1. 业务代码
- 数据表结构
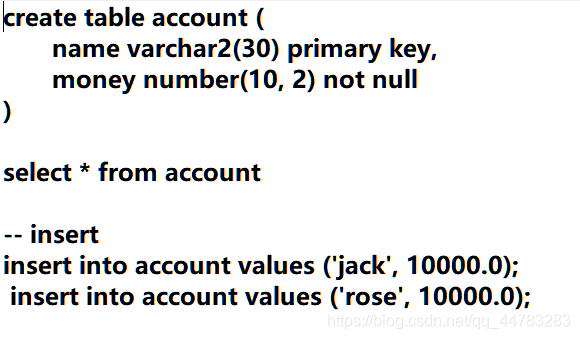
- dao
package com.lovely.dao.impl;import com.lovely.dao.AccountDao;
import org.springframework.jdbc.core.JdbcTemplate;
public class AccountDaoImpl implements AccountDao {private JdbcTemplate jdbcTemplate;public void setJdbcTemplate(JdbcTemplate jdbcTemplate) {this.jdbcTemplate = jdbcTemplate;}public void out(String outMan, Double money) {String sql = "update account set money = money - ? where name = ?";jdbcTemplate.update(sql, new Object[]{money, outMan});}public void in(String inMan, Double money) {String sql = "update account set money = money + ? where name = ?";jdbcTemplate.update(sql, new Object[]{money, inMan});}
}
package com.lovely.service.impl;import com.lovely.dao.AccountDao;
import com.lovely.service.AccountService;
public class AccountServiceImpl implements AccountService {private AccountDao accountDao;public void setAccountDao(AccountDao accountDao) {this.accountDao = accountDao;}public void transfer(String outMan, String inMan, Double money) {accountDao.in(inMan, money);System.out.println(1/0);accountDao.out(outMan, money);}
}
package com.lovely.controller;import com.lovely.service.AccountService;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class AccountController {public static void main(String[] args) {ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");AccountService accountService = app.getBean(AccountService.class);accountService.transfer("jack", "rose", 1000.0);}
}
2. xml配置
<bean id="tranManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"><property name="dataSource" ref="dataSource" /></bean><tx:advice id="txAdvice" transaction-manager="tranManager"><tx:attributes><tx:method name="*"/></tx:attributes></tx:advice><aop:config><aop:advisor advice-ref="txAdvice" pointcut="execution(* com.lovely.service.impl.*.*(..))"/></aop:config>
3. 注解配置
- 注解配置
<bean id="tranManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"><property name="dataSource" ref="dataSource" /></bean><tx:annotation-driven transaction-manager="tranManager"></tx:annotation-driven>
- 事务注解
package com.lovely.service.impl;import com.lovely.dao.AccountDao;
import com.lovely.service.AccountService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;@Service("accountServiceImpl")
public class AccountServiceImpl implements AccountService {@Autowiredprivate AccountDao accountDao;@Transactional(timeout = 1)public void transfer(String outMan, String inMan, Double money) {accountDao.in(inMan, money);System.out.println(1/0);accountDao.out(outMan, money);}
}