目录
一、数组
1、数组的声明
1.1 一维数组声明:
1.2 多维数组声明:
2、数组的实例化和赋值
2.1 数组在声明时通过在花括号中使用以逗号分隔的数据项对数组赋值, 例如:
2.2 如果在声明后赋值,则需要使用new
2.3 C# 也支持将new 作为声明语句的一部分, 例如:
2.4 在new 关键字作为数组赋值的一部分,可以同时在括号内指定数组的大小
2.5 在为数组分配空间时可以不指定初始值。 例如:
2.6 分配数组但不指定初始值仍会初始化每个元素,每个元素都将初始化成默认值
2.8 类似一维数组,多维数组也能声明的时候初始化 或者仅分配空间, 例如:
2.9 多维数组每一维的大小必须一致,例如下列声明是错误的
2.10 在多维数组中,元素是用每个维上的一个索引来标识的, 例如:
3、交错数组
3.1 交错数组是指数组的数组,它不要求数组的每一维大小一致,但需要为内部的每个数组都创建数组实例。例如:
3.2 交错数组中元素是用每个维上的一个索引来标识的, 例如:
4、获取数组长度和维度
4.1 数组的长度是固定的,不能随便更改,除非重新创建数组。此外,数组越界会抛出异常
4.2 数组的 Length 属性返回数组的长度
4.3 要获得数组特定维的长度需要使用GetLength() 方法,调用时要指定返回哪一维的长度,还可以使用Rank 成员获取整个数组的维度, 例如:
5、数组方法
数组提供了更多的方法来操纵数组中的元素,例如 Sort, BinarySearch 等:
二、操作符
1、操作符
1.1 操作符通常操作符分为3大类:一元操作符、二元操作符、三元操作符
1.2 一元操作符正负(+/-)。 例:decimal debt = -18125876697430.99M
1.3 二元算术操作符
1.4 加法操作符用于字符串
1.5 加法操作符可用来拼接两个或更多字符
1.6 在算术运算中可以直接操作字符, 例如:
2、浮点数
2.1 比较两个浮点数是否相等时,浮点类型的不准确性可能造成严重的后果
2.2 避免将二进制浮点数用于相等性条件式。要么判断两个值之差是否在容差范围内,要么使用 decimal 类型
2.3 C#中,浮点数0除以0将会得到 “NaN”; 当浮点向上溢出时,将会得到Infinity,向下溢出将会得到 –Infinity
3、复合赋值操作符
3.1 C#中同样支持下列复合赋值操作符:
3.2 前缀操作符和后缀操作符例子:
3.3 常量表达式和常量符号
3.4 常量表达式是指C# 编译时能求值的表达式
3.5 值为常量表达式的符号称为常量符号。常量符号的值在生命周期内不能被改变。C#中使用 const 声明常量符号
三、控制流
1、if 语句
1.1 语法形式
1.2 if 语句中的条件称为布尔表达式,不同于C++, C# 要求条件必须是布尔类型
1.3 嵌套if 语句
2、switch 语句
2.1 语法形式
3、关系操作符
3.1 关系和相等型操作符编辑
3.2 使用相等操作符例子
4、逻辑布尔操作符
4.1 OR 操作符 (||)
4.2 AND (&&)操作符
4.3 XOR (^) 操作符
4.4 逻辑反(!)操作符
5、条件及空结合操作符
5.1 条件运算符语法:
5.2 C# 要求条件运算符的 consequence 和alternative 类型一致,而且在判定类型时不会检查表达式上下文。
5.3 空结合运算符 (??) (C# 6.0) expr1 ?? expr2
5.4 表示如果expr1 不为null,返回expr1的值,否则返回expr2的值。
5.5 空结合运算符 (??) 能组成调用链,
6、null 条件操作符
6.1 在调用值为null的方法时,程序将抛出System.NullReferenceException 异常
6.2 null 条件运算符(C# 6.0) 在调用方法或属性之前检查操作数是否为null,如果为null 则忽略调用直接返回null
6.3 当调用成员返回是一个值类型时,null 条件运算符总是返回该类型可为空版本,
6.4 null条件操作符可以组成调用链。
6.5 null 条件运算符 也可以和索引操作符结合使用
7、位操作符
7.1 移位操作符
7.2 按位操作符
