http://blog.csdn.net/xieqiaokang/article/details/60780608
1. 函数
用 OpenCV 标注 bounding box 主要用到下面两个工具——cv2.rectangle() 和 cv2.putText()。用法如下:
# cv2.rectangle()
# 输入参数分别为图像、左上角坐标、右下角坐标、颜色数组、粗细
cv2.rectangle(img, (x,y), (x+w,y+h), (B,G,R), Thickness)# cv2.putText()
# 输入参数为图像、文本、位置、字体、大小、颜色数组、粗细
cv2.putText(img, text, (x,y), Font, Size, (B,G,R), Thickness)
- 1
- 2
- 3
- 4
- 5
- 6
- 7
2. Example
import cv2fname = '001.jpg'
img = cv2.imread(fname)
# 画矩形框
cv2.rectangle(img, (212,317), (290,436), (0,255,0), 4)
# 标注文本
font = cv2.FONT_HERSHEY_SUPLEX
text = '001'
cv2.putText(img, text, (212, 310), font, 2, (0,0,255), 1)
cv2.imwrite('001_new.jpg', img)
documents:
https://docs.opencv.org/3.1.0/dc/da5/tutorial_py_drawing_functions.html
Goal
- Learn to draw different geometric shapes with OpenCV
- You will learn these functions : cv2.line(), cv2.circle() , cv2.rectangle(), cv2.ellipse(), cv2.putText() etc.
Code
In all the above functions, you will see some common arguments as given below:
- img : The image where you want to draw the shapes
- color : Color of the shape. for BGR, pass it as a tuple, eg: (255,0,0) for blue. For grayscale, just pass the scalar value.
- thickness : Thickness of the line or circle etc. If **-1** is passed for closed figures like circles, it will fill the shape. default thickness = 1
- lineType : Type of line, whether 8-connected, anti-aliased line etc. By default, it is 8-connected. cv2.LINE_AA gives anti-aliased line which looks great for curves.
Drawing Line
To draw a line, you need to pass starting and ending coordinates of line. We will create a black image and draw a blue line on it from top-left to bottom-right corners.
Drawing Rectangle
To draw a rectangle, you need top-left corner and bottom-right corner of rectangle. This time we will draw a green rectangle at the top-right corner of image.
Drawing Circle
To draw a circle, you need its center coordinates and radius. We will draw a circle inside the rectangle drawn above.
Drawing Ellipse
To draw the ellipse, we need to pass several arguments. One argument is the center location (x,y). Next argument is axes lengths (major axis length, minor axis length). angle is the angle of rotation of ellipse in anti-clockwise direction. startAngle and endAngle denotes the starting and ending of ellipse arc measured in clockwise direction from major axis. i.e. giving values 0 and 360 gives the full ellipse. For more details, check the documentation of cv2.ellipse(). Below example draws a half ellipse at the center of the image.
Drawing Polygon
To draw a polygon, first you need coordinates of vertices. Make those points into an array of shape ROWSx1x2 where ROWS are number of vertices and it should be of type int32. Here we draw a small polygon of with four vertices in yellow color.
- Note
- If third argument is False, you will get a polylines joining all the points, not a closed shape.
- cv2.polylines() can be used to draw multiple lines. Just create a list of all the lines you want to draw and pass it to the function. All lines will be drawn individually. It is a much better and faster way to draw a group of lines than calling cv2.line() for each line.
Adding Text to Images:
To put texts in images, you need specify following things.
- Text data that you want to write
- Position coordinates of where you want put it (i.e. bottom-left corner where data starts).
- Font type (Check cv2.putText() docs for supported fonts)
- Font Scale (specifies the size of font)
- regular things like color, thickness, lineType etc. For better look, lineType = cv2.LINE_AA is recommended.
We will write OpenCV on our image in white color.
Result
So it is time to see the final result of our drawing. As you studied in previous articles, display the image to see it.
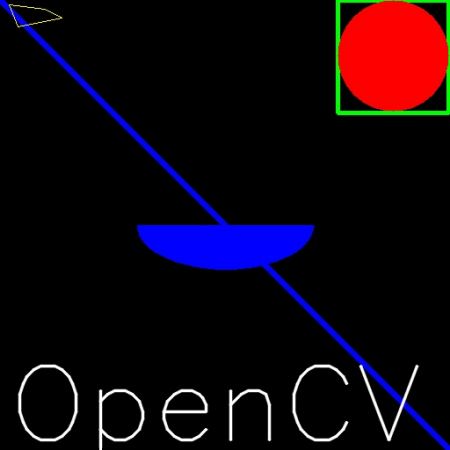
Additional Resources
- The angles used in ellipse function is not our circular angles. For more details, visit this discussion.
Exercises
- Try to create the logo of OpenCV using drawing functions available in OpenCV.