目录
视频演示
代码实现
视频演示
Chrome小恐龙快跑小游戏——Python实现
代码实现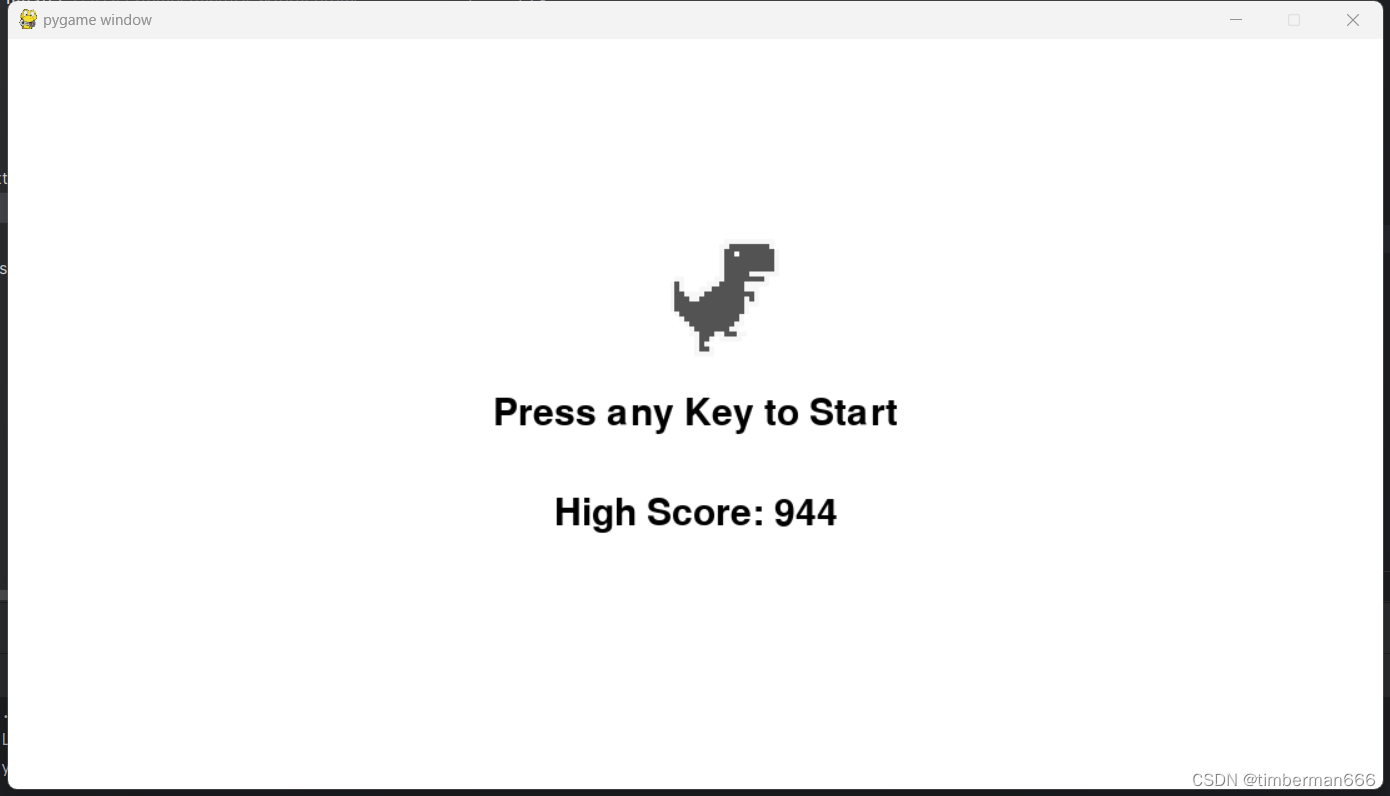
import pygame
import os
import random
pygame.init()# Global Constants
SCREEN_HEIGHT = 600
SCREEN_WIDTH = 1100
game_over = False
SCREEN = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))RUNNING = [pygame.image.load(os.path.join("Dino", "DinoRun1.png")),pygame.image.load(os.path.join("Dino", "DinoRun2.png"))]
JUMPING = pygame.image.load(os.path.join("Dino", "DinoJump.png"))
DUCKING = [pygame.image.load(os.path.join("Dino", "DinoDuck1.png")),pygame.image.load(os.path.join("Dino", "DinoDuck2.png"))]SMALL_CACTUS = [pygame.image.load(os.path.join("Cactus", "SmallCactus1.png")),pygame.image.load(os.path.join("Cactus", "SmallCactus2.png")),pygame.image.load(os.path.join("Cactus", "SmallCactus3.png"))]
LARGE_CACTUS = [pygame.image.load(os.path.join("Cactus", "LargeCactus1.png")),pygame.image.load(os.path.join("Cactus", "LargeCactus2.png")),pygame.image.load(os.path.join("Cactus", "LargeCactus3.png"))]BIRD = [pygame.image.load(os.path.join("Bird", "Bird1.png")),pygame.image.load(os.path.join("Bird", "Bird2.png"))]CLOUD = pygame.image.load(os.path.join("Other", "Cloud.png"))BG = pygame.image.load(os.path.join("Other", "Track.png"))class Dinosaur:X_POS = 80Y_POS = 310Y_POS_DUCK = 340JUMP_VEL = 8.5def __init__(self):self.duck_img = DUCKINGself.run_img = RUNNINGself.jump_img = JUMPINGself.dino_duck = Falseself.dino_run = Trueself.dino_jump = Falseself.step_index = 0self.jump_vel = self.JUMP_VELself.image = self.run_img[0]self.dino_rect = self.image.get_rect()self.dino_rect.x = self.X_POSself.dino_rect.y = self.Y_POSdef update(self, userInput):if self.dino_duck:self.duck()if self.dino_run:self.run()if self.dino_jump:self.jump()if self.step_index >= 10:self.step_index = 0if userInput[pygame.K_UP] and not self.dino_jump:self.dino_duck = Falseself.dino_run = Falseself.dino_jump = Trueelif userInput[pygame.K_DOWN] and not self.dino_jump:self.dino_duck = Trueself.dino_run = Falseself.dino_jump = Falseelif not (self.dino_jump or userInput[pygame.K_DOWN]):self.dino_duck = Falseself.dino_run = Trueself.dino_jump = Falsedef duck(self):self.image = self.duck_img[self.step_index // 5]self.dino_rect = self.image.get_rect()self.dino_rect.x = self.X_POSself.dino_rect.y = self.Y_POS_DUCKself.step_index += 1def run(self):self.image = self.run_img[self.step_index // 5]self.dino_rect = self.image.get_rect()self.dino_rect.x = self.X_POSself.dino_rect.y = self.Y_POSself.step_index += 1def jump(self):self.image = self.jump_imgif self.dino_jump:self.dino_rect.y -= self.jump_vel * 4self.jump_vel -= 0.8if self.jump_vel < - self.JUMP_VEL:self.dino_jump = Falseself.jump_vel = self.JUMP_VELdef draw(self, SCREEN):SCREEN.blit(self.image, (self.dino_rect.x, self.dino_rect.y))class Cloud:def __init__(self):self.x = SCREEN_WIDTH + random.randint(800, 1000)self.y = random.randint(50, 100)self.image = CLOUDself.width = self.image.get_width()def update(self):self.x -= game_speedif self.x < -self.width:self.x = SCREEN_WIDTH + random.randint(2500, 3000)self.y = random.randint(50, 100)def draw(self, SCREEN):SCREEN.blit(self.image, (self.x, self.y))class Obstacle:def __init__(self, image, type):self.image = imageself.type = typeself.rect = self.image[self.type].get_rect()self.rect.x = SCREEN_WIDTHdef update(self):self.rect.x -= game_speedif self.rect.x < -self.rect.width:obstacles.pop()def draw(self, SCREEN):SCREEN.blit(self.image[self.type], self.rect)class SmallCactus(Obstacle):def __init__(self, image):self.type = random.randint(0, 2)super().__init__(image, self.type)self.rect.y = 325class LargeCactus(Obstacle):def __init__(self, image):self.type = random.randint(0, 2)super().__init__(image, self.type)self.rect.y = 300class Bird(Obstacle):def __init__(self, image):self.type = 0super().__init__(image, self.type)self.rect.y = 250self.index = 0def draw(self, SCREEN):if self.index >= 9:self.index = 0SCREEN.blit(self.image[self.index//5], self.rect)self.index += 1def main():global game_speed, x_pos_bg, y_pos_bg, points, obstaclesrun = Trueclock = pygame.time.Clock()player = Dinosaur()cloud = Cloud()game_speed = 20x_pos_bg = 0y_pos_bg = 380points = 0font = pygame.font.Font('freesansbold.ttf', 20)obstacles = []death_count = 0def score():global points, game_speedpoints += 1if points % 100 == 0:game_speed += 1text = font.render("Points: " + str(points), True, (0, 0, 0))textRect = text.get_rect()textRect.center = (1000, 40)SCREEN.blit(text, textRect)def update_high_score(score):high_score = read_high_score()if score > high_score:with open("high_score.txt", "w") as file:file.write(str(score))def