8、循环
8.1 while 语法
8.2 do/while 语法
8.3 for 循环
8.4 所有循环的条件必须是布尔表达式
8.5 foreach 循环
8.5.1 foreach 遍历数据项集合,设置循环变量来依次表示每一项,语法形式如下:
8.5.2 例子:
9、C#预处理指令
10、错误和警告
10.1 C# 允许在代码中插入 #error 和 #warning 指令分别来生成错误和警告信息, 例如:
10.2 C#编译器提供了预处理指令#pragma 来关闭或还原警告
11、#region
11.1 C# 允许使用#region指令展开或折叠代码区域 ,当这组代码被折叠起来的时候,我们可以看到#region后面的说明文字。
11.2 #region和#endregion 必须成对使用,两个指令都可以选择在指令后面跟随一个描述性字符
11.3 #region 块不能与 #if块重叠。但是,可以将 #region 块嵌套在 #if 块内,或将 #if 块嵌套在 #region 块内。
一、数组
1、数组的声明
1.1 一维数组声明:
T[] 表示T类型元素的数组,元素的索引值范围从0 到 size -1,可以使用下标运算符[]访问数组中元素, 例如:string[] languages
1.2 多维数组声明:
T[, …, ] 表示T类型元素的多维数组,数组的维数等于逗号数加1, 例如:int[, ] cells
2、数组的实例化和赋值
2.1 数组在声明时通过在花括号中使用以逗号分隔的数据项对数组赋值, 例如:
string[] languages={ "C#", "COBOL", "JAVA", "C++"};
2.2 如果在声明后赋值,则需要使用new
string[] languages;
languages = new string[]{ "C#", "COBOL", "JAVA", "C++"};
2.3 C# 也支持将new 作为声明语句的一部分, 例如:
string[] languages= new string[]{ "C#", "COBOL", "JAVA", "C++"};
2.4 在new 关键字作为数组赋值的一部分,可以同时在括号内指定数组的大小
string[] languages= new string[4]{ "C#", "COBOL", "JAVA", "C++"};
2.5 在为数组分配空间时可以不指定初始值。 例如:
string[] languages = new string[9];
2.6 分配数组但不指定初始值仍会初始化每个元素,每个元素都将初始化成默认值
- 引用类型初始化为null
- 数值类型初始化为0
- bool 初始化为 false
- char 初始化为 \0
- 非基础值类型以递归方式初始化,每个字段被初始化为默认值
2.8 类似一维数组,多维数组也能声明的时候初始化 或者仅分配空间, 例如:
int[,] cells = { {1, 0, 2}, {1, 2, 0}, {1, 2, 1} };
Int[,] arr = int[3,3];
2.9 多维数组每一维的大小必须一致,例如下列声明是错误的
int[,] cells = { {1, 0, 2, 0}, {1, 2, 0}, {1, 2}, {1}};
2.10 在多维数组中,元素是用每个维上的一个索引来标识的, 例如:
int[,] cells = { {1, 0, 2}, {1, 2, 0}, {1, 2, 1} };
cells[1, 0] = 1;
3、交错数组
3.1 交错数组是指数组的数组,它不要求数组的每一维大小一致,但需要为内部的每个数组都创建数组实例。例如:
int[][] cells = {new int[] {1, 0, 2, 0}, new int[] {1, 2, 0}, new int[] {1, 2}, new int[] {1}};
3.2 交错数组中元素是用每个维上的一个索引来标识的, 例如:
int[][] cells = { new int[] {1, 0, 2}, new int[] {1, 2, 0}, new int[] {1, 2, 1}}; cells[1][0] = 1; // ...
