定义一个Person类,私有成员int age,string &name,定义一个Stu类,包含私有成员double *score,写出两个类的构造函数、析构函数、拷贝构造和拷贝赋值函数,完成对Person的运算符重载(算术运算符、条件运算符、逻辑运算符、自增自减运算符、插入/提取运算符)
#include <iostream>using namespace std;class Person{int age;string &name;
public:Person(string &name):age(15),name(name){}Person(int age, string &name):age(age),name(name){}~Person(){cout << "Person的析构函数" << endl;}Person(const Person &other):age(other.age),name(other.name){}Person &operator=(const Person &other){age = other.age;name = other.name;return *this;}Person operator+(const Person other){string tempname = name + other.name;int tempage = age + other.age;return Person(tempage, tempname);}bool operator==(const Person other){return age==other.age && name==other.name;}bool operator>=(const Person other){return age>=other.age && name>=other.name;}Person operator++(){age++;return *this;}Person operator++(int){return Person(age++, name);}Person operator--(){age--;return *this;}Person operator--(int){return Person(age--, name);}friend istream &operator>>(istream &cin, Person &p);friend ostream &operator<<(ostream &cout, Person &p);
};istream &operator>>(istream &cin, Person &p){cin >> p.age >> p.name;return cin;
}ostream &operator<<(ostream &cout, Person &p){cout << "age=" << p.age << ", name=" << p.name;return cout;
}class Stu{double * const score;
public:Stu():score(new double(12.0)){}Stu(double score):score(new double(score)){}~Stu(){delete score;cout << "Stu的析构函数" << endl;}Stu(Stu &other):score(new double(*(other.score))){}Stu &operator=(Stu &other){*score = *other.score;return *this;}void show(){cout << *score << endl;}
};int main()
{string name1 = "a";Person p1(name1);cin >> p1;Person p2 = p1;cout << p2 << endl;p2++;cout << p2 << endl;string name2 = "b";Person p3(name2);cout << p3 << endl;p3 = p2 + p1;cout << boolalpha << (p2 == p1) << endl;cout << (p2 >= p1) << endl;cout << p3 << endl;cout << "-----------" << endl;Stu s1;s1.show();Stu s2(16.5);s2.show();Stu s3 = s2;s3.show();s2 = s1;s2.show();return 0;
}
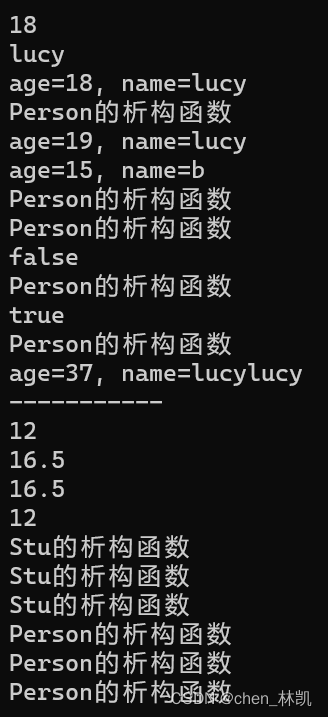
思维导图
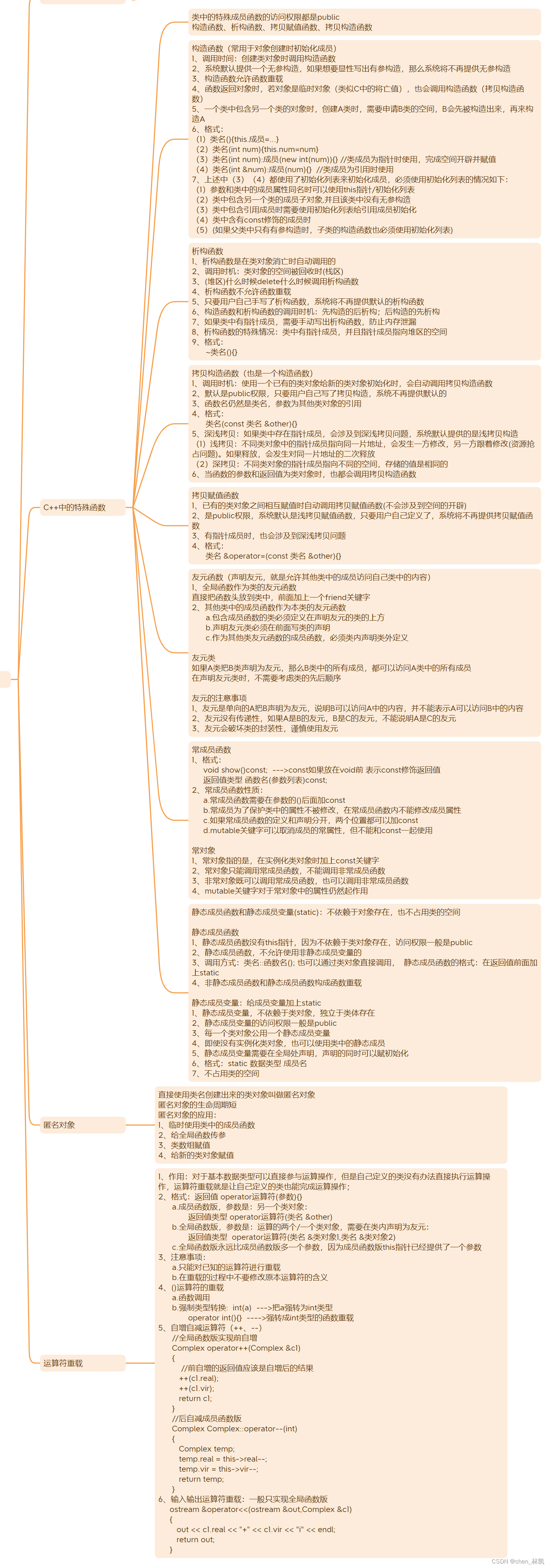