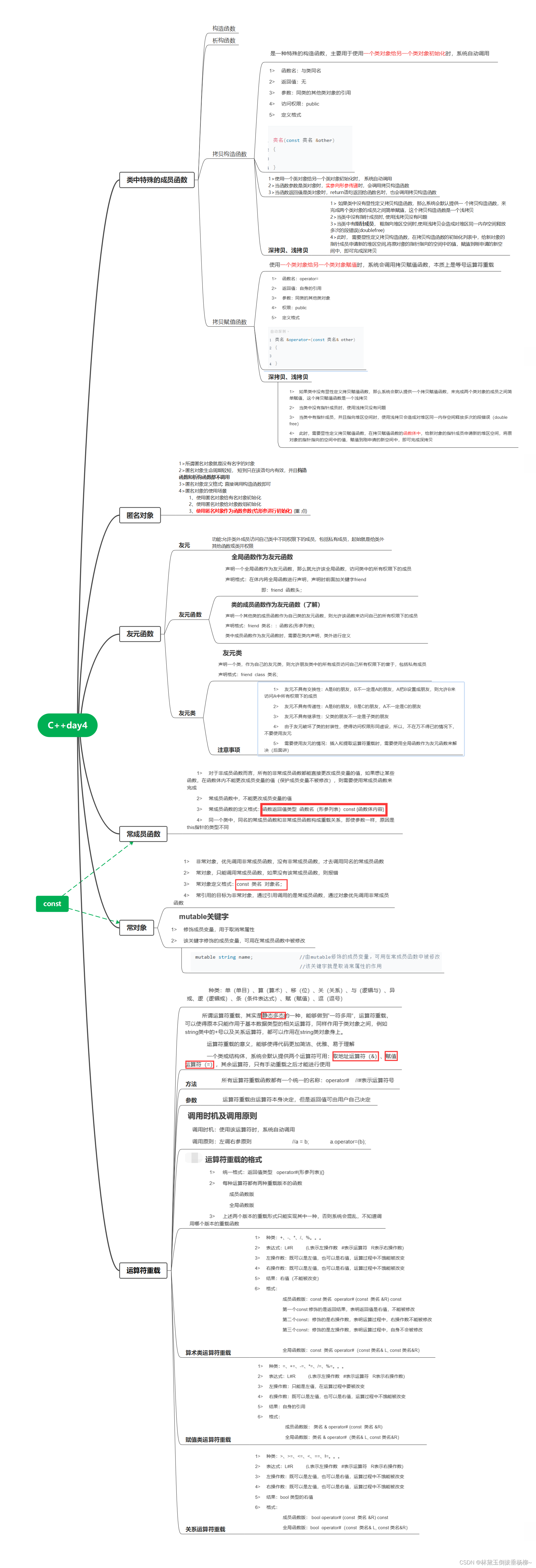
#include <iostream>
#include <cstring>
using namespace std;class mystring
{
private:char *str; //记录C风格字符串int size; //记录字符串的实际长度public://无参构造mystring():size(10){str=new char[size];//构造出一个长度为10的字符串strcpy(str,"");//赋值为空串}//有参构造mystring(const char *s){size=strlen(s);str=new char[size+1];strcpy(str,s);}//拷贝构造mystring (const mystring &other):str(new char(*(other.str))),size(other.size){strcpy(this->str,other.str);}//析构函数~mystring(){delete []str;str=nullptr;}//拷贝赋值函数mystring &operator=(const mystring &other){if(this!=&other){this->size=other.size;this->str=new char[size+1];strcpy(str,other.str);}return *this;}//判空函数bool mystring_empty(char *s){if(strlen(s)==0)return 1;elsereturn 0;}//size函数void mystring_size(){cout<<strlen(str)<<endl;}//c_ str函数char *c_str(){return str;}//at函数char &mystring_at(int pos){return this->str[pos];}//加号运算符重载const mystring operator+(const mystring &R)const{mystring temp;strcpy(temp.str,strcat(this->str,R.str));return temp;}//关系运算符重载(>)bool operator>(const mystring &R)const{if(strcmp(this->str,R.str)>=0)return 1;elsereturn 0;}//加等于运算符重载mystring &operator+= (const mystring &R){strcat(this->str,R.str);return *this;}void show(){cout<<str<<endl;}};
int main()
{mystring s1("hello");s1.show();s1.mystring_size();cout<<s1.mystring_at(2)<<endl;mystring s2;s2=s1;s2.show();mystring s3=s2+s1;s3.show();mystring s4;s4+=s1;s4.show();if(s3>s1){cout<<"yes"<<endl;}elsecout<<"no"<<endl;return 0;
}