4、获取数组长度和维度
4.1 数组的长度是固定的,不能随便更改,除非重新创建数组。此外,数组越界会抛出异常
4.2 数组的 Length 属性返回数组的长度
- 对于一维和多维数组,它返回数组中元素的总数
- 对于交错数组,它返回最外层数组的元素数
4.3 要获得数组特定维的长度需要使用GetLength() 方法,调用时要指定返回哪一维的长度,还可以使用Rank 成员获取整个数组的维度, 例如:
bool[, ,] cells;
cells = new bool[2, 3, 3];
System.Console.WriteLine(cells.GetLength(0)); // Displays 2
System.Console.WriteLine(cells.Rank); // Displays 3
5、数组方法
数组提供了更多的方法来操纵数组中的元素,例如 Sort, BinarySearch 等:
public static void Main()
{ string[] languages = new string[] { "C#", "COBOL", "Java", "C++", "Visual Basic", "Pascal", "Fortran", "Lisp", "J#" };System.Array.Sort(languages);string searchString = "COBOL";int index = System.Array.BinarySearch(languages, searchString);System.Console.WriteLine("The wave of the future, " + $"{ searchString}, is at index {index }.");System.Console.WriteLine();System.Console.WriteLine($"{"First Element",-20 }\t{"Last Element", -20 }"); System.Console.WriteLine($"{"-------------",-20 }\t{"------------",-20 } "); System.Console.WriteLine($"{ languages[0],-20 }\t{ languages[languages.Length - 1],-20 } "); System.Array.Reverse(languages); System.Console.WriteLine($"{ languages[0],-20 }\t{ languages[languages.Length - 1],-20 }"); // Note this does not remove all items from the array. Rather it sets each item to the type’s default value. System.Array.Clear(languages, 0, languages.Length); System.Console.WriteLine($"{ languages[0],-20 }\t{ languages[languages.Length - 1],-20 }"); System.Console.WriteLine($"After clearing, the array size is: { languages.Length }");
}
二、操作符
1、操作符
1.1 操作符通常操作符分为3大类:一元操作符、二元操作符、三元操作符
1.2 一元操作符正负(+/-)。 例:decimal debt = -18125876697430.99M
1.3 二元算术操作符
- *、 / 和 % 具有最高优先级
- + 和 – 具有较低优先级
- = 优先级最低
1.4 加法操作符用于字符串
1.5 加法操作符可用来拼接两个或更多字符
public static void Main()
{ short windSpeed = 42; Console.WriteLine("The original Tacoma Bridge in Washington\nwas " + "brought down by a " + windSpeed + " mile/hour wind.");
}
1.6 在算术运算中可以直接操作字符, 例如:
public static void Main()
{ int n = '3' + '4';char c = (char)n;System.Console.WriteLine(c); // Writes out g.int a = '3';System.Console.WriteLine($"0x{a:x}");
}
2、浮点数
2.1 比较两个浮点数是否相等时,浮点类型的不准确性可能造成严重的后果
public static void Main()
{ decimal decimalNumber = 4.2M; double doubleNumber1 = 0.1F * 42F; double doubleNumber2 = 0.1D * 42D; float floatNumber = 0.1F * 42F; Console.WriteLine($"{decimalNumber} != {(decimal)doubleNumber1}"); Console.WriteLine($"{(double)decimalNumber} != {doubleNumber1}"); Console.WriteLine($"(float){(float)decimalNumber}M != {floatNumber}F"); Console.WriteLine($"{doubleNumber1} != {(double)floatNumber}"); Console.WriteLine($"{doubleNumber1} != {doubleNumber2}"); Console.WriteLine($"{floatNumber}F != {doubleNumber2}D"); Console.WriteLine($"{(double)4.2F} != {4.2D}"); Console.WriteLine($“{4.2F}F != {4.2D}D");
}
2.2 避免将二进制浮点数用于相等性条件式。要么判断两个值之差是否在容差范围内,要么使用 decimal 类型
2.3 C#中,浮点数0除以0将会得到 “NaN”; 当浮点向上溢出时,将会得到Infinity,向下溢出将会得到 –Infinity
public static void Main()
{ System.Console.WriteLine(0 / 0); // Displays: -Infinity System.Console.WriteLine(-1f / 0); // Displays: Infinity System.Console.WriteLine(3.402823E+38f * 2f);
}
3、复合赋值操作符
3.1 C#中同样支持下列复合赋值操作符:
*=expr /=expr %=expr +=expr -=expr ++expr expr ++ --expr expr--
3.2 前缀操作符和后缀操作符例子:
public static void Main()
{ int x = 123; // Displays 123, 124, 125. Console.WriteLine($"{x++}, {x++}, {x}"); // x now contains the value 125. // Displays 126, 127, 127. Console.WriteLine($"{++x}, {++x}, {x}"); // x now contains the value 127.
