一、http含义的介绍
1.http协议是超文本传输协议--具体含义请百度
2.基于tcp/ip协议--注意和udp的区别
3.无状态---本次请求记不住以往请求的状态
4.无连接--每次连接只处理一个请求
5.媒体独立
二、请求报文的组成部分
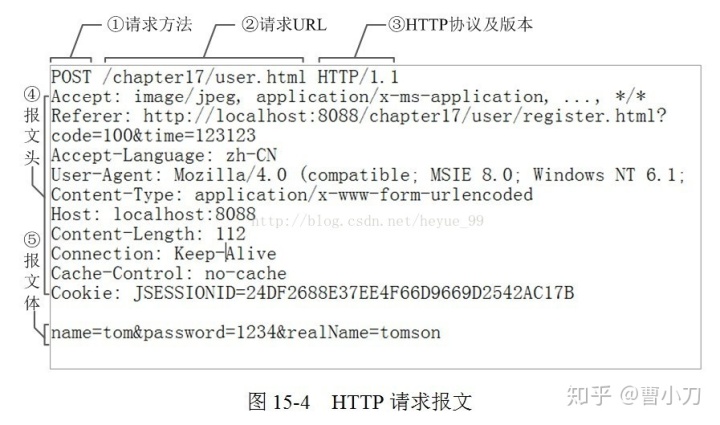
请求行 请求方式 请求url http协议版本
请求头
关于请求头和响应头的信息可以参考以下信息
https://www.cnblogs.com/yumo1627129/p/7941220.html
请求体
post编码方式
表格方式:application/x-www-form-urlencoded; charset=UTF-8
json格式:application/json
多媒体文件格式:multipart/form-data
三、java发送http请求(多种方式,目前只是用了一种方式,所以只记录一种)
第一种:使用httpUrlConnection对象
知识:
httpUrlConnection属性
// 设置连接超时时间
connection.setConnectTimeOut(15000);// 设置读取超时时间
connection.setReadTimeOut(15000);// 设置http的请求方式
connection.setRequestMethod("POST");// 设置http的header的信息,可参考《Java 核心技术 卷II》
connection.setRequestProperty("Connection", "Keep-Alive");// 设置是否向 httpUrlConnection 输出,
// 对于post请求,参数要放在 http 正文内,因此需要设为true。
// 默认情况下是false;
connection.setDoOutput(true);// 设置是否从 httpUrlConnection 读入,默认情况下是true;
connection.setDoInput(true);
请求的步骤
//第一步--获取httpUrlConnection的对象
//第二部--设置httpUrlConnection的属性
//第三步--获取读出输出流,写入信息给服务器outputstream
//第三步--获取输入流。获取服务器的信息 inputstream
方式一:
1.get请求:
public static void testGetMethod(String httpUrl){URL url;HttpURLConnection conn = null;InputStream inputStream = null;try {1.创建对象url = new URL(httpUrl);conn = (HttpURLConnection) url.openConnection();2.设置参数conn.setDoInput(true);conn.setRequestMethod("GET");conn.setRequestProperty("Authorization","Bearer eyJhbGciOiJIUzUxMiJ9.eyJzdWIiOiJOekU0WVdRd1pHRXRZVFZpWmkwME1tSmtMV0kzWXpRdE56STJPVEl6TWpjMFpqUTQifQ.MDnJHzEiLlNlwarfPeE8nExnGKfeNnSAprZcJdQMTBxrgePu1fe-0iE1ymn5hexs6xWRng1xJ9pP49QIlNZCIA");3.建立连接但是可以不显示调用connection。int responseCode = conn.getResponseCode();System.out.println(responseCode);if (responseCode ==200){String str="";StringBuffer stringBuffer = new StringBuffer();4.读取返回值inputStream = conn.getInputStream();BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));while ((str =reader.readLine())!=null){stringBuffer.append(str);}//放到数组里System.out.println(stringBuffer);String[] split = Json.fromJsonAsArray(String.class,stringBuffer);System.out.println(split[0]);}} catch (Exception e) {e.printStackTrace();}finally {if (inputStream==null){try {inputStream.close();} catch (IOException e) {e.printStackTrace();}}if (conn==null){conn.disconnect();}}}
2.post请求
表单类型:
public static void interfaceUntil(String path,String data){try {StringBuffer stringBuffer = new StringBuffer();1.创建对象URL url =new URL(path);HttpURLConnection conn = (HttpURLConnection) url.openConnection();2.设置参数// 设置连接输出流为true,默认false (post请求是以流的方式隐式的传递参数)conn.setDoOutput(true);// 设置连接输入流为trueconn.setDoInput(true);// 设置请求方式为postconn.setRequestMethod("POST");// post请求缓存设为falseconn.setUseCaches(false);
// // 设置该HttpURLConnection实例是否自动执行重定向
// conn.setInstanceFollowRedirects(true);// 设置内容的类型,设置为经过urlEncoded编码过的from参数conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded; charset=UTF-8");
// conn.setRequestProperty("accept", "application/xml");
