前序线索化
#include<iostream>
using namespace std;typedef int datatype;
typedef struct BitNode
{datatype Data;struct BitNode* leftchild;struct BitNode* rightchild;int lefttag;int righttag;
}Node;
#pragma region 前序线索化递归遍历
Node* previous = NULL;
void PreCuleOrder(Node* root)
{if (root == NULL){return;}Node* tmpnode = NULL;tmpnode = root;if (tmpnode->leftchild == NULL ){tmpnode->leftchild = previous;tmpnode->lefttag = 1;}if (previous != NULL && previous->rightchild == NULL){previous->rightchild = tmpnode;previous->righttag = 1;}previous = tmpnode;if (tmpnode->lefttag ==0){PreCuleOrder(tmpnode->leftchild);}if (tmpnode->righttag == 0){PreCuleOrder(tmpnode->rightchild);}
}
#pragma endregion
前序线索化遍历
#pragma region 前序遍历线索化二叉树
void PreIterativeTree(Node* root)
{if (root == NULL){return;}Node* tmpnode = NULL;tmpnode = root;while (tmpnode!=NULL){/*如果左子树一直非存在前继结点 那么一直遍历*/while (tmpnode->lefttag ==0){ cout << tmpnode->Data << endl;tmpnode = tmpnode->leftchild;}/*出现连接上一层前继结点的叶子结点*/while (tmpnode->rightchild !=NULL){if (tmpnode->lefttag ==0){break;}else{cout << tmpnode->Data << endl;tmpnode = tmpnode->rightchild;}}if (tmpnode->rightchild == NULL){cout << tmpnode->Data << endl;break;}}
}
#pragma endregion
主函数代码
int main(void)
{BitNode* n1 = new BitNode();BitNode* n2 = new BitNode();BitNode* n3 = new BitNode();BitNode* n4 = new BitNode();BitNode* n5 = new BitNode();BitNode* n6 = new BitNode();BitNode* n7 = new BitNode();BitNode* n8 = new BitNode();BitNode* n9 = new BitNode();n1->Data = 1, n2->Data = 2, n3->Data = 3, n4->Data = 4, n5->Data = 5, n6->Data = 6, n7->Data = 7, n8->Data = 8, n9->Data = 9;n1->leftchild = n2;n1->lefttag = 0;n2->leftchild = n4;n2->lefttag = 0;n4->leftchild = n5;n4->lefttag = 0;n1->rightchild = n3;n1->righttag = 0;n3->rightchild = n7;n3->righttag = 0;n3->leftchild = n6;n3->lefttag = 0;n7->leftchild = n9;n7->lefttag = 0;n7->rightchild = n8;n7->righttag = 0;PreCuleOrder(n1);PreIterativeTree(n1);return 0;
}
最终运行结果
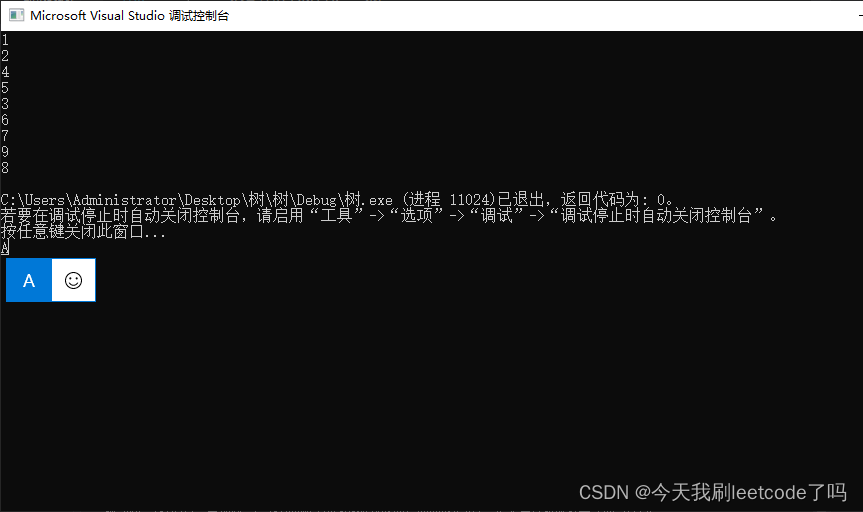