}
3.3 常量表达式和常量符号
3.4 常量表达式是指C# 编译时能求值的表达式
3.5 值为常量表达式的符号称为常量符号。常量符号的值在生命周期内不能被改变。C#中使用 const 声明常量符号
public static void Main()
{ const int secondsPerDay = 60 * 60 * 24; const int secondsPerWeek = secondsPerDay * 7; // ...
}
三、控制流
1、if 语句
1.1 语法形式
if(contidition)consequence-statement
else alternative-statement
1.2 if 语句中的条件称为布尔表达式,不同于C++, C# 要求条件必须是布尔类型
1.3 嵌套if 语句
public static void Main()
{if(input < 0)System.Console.WriteLine("Exiting...");else if(input < 9)System.Console.WriteLine($"Tic-tac-toe has more than {input}" + " maximum turns.");else if (input > 9)System.Console.WriteLine($"Tic-tac-toe has less than {input}" + " maximum turns.");elseSystem.Console.WriteLine("Correct, tic-tac-toe has a maximum " + " of 9 turns.");
}
public static int input { get; set; }
2、switch 语句
2.1 语法形式
switch (expr )
{case constant: statements default: statements }
expr的类型决定了switch 的“主导类型”(governing type).允许的主导类型包括:
- bool, sbyte, byte, short, ushort, int, uint, long, ulong, char, enum 类型 以及上述类型的可空
- 类型和string
constant: 与主导类型兼容的常量表达
3、关系操作符
3.1 关系和相等型操作符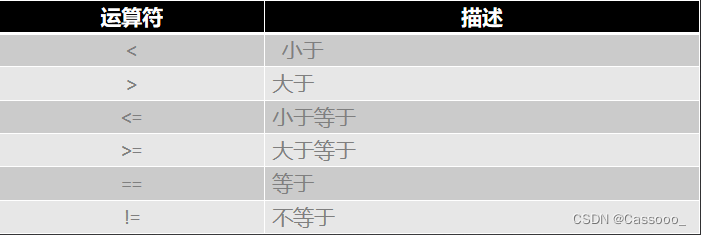
3.2 使用相等操作符例子
public static void Main()
{if(input == "" || input == "quit"){Console.WriteLine($"Player {currentPlayer} quit!!");}
}
public static string input { get; set; }
4、逻辑布尔操作符
4.1 OR 操作符 (||)
- || 对两个表达式求值, 如果其中任一个为true, 就返回true
- || 操作符是从左往右求值的,如果左边为true,则右表达式被忽略
4.2 AND (&&)操作符
- && 在两个表达式都为true的情况下才返回true
- &&操作符也是从左往右求值的,如果左边为false,则右表达式被忽略
4.3 XOR (^) 操作符
- ^操作符在两个表达式值互异的情况下才返回true.