// conn.setRequestProperty("user-agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/77.0.3865.120 Safari/537.36");conn.setRequestProperty("Authorization","Bearer eyJhbGciOiJIUzUxMiJ9.eyJzdWIiOiJaVFl3WkdVeVpUSXRabU13WXkwME1URmhMV0ptTURRdE1EWXhPR1ptWWpZMlpHSXkifQ.q2Yqzu-ZoXTMm3ZjT1Q4j8feXO2GB13ziZiSHQAc1_eAfFdMMVMaWrFA2VtC17WfDFTnCU7iMtky1bVgw30fZQ");//urlConnection的输入流
3.建立连接,可以不显示调用connection方法
4.创建输出函数,post请求体,字节流的形式OutputStream out = conn.getOutputStream();out.write(data.getBytes());out.flush();
5.创建输入流,读取接口写入的返回内容InputStream is = conn.getInputStream();BufferedReader br = new BufferedReader(new InputStreamReader(is));String str = "";while ((str = br.readLine()) != null) {stringBuffer.append(str);}System.out.println(stringBuffer);
// Pager pager = new Pager();
// System.out.println(pager);
// System.out.println(pager.getPageSize());
// System.out.println(pager.getPage());Pager pager = Json.fromJson(Pager.class, stringBuffer);System.out.println(pager);//关闭流is.close();conn.disconnect();System.out.println("完整结束");} catch (Exception e) {e.printStackTrace();}}public static void main(String[] args) {//合并告警idLong alertId =9626414696700001L;StringBuffer stringBuffer = new StringBuffer();stringBuffer.append("alertId=");stringBuffer.append(alertId);stringBuffer.append("&");stringBuffer.append("pager={page:");stringBuffer.append("1");stringBuffer.append(",pageSize:");stringBuffer.append("2");stringBuffer.append("}");注意:以下的形式是不可以采用的必须是上面的表单形式
// HashMap<String, Object> map = new HashMap<>();
// map.put("alertId",alertId);
// Pager pager = new Pager();
// pager.setPage(1L);
// pager.setPageSize(2L);
// map.put("pager",pager);
// System.out.println("传递的json数据:"+Json.toJson(map));interfaceUntil("http://192.168.101.244:16520/sap/alert/alertraw/pager",stringBuffer.toString());}
json类型:
public static void testPostJson(String path,String data){try {URL url = new URL(path);HttpURLConnection conn =(HttpURLConnection) url.openConnection();conn.setDoOutput(true);conn.setDoInput(true);conn.setRequestMethod("POST");conn.setUseCaches(false);conn.setRequestProperty("Content-Type","application/json;charset=UTF-8");conn.setRequestProperty("Authorization","Bearer eyJhbGciOiJIUzUxMiJ9.eyJzdWIiOiJNVFJtTmpreU5UVXROamd6TmkwMFpEVmtMV0V3TURNdE9UTTBOR1V5WWpCaU1XSXgifQ.pPvG67sbbZ-ZNVf8JAiHE6rzgb5nLPgCQgEOlMTYAx6eobB5tF3GnDHg6OwyYM4QPGPCSJ_0hZ0Qo1L1QbqtAQ");OutputStream os = conn.getOutputStream();os.write(data.getBytes());os.flush();
/* //读取客户端响应,变成string答应InputStream is = conn.getInputStream();InputStreamReader isr = new InputStreamReader(is);BufferedReader br = new BufferedReader(isr);int str;char buff[] = new char[1024];while ((str = isr.read(buff)) != -1) {System.out.println(buff);}*/is.close();os.close();conn.disconnect();System.out.println("完整结束");} catch (Exception e) {e.printStackTrace();}}public static void main(String[] args) {/*形式:"id":9575280686300014;代码:Map<String,String> dataMap = new HashMap<>();dataMap.put("id","9575280686300014");testPostJson("http://124.126.208.180:16520/sap/api/rest/alert/drill", Json.toJson(dataMap));*//* 形式{"pager":{"page":2,"pageSize":2}}
