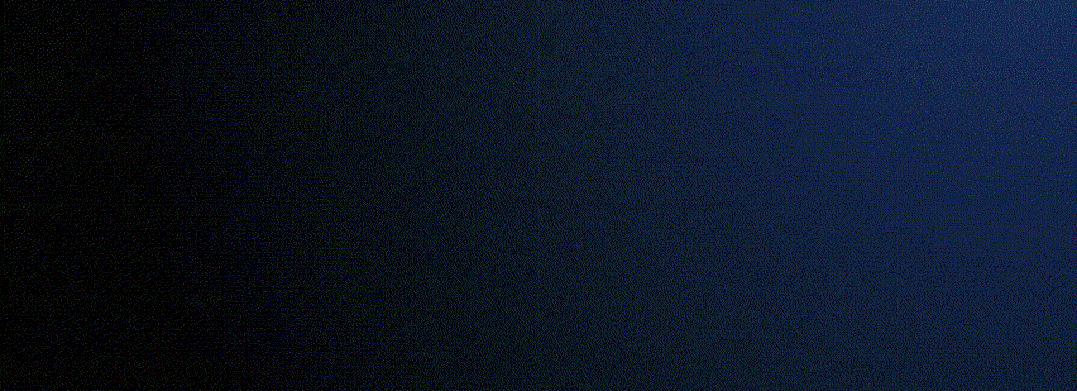
实战指南:如何在Spring Boot中无缝整合Dubbo【四】
- 前言
- 项目结构
- 主项目(作为主pom)
- 接口
- 服务提供者
- properties文件
- 实现类
- 服务消费者
- properties
- 接口层
- 实现效果图
前言
微服务架构已经成为现代应用开发的热门趋势,而Dubbo作为一款强大的分布式服务框架,与Spring Boot的结合是构建高性能微服务应用的理想选择。就像拼装一把锋利的刀刃,让我们一起揭开Spring Boot整合Dubbo的神秘面纱,探索如何在分布式世界中获得竞争优势。
项目结构
主项目(作为主pom)
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>fun.bo</groupId><artifactId>dubbo-studys</artifactId><version>1.0-SNAPSHOT</version><packaging>pom</packaging><modules><module>dubbo-provider</module><module>dubbo-interface</module><module>dubbo-consumer</module></modules><properties><java.version>1.8</java.version><maven.compiler.source>8</maven.compiler.source><maven.compiler.target>8</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding><dubbo-version>2.7.8</dubbo-version><spring-boot.version>2.3.0.RELEASE</spring-boot.version></properties><dependencyManagement><dependencies><!-- Spring Boot --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-dependencies</artifactId><version>${spring-boot.version}</version><type>pom</type><scope>import</scope></dependency><!-- Apache Dubbo --><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo-dependencies-bom</artifactId><version>${dubbo-version}</version><type>pom</type><scope>import</scope></dependency><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo-spring-boot-starter</artifactId><version>${dubbo-version}</version></dependency><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo</artifactId><version>${dubbo-version}</version><exclusions><exclusion><groupId>org.springframework</groupId><artifactId>spring</artifactId></exclusion><exclusion><groupId>javax.servlet</groupId><artifactId>servlet-api</artifactId></exclusion><exclusion><groupId>log4j</groupId><artifactId>log4j</artifactId></exclusion></exclusions></dependency></dependencies></dependencyManagement><dependencies><!-- Dubbo Spring Boot Starter --><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo-spring-boot-starter</artifactId><version>${dubbo-version}</version></dependency><!-- Dubbo核心组件 --><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo</artifactId></dependency><!--Spring Boot 依赖 --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId><version>${spring-boot.version}</version></dependency><!-- Zookeeper客户端框架 --><dependency><groupId>org.apache.curator</groupId><artifactId>curator-framework</artifactId><version>4.0.1</version></dependency><!-- Zookeeper dependencies --><dependency><groupId>org.apache.dubbo</groupId><artifactId>dubbo-dependencies-zookeeper</artifactId><version>${dubbo-version}</version><type>pom</type><exclusions><exclusion><groupId>org.slf4j</groupId><artifactId>slf4j-log4j12</artifactId></exclusion></exclusions></dependency></dependencies></project>
接口
package fun.bo.api;/*** @author todoitbo* @date 2024/1/12*/
public interface TestDubboService {String sayHello(String name);
}
服务提供者
properties文件
# 服务端口
server.port=18081
# 应用程序名称
spring.application.name=dubbo-provider
# Dubbo服务扫描路径
dubbo.scan.base-packages=fun.bo# Dubbo 通讯协议
dubbo.protocol.name=dubbo
# Dubbo服务提供的端口, 配置为-1,代表为随机端口 默认20880
dubbo.protocol.port=-1## Dubbo 注册器配置信息
dubbo.registry.address=zookeeper://127.0.0.1:2181
dubbo.registry.file = ${user.home}/dubbo-cache/${spring.application.name}/dubbo.cache
dubbo.spring.provider.version = 1.0.0
实现类
package fun.bo.iml;import fun.bo.api.TestDubboService;
import org.apache.dubbo.config.annotation.DubboService;
import org.springframework.beans.factory.annotation.Value;/*** @author todoitbo* @date 2024/1/12*/
@DubboService(version = "${dubbo.spring.provider.version}")
public class TestDubboServiceImpl implements TestDubboService {@Overridepublic String sayHello(String name) {return "hello " + name;}
}
服务消费者
properties
# 服务端口
server.port=18084
#服务名称
spring.application.name=dubbo-spring-consumer
#服务版本号
dubbo.spring.provider.version = 1.0.0
#消费端注册器配置信息
dubbo.registry.address=zookeeper://127.0.0.1:2181
#dubbo.consumer.check=false
dubbo.registry.file = ${user.home}/dubbo-cache/${spring.application.name}/dubbo.cache
接口层
package fun.bo.controller;import fun.bo.api.TestDubboService;
import org.apache.dubbo.config.annotation.DubboReference;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;/*** @author todoitbo* @date 2024/1/12*/
@RestController
public class TestDubboController {@DubboReference(version = "${dubbo.spring.provider.version}")private TestDubboService testDubboService;@GetMapping("/test-dubbo/{name}")public String testDubbo(@PathVariable String name) {return testDubboService.sayHello(name);}
}