [] ch,int off,int len) | 一次读取一个字符数组一部分 |
3.3 FileWriter(文件字符输出流)
- 作用:以内存为基准,把内存中的数据以字符的形式写出到文件中去。
构造方法 | 描述 |
---|
FileWriter(String FileName) | 根据指定文件路径实例化FileWriter对象 |
FileWriter(String FileName,Boolean append) | 同上可追加数据 |
FileWriter(File file) | 根据指定文件实例化FileWriter对象 |
FileWriter(File file,Boolean append) | 同上可追加数据 |
方法名 | 描述 |
---|
write(int c) | 写入一个字符 |
write(char [] ch) | 写入一个字符数组 |
write(char [] ch ,int off ,int len) | 写入一个字符数组一部分 |
write(String str) | 写入一个字符串 |
write(String str,int off ,int len) | 写入一个字符串一部分 |