目录
一、二叉搜索树
二、AVL树
2.1 左单旋
2.2 右单旋
2.3 左右双旋
2.4 右左双旋
三、AVL.h
四、test.cpp
一、二叉搜索树
二叉搜索树,又称二叉排序树(Binary Search Tree),相比于普通二叉树,BST的特性有:
- 若它的左子树不为空,则左子树上所有节点的值都小于根节点的值
- 若它的右子树不为空,则右子树上所有节点的值都大于根节点的值
- 它的左右子树也分别为二叉搜索树
二叉搜索树通常在数据插入的时候便会将数据正确排序(不允许插入重复值),这样在进行数据查询的时候,一般情况下时间复杂度是,但是如果插入的数据为一个有序或接进有序的序列,即退化成了单支树,这时时间复杂度退化到
。
二、AVL树
AVL树也叫平衡二叉搜索树,是二叉搜索树的进化版,设计是原理是弥补二叉搜索树的缺陷:当插入的数据接近于有序数列时,二叉搜索树的性能严重下降。
AVL的节点设计采用三叉链结构(每个节点包含left, right, parent三个节点指针),每个节点中都有平衡因子bf。
AVL树在BST的基础上引入了一个平衡因子bf用于表示左右子树的高度差,并控制其不超过1,若在数据插入时左右子树的高度差超过1了,AVL树会寻找新的节点作为根节点进行调整树的结构,这样AVL树进行数据查询的时间复杂度就稳定在了。
AVL的特点是左子树和右子树高度差 < 2,平衡因子bf就是右子树高度 - 左子树高度的差,当bf等于2或-2时,AVL将根据不同情况进行旋转调节,使其始终保持AVL树的特性。
对于一棵AVL树,若它的节点数为n,则它的深度为。
2.1 左单旋
若数列 1,2,3 按顺序插入AVL树,则树的结构为图1:
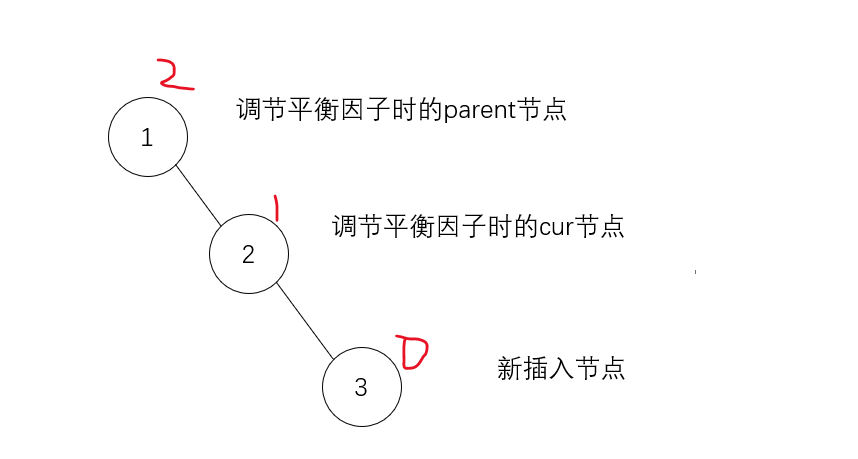