background():global x_pos_bg, y_pos_bgimage_width = BG.get_width()SCREEN.blit(BG, (x_pos_bg, y_pos_bg))SCREEN.blit(BG, (image_width + x_pos_bg, y_pos_bg))if x_pos_bg <= -image_width:SCREEN.blit(BG, (image_width + x_pos_bg, y_pos_bg))x_pos_bg = 0x_pos_bg -= game_speedwhile run:for event in pygame.event.get():if event.type == pygame.QUIT:run = FalseSCREEN.fill((255, 255, 255))userInput = pygame.key.get_pressed()player.draw(SCREEN)player.update(userInput)if len(obstacles) == 0:if random.randint(0, 2) == 0:obstacles.append(SmallCactus(SMALL_CACTUS))elif random.randint(0, 2) == 1:obstacles.append(LargeCactus(LARGE_CACTUS))elif random.randint(0, 2) == 2:obstacles.append(Bird(BIRD))for obstacle in obstacles:obstacle.draw(SCREEN)obstacle.update()if player.dino_rect.colliderect(obstacle.rect) and player.dino_rect.right >= obstacle.rect.left:pygame.time.delay(2000)death_count += 1update_high_score(points)menu(death_count)background()cloud.draw(SCREEN)cloud.update()score()clock.tick(30)pygame.display.update()def read_high_score():try:with open("high_score.txt", "r") as file:high_score = int(file.read())except FileNotFoundError:high_score = 0return high_scoredef menu(death_count):high_score = read_high_score()global pointsrun = Truewhile run:SCREEN.fill((255, 255, 255))font = pygame.font.Font('freesansbold.ttf', 30)if death_count == 0:text = font.render("Press any Key to Start", True, (0, 0, 0))elif death_count > 0:text = font.render("Press any Key to Restart", True, (0, 0, 0))score = font.render("Your Score: " + str(points), True, (0, 0, 0))scoreRect = score.get_rect()scoreRect.center = (SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2 + 50)SCREEN.blit(score, scoreRect)textRect = text.get_rect()textRect.center = (SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2)high_score_text = font.render("High Score: " + str(high_score), True, (0, 0, 0))high_score_rect = high_score_text.get_rect()high_score_rect.center = (SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2 + 80)SCREEN.blit(high_score_text, high_score_rect)SCREEN.blit(text, textRect)SCREEN.blit(RUNNING[0], (SCREEN_WIDTH // 2 - 20, SCREEN_HEIGHT // 2 - 140))pygame.display.update()for event in pygame.event.get():if event.type == pygame.QUIT:run = Falseif event.type == pygame.KEYDOWN:main()menu(death_count=0)