- ^操作符总是对两个表达式求值
4.4 逻辑反(!)操作符
- 用于反转一个布尔类型数值,例如:bool valid = false;bool result = !valid;
5、条件及空结合操作符
5.1 条件运算符语法:
- condition ? consequence : alternative;
5.2 C# 要求条件运算符的 consequence 和alternative 类型一致,而且在判定类型时不会检查表达式上下文。
- 例如:f? “abc” : 123 ; // illegal expr
5.3 空结合运算符 (??) (C# 6.0) expr1 ?? expr2
5.4 表示如果expr1 不为null,返回expr1的值,否则返回expr2的值。
- 例如:string file_name = file_name?? “default.txt”;
5.5 空结合运算符 (??) 能组成调用链,
- 例如:x ?? y ?? z;
6、null 条件操作符
6.1 在调用值为null的方法时,程序将抛出System.NullReferenceException 异常
6.2 null 条件运算符(C# 6.0) 在调用方法或属性之前检查操作数是否为null,如果为null 则忽略调用直接返回null
static void Main(string[] args)
{if (args?.Length == null)System.Console.WriteLine("ERROR: File missing. "+ "Use:\n\tfind.exe file:<filename>");else{if (args[0]?.ToLower().StartsWith("file:")??false){string fileName = args[0]?.Remove(0, 5);// ...}}
}
6.3 当调用成员返回是一个值类型时,null 条件运算符总是返回该类型可为空版本,
- 例如:args?.Length 返回 int ?
6.4 null条件操作符可以组成调用链。
例如:args[0]?.ToLower().StartsWith("file:")
6.5 null 条件运算符 也可以和索引操作符结合使用
public static void Main(string[] args)
{// CAUTION: args?.Length not verified.string directoryPath = args?[0];string searchPattern = args?[1];// ...
}
7、位操作符
7.1 移位操作符
- 和C++ 一样,C#支持 << >> <<= >>= 操作符,例如: int x= -7 >>2;
7.2 按位操作符
- C#支持 ~,& ,| , ^, 及 &= ,|= , ^= 操作符
public static void Main()
{const int size = 64;ulong value;char bit;System.Console.Write("Enter an integer: ");value = (ulong)long.Parse(System.Console.ReadLine());// Use long.Parse() to support negative numbers ulong mask = 1UL << size - 1;// Set initial mask to 100....for(int count = 0; count < size; count++){bit = ((mask & value) != 0) ? '1' : '0';System.Console.Write(bit);mask >>= 1;// Shift mask one location over to the right}System.Console.WriteLine();
}
8、循环
8.1 while 语法
while (condition)
{ statement;
}
8.2 do/while 语法
do
{ statement
}while(condition);
8.3 for 循环
for (initial; condition; loop)
{ statement;
}
8.4 所有循环的条件必须是布尔表达式
8.5 foreach 循环
8.5.1 foreach 遍历数据项集合,设置循环变量来依次表示每一项,语法形式如下:
foreach(type variable in collection)
{statement;
}
- type:collection每一项的variable 的数据类型,可将类型设置为var
- variable:只读变量,foreach循环自动将collection中下一项赋给它
- collection:多个数据项的表达式,如 数组等
- Statement : 循环体语句集合
8.5.2 例子:
public static void Main() // Declares the entry point of the program.
{char[] cells = {'1', '2', '3', '4', '5', '6', '7', '8', '9'};// Write out the initial available movesforeach(char cell in cells){if(cell != 'O' && cell != 'X’)System.Console.Write($"{ cell } ");}
}
9、C#预处理指令
10、错误和警告
10.1 C# 允许在代码中插入 #error 和 #warning 指令分别来生成错误和警告信息, 例如:
public static void Main()
{#warning "Same move allowed multiple times."
}
10.2 C#编译器提供了预处理指令#pragma 来关闭或还原警告
- #pragma warning disable 1030 禁用 #warning指令
- #pragma warning restore 1030 还原 #warning指令
11、#region
- C# 允许使用#region指令展开或折叠代码区域 ,当这组代码被折叠起来的时候,我们可以看到#region后面的说明文字。
- #region和#endregion 必须成对使用,两个指令都可以选择在指令后面跟随一个描述性字符
- #region 块不能与 #if块重叠。但是,可以将 #region 块嵌套在 #if 块内,或将 #if 块嵌套在 #region 块内。