图1 中,parent节点的平衡因子bf为2,新节点向上调节时的cur节点的平衡因子为1,这种情况需要左单旋:cur节点的左子树(空)变成parent节点的右子树,parent节点变成cur节点的左子树。
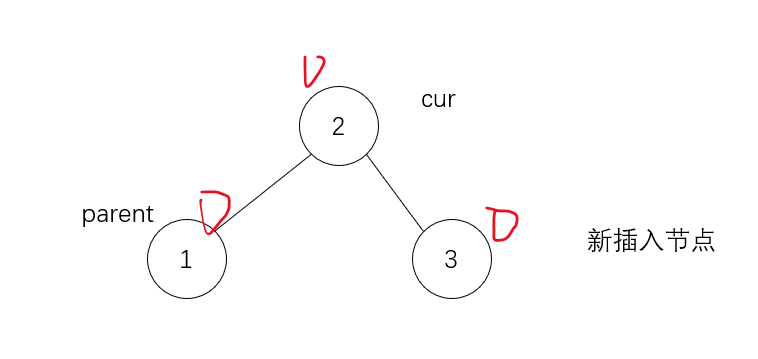
2.2 右单旋
右单旋与左单旋类似,也是根据平衡因子情况进行旋转调整。
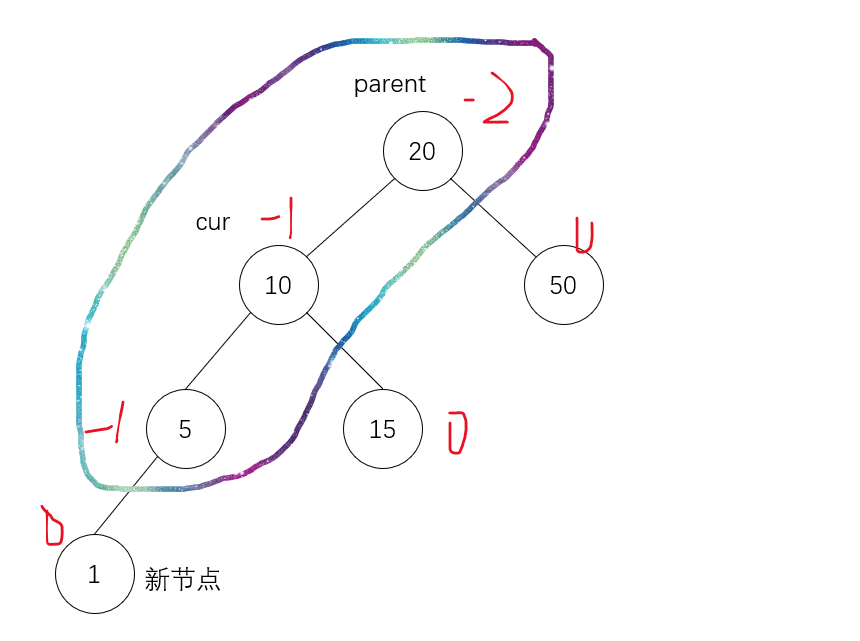
图1 新节点1插入,向上调整平衡因子,出现parent->bf == -2, cur->bf == -1,此时需要右单旋:cur节点的右子树变为parent节点的左子树,parent变成cur节点的右子树。
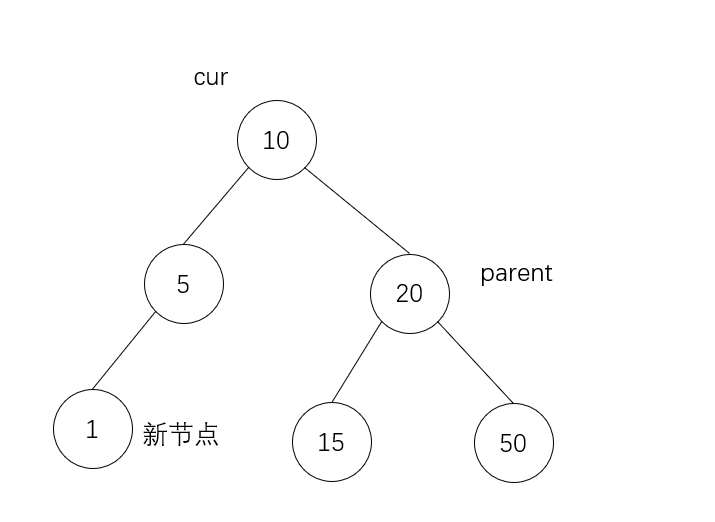
2.3 左右双旋
左右双旋在设计上可以调用左单旋和右单旋函数,但是需要不同情况讨论旋转后的平衡因子。
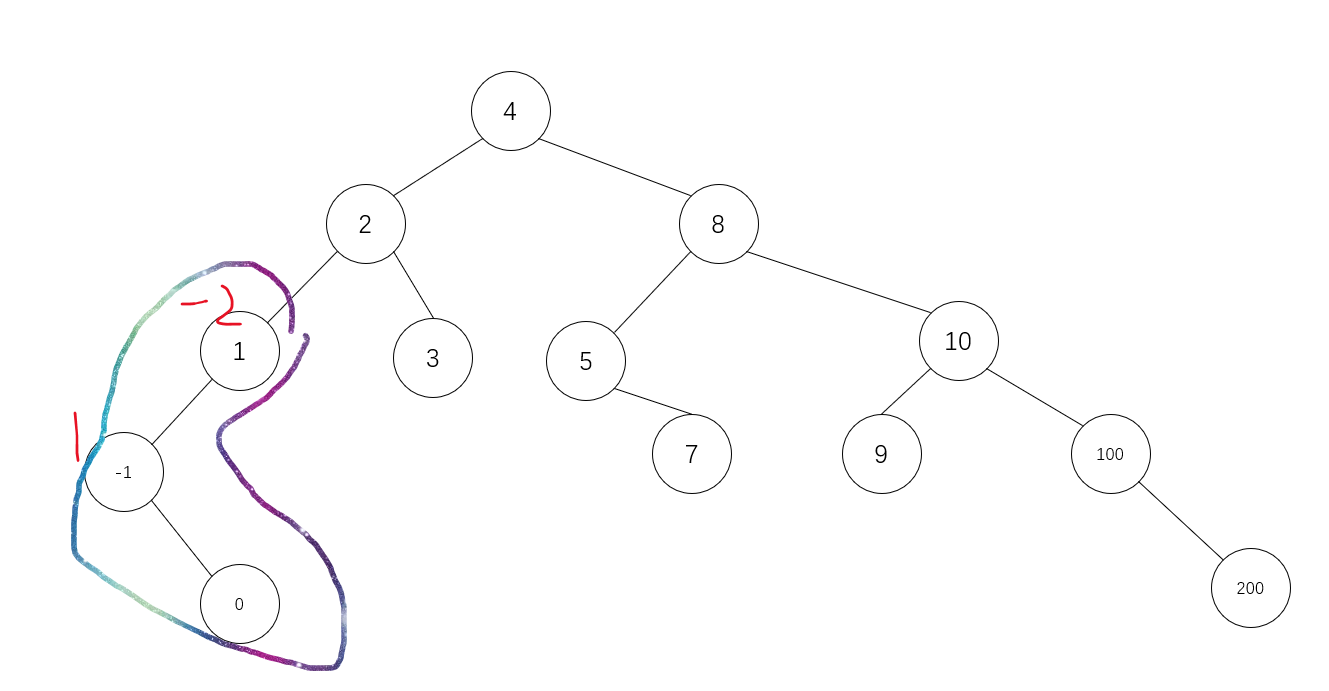
图1 新插入节点0后,parent->bf == -2, cur->bf == 1,此时需要左右双旋,即先以节点-1为父节点进行一次左单旋,再以1为父节点进行一次左单旋:节点0的左子树(空)变成节点-1的左子树,节点-1变成节点0的左子树。
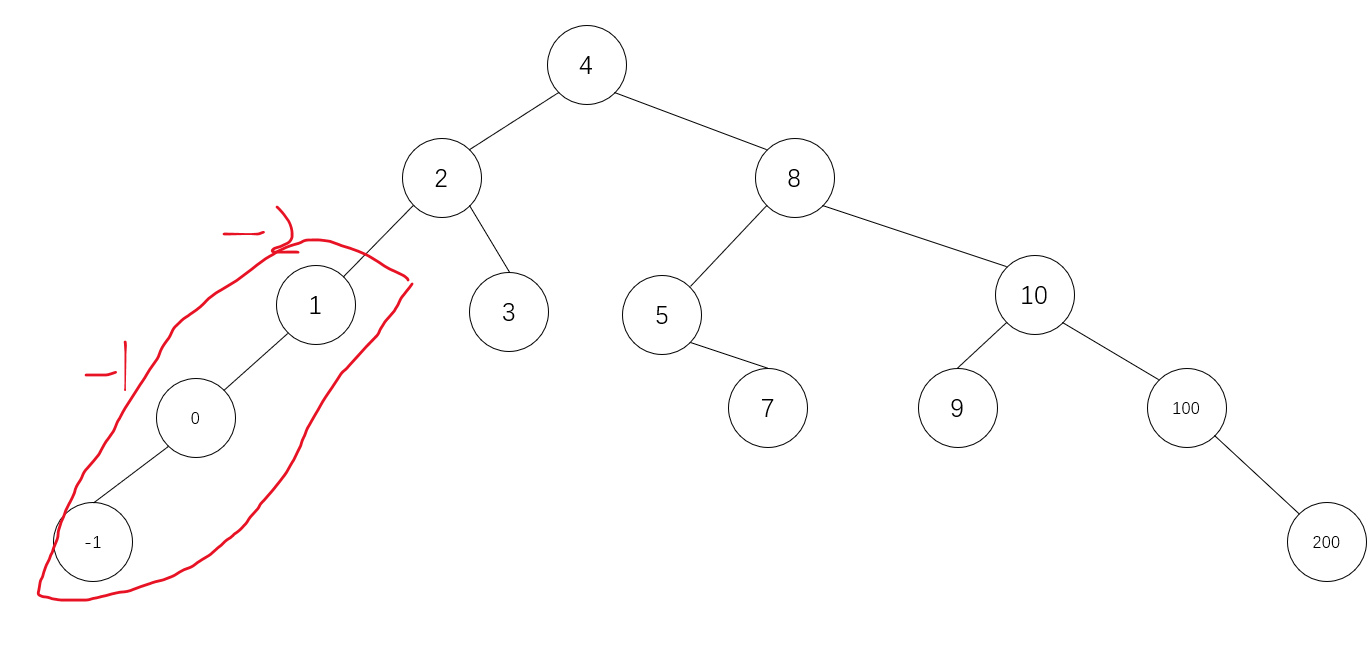
图2 完成左单旋之后再以1位父节点进行一次右单旋:节点0的右子树(空)变成节点1的左子树,节点1变成节点0的右子树。
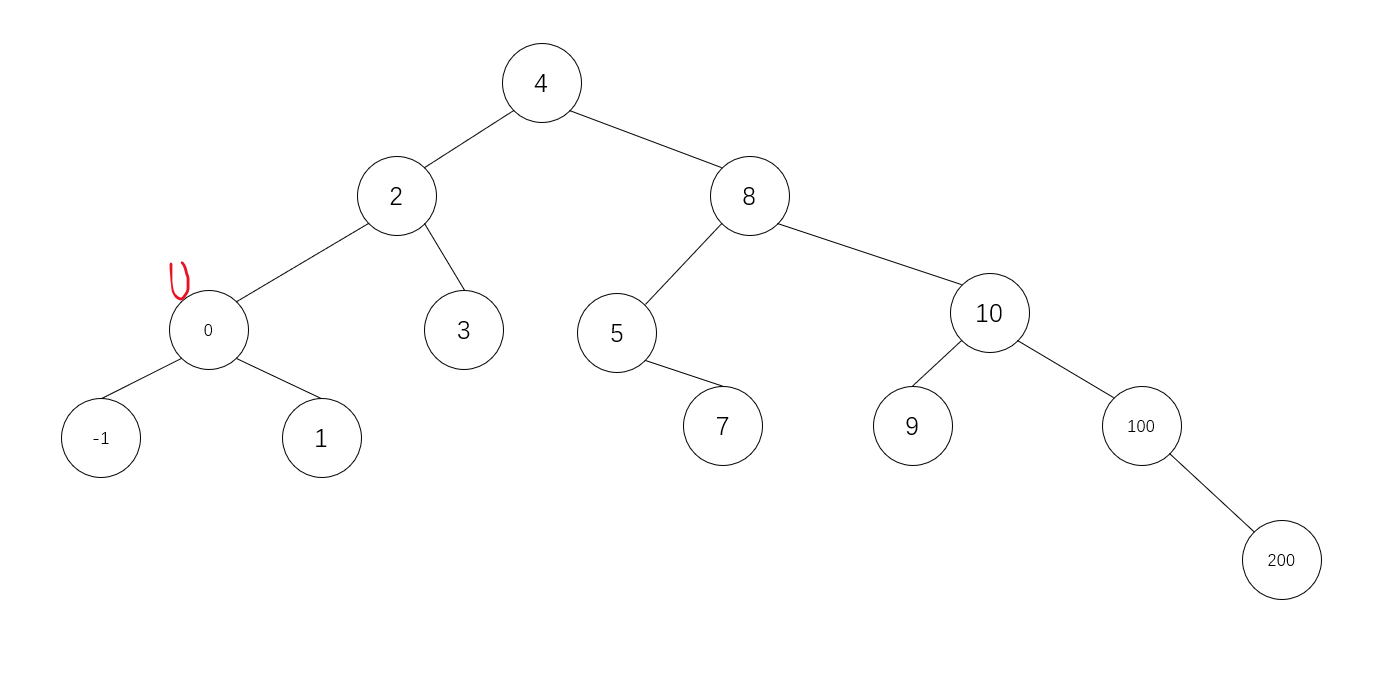
2.4 右左双旋
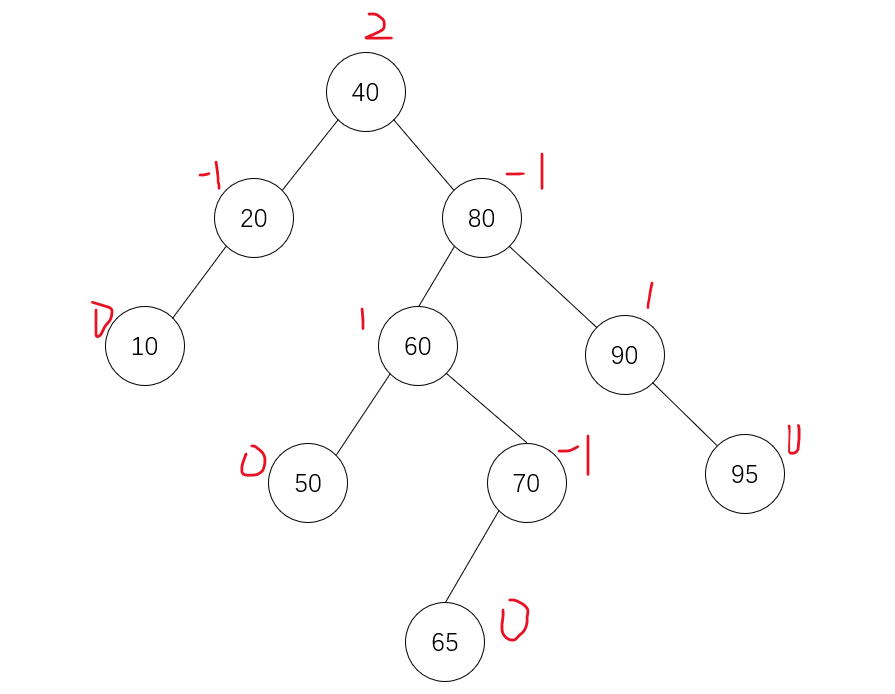