*/Pager pager = new Pager();pager.setPage(2L);pager.setPageSize(2L);testPostJson("http://124.126.208.180:16520/sap/api/rest/alert/getAlert",Json.toJson(pager));}
多媒体类型:
public static void postFile(String urlPath, File file, Map<String,Object> valueMap){HttpURLConnection conn;InputStream inputStream;OutputStream outputStream;final String newLine = "rn";final String boundaryPrefix = "--";try {String BOUNDARY="----------"+System.currentTimeMillis();//参数分割线StringBuffer divider = new StringBuffer();divider.append(boundaryPrefix).append(BOUNDARY).append(newLine);//结尾// 定义最后数据分隔线,即--加上BOUNDARY再加上--。StringBuffer end = new StringBuffer();end.append(newLine).append(boundaryPrefix).append(BOUNDARY).append(boundaryPrefix);URL url = new URL(urlPath);conn = (HttpURLConnection) url.openConnection();conn.setDoInput(true);conn.setDoOutput(true);conn.setUseCaches(false);conn.setRequestMethod("POST");conn.setRequestProperty("Content-Type","multipart/form-data;boundary="+ BOUNDARY);String fileName = null;String fileValue = null;/*** 开始传递非文件参数* 参数分割符号-content内容-两个空行-正文参数内容-结束标记*/StringBuffer stringBuffer = new StringBuffer();stringBuffer.append(divider);stringBuffer.append("Content-Disposition:form-data;name="fileId"");//两个空行stringBuffer.append(newLine);stringBuffer.append(newLine);//参数stringBuffer.append("789");stringBuffer.append(end);System.out.println("非文件上传参数"+stringBuffer);outputStream = conn.getOutputStream();outputStream.write(stringBuffer.toString().getBytes());/*开始文件上传*/StringBuilder sb = new StringBuilder();sb.append(divider);// 文件参数,photo参数名可以随意修改sb.append("Content-Disposition: form-data;name="file";filename="" + file.getName() + """);sb.append(newLine);sb.append(newLine);outputStream.write(sb.toString().getBytes());//开始输出文件FileInputStream fileInputStream = new FileInputStream(file);byte[] bytes = new byte[1024];int length;while ((length=fileInputStream.read(bytes)) !=-1){outputStream.write(bytes);}//结束符号outputStream.write(end.toString().getBytes());outputStream.flush();//读取响应inputStream = conn.getInputStream();BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));String str;if ((str = bufferedReader.readLine())!=null){System.out.println(str);}} catch (Exception e) {e.printStackTrace();}}public static void main(String[] args) {File file = new File("D://wecenter//readme_first.html");HashMap<String, Object> map = new HashMap<>();map.put("fileId","123");System.out.println(file.exists());postFile("http://localhost:16520/test/upload",file,map);}
3.关于结果的处理
string类型: 使用reader读出来的本来就是string类型
数组,list类型:

javabean对象:fastjson都可以这类的问题,目前使用的不是fastjson

三、胡思乱想记录中
1.为什么不能直接使用objectOutputStream直接序列化输出结果?
objectOutputstream需要写入和写出的版本号一致,这边很明显没有版本号可以用。
2.fastjson的相关知识有待总结。