cesium广告牌
Description:
描述:
This is a standard dynamic programing problem of finding maximum profits with some constraints. This can be featured in any interview coding rounds.
这是在某些约束条件下找到最大利润的标准动态编程问题。 这可以在任何采访编码回合中体现。
Problem statement:
问题陈述:
Consider a highway of M miles. The task is to place billboards on the highway such that revenue is maximized. The possible sites for billboards are given by number x1 < x2 < ... < x(n-1) < xn specifying positions in miles measured from one end of the road. If we place a billboard at position xi, we receive a revenue of ri > 0. The constraint is that no two billboards can be placed within t miles or less than it.
考虑一条M英里的高速公路。 任务是在高速公路上放置广告牌,以使收入最大化。 广告牌的可能位置由数字x 1 <x 2 <... <x (n-1) <x n给出,指定从道路的一端算起的英里位置。 如果将广告牌放置在x i位置,我们的收入r i > 0 。 约束条件是在t英里以内或小于t英里内不能放置两个广告牌。
Input:
M=15, n=5
x[n]= {6,8,12,14,15}
revenue[n] = {3,6,5,3,5}
t = 5
Output:
11
Explanation with example
举例说明
So, we have to maximize the revenue by placing the billboards where the gap between any two billboard is t.
因此,我们必须通过将广告牌放置在任意两个广告牌之间的距离为t的位置来最大化收入。
Here,
这里,
The corresponding distances of the billboards with respect to the origin are,
广告牌相对于原点的相应距离为
x[5]= {6,8,12,14,15}
We can augment the origin, so the augmented array becomes: (no need to augment if origin revenue exists)
我们可以扩充原点,因此扩充后的数组将变为:(如果存在原点收入,则无需扩充)
x[6]= {0,6,8,12,14,15}
t=5
The graphical interpretation of the billboards is like following:
广告牌的图形解释如下:
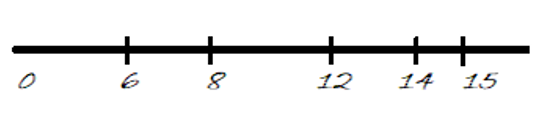
Figure 1: Billboards
图1:广告牌
The augmented revenue array:
扩充收益阵列:
revenue[6] = {0,3,6,5,3,5}
Now, the brute force approach can be to check all the possible combination of billboards and updating the maximum revenue accumulated from each possible combinations.
现在,暴力破解方法可以是检查广告牌的所有可能组合,并更新每个可能组合所累积的最大收益。
Few of such possible combinations can be:
这样的可能组合很少是:
So, maximum revenue that can be collected is 11.
因此,可以收取的最大收入为11 。
For any billboard bi we have three choices
对于任何广告牌b 我都有三种选择
Don't pick bi
不要选择我
Start from bi
从我开始
Pick bi and other billboards accordingly
相应地选择b i和其他广告牌
Say,
说,
M(i)=maximum revenue upto first i billboards
So,
所以,
M(0)=0
if bi is not picked, M(i)=M(i-1)
if billboard placement starts from bi M(i)=r[i]
If we place bi then need to pace bj where d[i]>d[j]-t such that revenue maximizes
So,
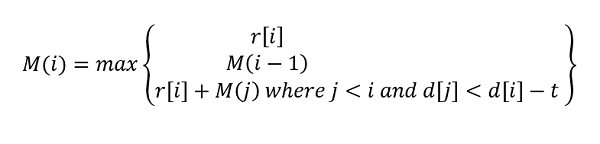
Problem Solution approach
问题解决方法
We convert the above recursion to dynamic programing as there will many overlapping sub problems solving the recursion. (Try to generate the recursion tree yourself)
我们将上述递归转换为动态编程,因为将会有许多重叠的子问题解决递归。 (尝试自己生成递归树)
1) Construct DP[n] for n billboards
// considering billboard placing starts from it
2) Fill the array with base value which is r[i]
3) for i=1 to n-1
// recursion case 1 and 2
// either not picking ith billboard or starting
// from ith billboard which ever is maximum
dp[i] = max(dp[i-1],dp[i]);
// recursion case 3
// picking ith billboard
for j=0 to i-1
if(a[j]< a[i]-k)//feasible placing
dp[i]= max(dp[i],dp[j]+r[i]);
end for
end for
Result is dp[n-1]
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
int max(int x, int y)
{
return (x > y) ? x : y;
}
int billboard(vector<int> a, vector<int> r, int n, int k)
{
int dp[n];
for (int i = 0; i < n; i++)
dp[i] = r[i];
//base value
dp[0] = r[0];
for (int i = 1; i < n; i++) {
//first two recursion case
int mxn = max(dp[i - 1], dp[i]);
//picking ith billboard, third recursion case
for (int j = 0; j < i; j++) {
if (a[j] < a[i] - k)
mxn = max(mxn, dp[j] + r[i]);
}
dp[i] = mxn;
}
return dp[n - 1];
}
int main()
{
int n, k, item, m;
cout << "Enter highway length:\n";
cin >> m;
cout << "Enter number of billboards\n";
cin >> n;
cout << "Enter minimum distance between any two billboards\n";
cin >> k;
vector<int> a;
vector<int> r;
cout << "Enter billboard distances one by one from origin\n";
for (int i = 0; i < n; i++) {
cin >> item;
a.push_back(item);
}
cout << "Enter revenues for the respective billboards\n";
for (int i = 0; i < n; i++) {
cin >> item;
r.push_back(item);
}
if (a[0] == 0) {
a.insert(a.begin(), 0);
r.insert(r.begin(), 0);
n = n + 1;
}
cout << "Maximum revenue that can be collected is: " << billboard(a, r, n, k) << endl;
return 0;
}
Output
输出量
RUN 1:
Enter highway length:
20
Enter number of billboards
5
Enter minimum distance between any two billboards
5
Enter billboard distances one by one from origin
3 7 12 13 14
Enter revenues for the respective billboards
15 10 1 6 2
Maximum revenue that can be collected is: 21
RUN 2:
Enter highway length:
15
Enter number of billboards
5
Enter minimum distance between any two billboards
5
Enter billboard distances one by one from origin
6 8 12 14 15
Enter revenues for the respective billboards
3 6 5 3 5
Maximum revenue that can be collected is: 11
翻译自: https://www.includehelp.com/icp/highway-billboard.aspx
cesium广告牌