图1 中红色数字就是每个节点平衡因子,值为65的节点是新插入的节点,当其插入之后,所有节点的平衡因子更新,出现了parent平衡因子为2,cur平衡因子为1,此时需要进行旋转调节。
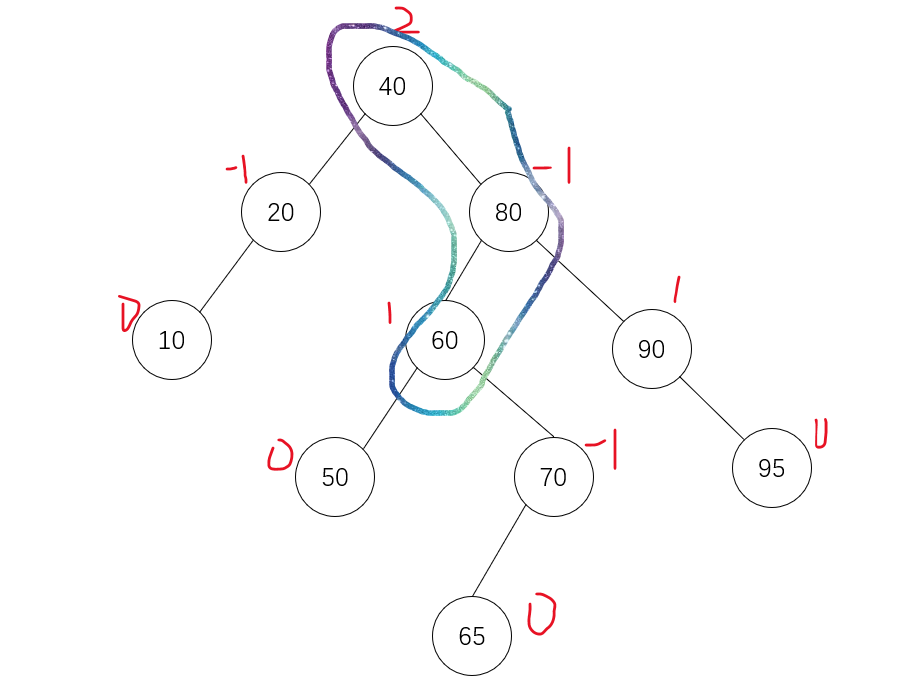
图2 观察平衡因子,决定旋转策略为右左双旋,即先进行一次右单旋,再进行一次左单旋。右单旋是以节点80为父节点进行,即节点60的右子树变成节点80的左子树,节点80变成节点60的右子树。
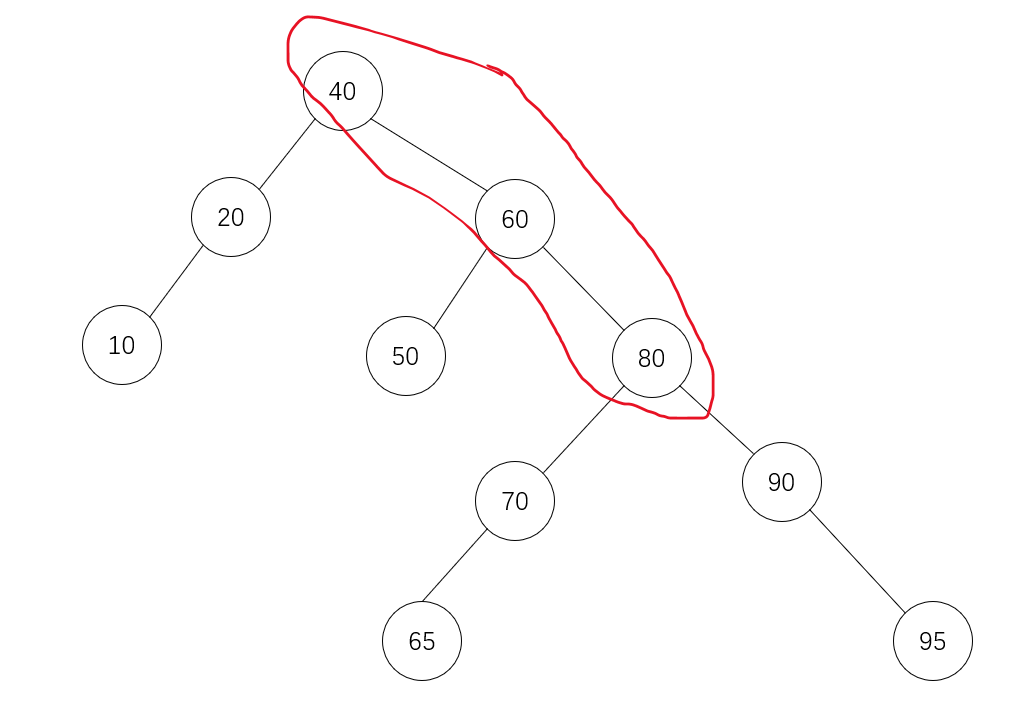
图3 右单旋结束,接下来再一次进行以节点40为父节点的左单旋,即节点60的左子树变成节点40的右子树,节点40变成节点60的左子树。
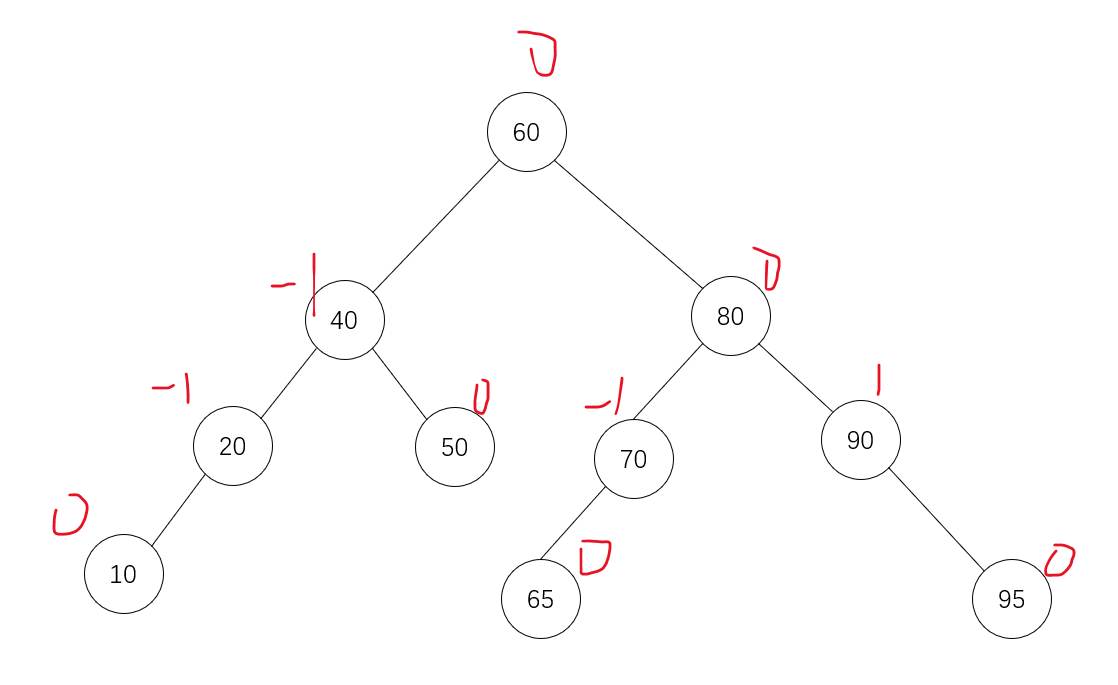
三、AVL.h
#define _CRT_SECURE_NO_WARNINGS 1#pragma once
#include <iostream>template<class K, class V>
struct AVLTreeNode
{std::pair<K, V> kv;AVLTreeNode* parent;AVLTreeNode* left;AVLTreeNode* right;int bf;AVLTreeNode(const std::pair<K, V> x): kv(x), parent(nullptr), left(nullptr), right(nullptr), bf(0){}
};template<class K, class V>
class AVLTree
{typedef AVLTreeNode<K, V> Node;
