模块是一个文件(.py文件),包含变量,类定义语句和与特定任务相关的功能。预先装有Python的Python模块称为标准库模块。
创建我们的模块
我们将创建一个名为tempConversion.py的模块,该模块将值从F转换为C,反之亦然。
# tempConversion.py to convert between# between Fahrenheit and Centigrade # function to convert F to Cdef to_centigrade(x): return 5 * (x - 32) / 9.0 # function to convert C to Fdef to_fahrenheit(x): return 9 * x / 5.0 + 32 # constants # water freezing temperature(in Celcius)FREEZING_C = 0.0 # water freezing temperature(in Fahrenheit) FREEZING_F = 32.0
现在保存此python文件并创建模块。导入后,该模块可以在其他程序中使用。
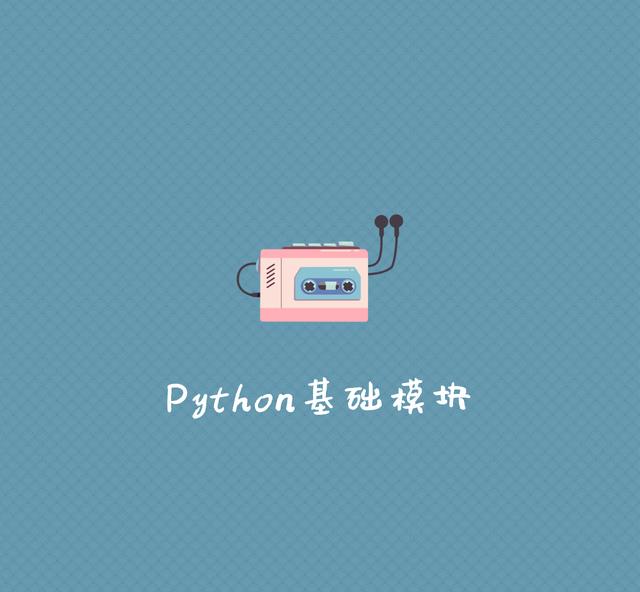
导入模块
在python中,为了使用模块,必须将其导入。Python提供了多种在程序中导入模块的方法:
- 导入整个模块:导入模块名
- 要仅导入模块的特定部分:从module_name导入object_name
- 导入模块的所有对象:从module_name导入*
使用导入的模块
导入模块后,我们可以根据以下语法使用导入模块的任何功能/定义:
module_name.function_name()
这种引用模块对象的方式称为点表示法。
如果我们使用导入函数from,则无需提及模块名称和点号即可使用该函数。
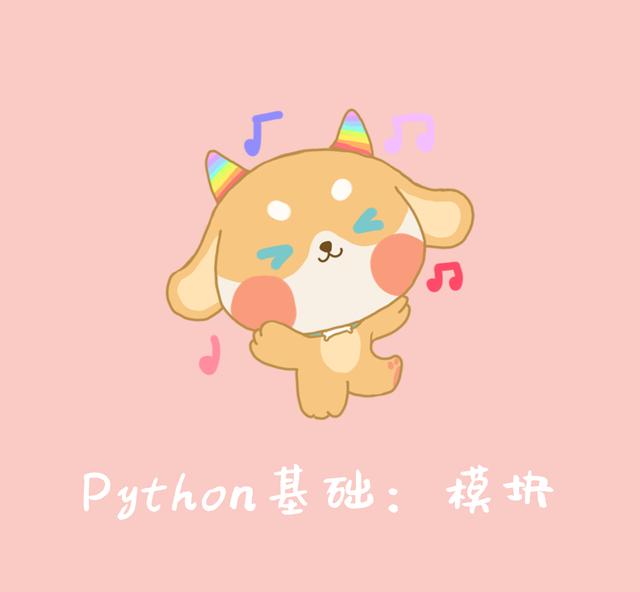
示例1:导入整个模块:
# importing the moduleimport tempConversion # using a function of the moduleprint(tempConversion.to_centigrade(12)) # fetching an object of the moduleprint(tempConversion.FREEZING_F)
输出:
-11.1111111111111132.0
示例2:导入模块的特定组件:
# importing the to_fahrenheit() method from tempConversion import to_fahrenheit # using the imported method print(to_fahrenheit(20)) # importing the FREEZING_C object from tempConversion import FREEZING_C # printing the imported variable print(FREEZING_C)
输出:
68.00.0
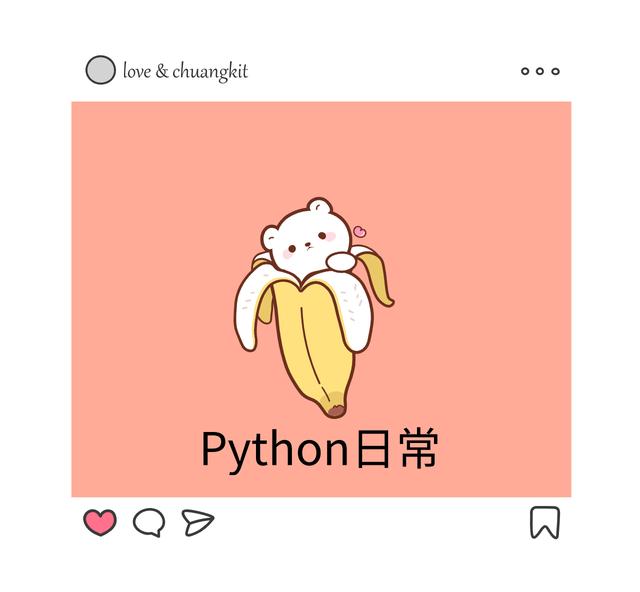
Python标准库功能
python解释器内置了许多始终可用的功能。要使用python的这些内置函数,请直接调用函数,例如function_name()。一些内置的库函数是:input(),int(),float()等
num = 5print("Number entered = ", num) # oct() converts to octal number-stringonum = oct(num) # hex() coverts to hexadecimal number-string hnum = hex(num) print("Octal conversion yields", onum)print("Hexadecimal conversion yields", hnum)print(num)
输出:
输入的数字= 5八进制转换得出0o5十六进制转换产生0x55