public:bool Insert(const std::pair<K, V>& x){if (_root == nullptr){_root = new Node(x);return true;}// 通过二叉搜索树找到需要插入节点的位置Node* parent = nullptr;Node* cur = _root;while (cur){parent = cur;if (cur->kv.first < x.first)cur = cur->right;else if (cur->kv.first > x.first)cur = cur->left;elsereturn false;}cur = new Node(x);cur->parent = parent;if (parent->kv.first > x.first)parent->left = cur;elseparent->right = cur;// 更新平衡因子while (parent){if (parent->left == cur)--parent->bf;else++parent->bf;if (parent->bf == 0){return true;}else if (parent->bf == 1 || parent->bf == -1){// 继续向上更新cur = parent;parent = parent->parent;}else if (parent->bf == 2 || parent->bf == -2){if (parent->bf == 2 && cur->bf == 1)_RotateLeft(parent);else if (parent->bf == -2 && cur->bf == -1)_RotateRight(parent);else if (parent->bf == -2 && cur->bf == 1)_RotateLeftRight(parent);else if (parent->bf == 2 && cur->bf == -1)_RotateRightLeft(parent);break;}}}void InOrder(){_InOrder(_root);std::cout << std::endl;}bool IsBalance(){return _IsBalance(_root);}private:void _RotateLeft(Node* parent){Node* subR = parent->right;Node* subRL = subR->left;parent->right = subRL;if (subRL != nullptr)subRL->parent = parent;subR->left = parent;Node* ppNode = parent->parent;parent->parent = subR;if (ppNode == nullptr){_root = subR;_root->parent = nullptr;}else{if (ppNode->left == parent)ppNode->left = subR;elseppNode->right = subR;subR->parent = ppNode;}parent->bf = subR->bf = 0;}void _RotateRight(Node* parent){Node* subL = parent->left;Node* subLR = subL->right;parent->left = subLR;if (subLR != nullptr)subLR->parent = parent;subL->right = parent;Node* ppNode = parent->parent;parent->parent = subL;if (ppNode == nullptr){_root = subL;_root->parent = nullptr;}else{if (ppNode->left == parent)ppNode->left = subL;elseppNode->right = subL;subL->parent = ppNode;}parent->bf = subL->bf = 0;}void _RotateLeftRight(Node* parent){Node* subL = parent->left;Node* subLR = subL->right;int bf = subLR->bf;_RotateLeft(subL);_RotateRight(parent);if (bf == 0){subLR->bf = 0;subL->bf = 0;parent->bf = 0;}else if (bf == -1){subLR->bf = 0;subL->bf = 0;parent->bf = 1;}else if (bf == 1){subLR->bf = 0;subL->bf = -1;parent->bf = 0;}}void _RotateRightLeft(Node* parent){Node* subR = parent->right;Node* subRL = subR->left;int bf = subRL->bf;_RotateRight(subR);_RotateLeft(parent);if (bf == 0){subRL->bf = 0;subR->bf = 0;parent->bf = 0;}else if (bf == -1){subRL->bf = 0;subR->bf = 1;parent->bf = 0;}else if (bf == 1){subRL->bf = 0;subR->bf = 0;parent->bf = -1;}}void _InOrder(Node* root){if (root == nullptr)return;_InOrder(root->left);std::cout << "<" << root->kv.first << "," << root->kv.second << ">" << " ";_InOrder(root->right);}int _Height(Node* root){if (root == nullptr)return 0;int leftHeight = _Height(root->left);int rightHeight = _Height(root->right);return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;}bool _IsBalance(Node* root){if (root == nullptr)return true;int leftHeight = _Height(root->left);int rightHeight = _Height(root->right);if (rightHeight - leftHeight != root->bf){std::cout << "平衡因子异常" << std::endl;return false;}return abs(rightHeight - leftHeight) < 2&& _IsBalance(root->left)&& _IsBalance(root->right);}private:Node* _root = nullptr;
};
四、test.cpp
#define _CRT_SECURE_NO_WARNINGS 1#include "AVL.h"void Test1()
{int a[] = { 2, 4, 5, 8, 10, 1, 3, 5, 7, 9, 100, 200, -100, 0 };AVLTree<int, int> t;for (auto e : a){t.Insert(std::make_pair(e, e * 2));}t.InOrder();std::cout << t.IsBalance() << std::endl << std::endl;
}void Test2()
{int a[] = { 16, 3, 7, 11, 9, 26, 18, 14, 15 };AVLTree<int, int> t;for (auto e : a){t.Insert(std::make_pair(e, e * 2));}t.InOrder();std::cout << t.IsBalance() << std::endl << std::endl;
}void Test3()
{int a[] = { 4, 2, 6, 1, 3, 5, 15, 7, 16, 14 };AVLTree<int, int> t;for (auto e : a){t.Insert(std::make_pair(e, e * 2));}t.InOrder();std::cout << t.IsBalance() << std::endl << std::endl;
}int main()
{Test1();Test2();Test3();return 0;
}