using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Text;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;

namespace Fanfajin.MyWebControls


{

/**//// <summary>
/// 增强的 GridView 控件 扩展
/// </summary>
[DefaultProperty("SelectedValue")]
[ToolboxData("<{0}:MyGridView runat=server></{0}:MyGridView>")]
public sealed class MyGridView : System.Web.UI.WebControls.GridView

{

公开属性成员#region 公开属性成员

/**//// <summary>
/// 鼠标经过时颜色
/// </summary>
[Category("扩展设置")]
[Description("鼠标经过时颜色")]
public string MouseOverColor

{
get

{
if (ViewState["MouseOverColor"] == null)

{
ViewState["MouseOverColor"] = "#9900FF";
}
return (string)ViewState["MouseOverColor"];
}

set

{
ViewState["MouseOverColor"] = value;
}
}


/**//// <summary>
/// 鼠标经过时颜色
/// </summary>
[Category("扩展设置")]
[Description("鼠标离开时颜色")]
public string MouseOutColor

{
get

{
if (ViewState["MouseOutColor"] == null)

{
ViewState["MouseOutColor"] = "#FFFFFF";
}
return (string)ViewState["MouseOutColor"];
}

set

{
ViewState["MouseOutColor"] = value;
}
}


/**//// <summary>
/// 是否启用 HighlightColor
/// </summary>
[Category("扩展设置")]
[Description("是否启用 MouseOverColor")]
public bool IsOpenMouseOverColor

{
get

{
if (ViewState["enableSelection"] == null)

{
ViewState["enableSelection"] = true;
}
return (bool)ViewState["enableSelection"];
}

set

{
ViewState["enableSelection"] = value;
}
}


/**//// <summary>
/// 是否启用扩展
/// </summary>
[Category("扩展设置")]
[Description("是否启用扩展")]
public bool ActivePagerBar

{
get

{
if (ViewState["enableLNSeaPager"] == null)

{
ViewState["enableLNSeaPager"] = true;
}
return (bool)ViewState["enableLNSeaPager"];
}

set

{
this.AllowPaging = value;
ViewState["enableLNSeaPager"] = value;
}
}


/**//// <summary>
/// 默认排序(图标显示升序降序时用)
/// </summary>
[Category("扩展设置")]
[Description("默认排序(图标显示升序降序时用)")]
public SortDirection SortDirectionAlt

{
get

{
if (ViewState["sortDirection"] == null)

{
ViewState["sortDirection"] = SortDirection.Ascending;
}
return (SortDirection)ViewState["sortDirection"];
}

set

{
ViewState["sortDirection"] = value;
}
}


/**//// <summary>
/// 默认排序字段(图标显示升序降序时用)
/// </summary>
[Category("扩展设置")]
[Description("默认排序字段(图标显示升序降序时用)")]
public string SortExpressionAlt

{
get

{
if (ViewState["sortExpressionAlt"] == null)

{
ViewState["sortExpressionAlt"] = "";
}
return (string)ViewState["sortExpressionAlt"];
}

set

{
ViewState["sortExpressionAlt"] = value;
}
}


/**//// <summary>
/// Get or Set Image location to be used to display Ascending Sort order.
/// </summary>
[
Description("降序时显示的图片URL"),
Category("扩展设置"),
Editor("System.Web.UI.Design.UrlEditor", typeof(System.Drawing.Design.UITypeEditor)),
DefaultValue(""),
]
public string SortDescImageUrl

{
get

{
object o = ViewState["SortImageDesc"];
return (o != null ? o.ToString() : "");
}
set

{
ViewState["SortImageDesc"] = value;
}
}


/**//// <summary>
/// Get or Set Image location to be used to display Ascending Sort order.
/// </summary>
[
Description("升序时显示的图片"),
Category("扩展设置"),
Editor("System.Web.UI.Design.UrlEditor", typeof(System.Drawing.Design.UITypeEditor)),
DefaultValue(""),
]
public string SortAscImageUrl

{
get

{
object o = ViewState["SortImageAsc"];
return (o != null ? o.ToString() : "");
}
set

{
ViewState["SortImageAsc"] = value;
}
}



/**//// <summary>
/// 是否显示升序降序图标
/// </summary>
[
Description("是否显示升序降序图片URL"),
Category("扩展设置"),
Editor("System.Web.UI.Design.UrlEditor", typeof(System.Drawing.Design.UITypeEditor)),
DefaultValue("false"),
]
public bool IsShowSortDirectionImg

{
get

{
object o = ViewState["ShowSortDirection"];
return (o != null ? Convert.ToBoolean(o) : false);
}
set

{
ViewState["ShowSortDirection"] = value;
}
}


/**//// <summary>
/// 分页信息当前第{0}页共{1}页{2}条记录
/// </summary>
[Category("扩展设置")]
[Description("分页信息当前第{0}页共{1}页{2}条记录")]
public string PageInfo

{
get

{
if (ViewState["PageInfo"] == null)

{
ViewState["PageInfo"] = "当前{0},共{1}页{2}条记录";
}
return (string)ViewState["PageInfo"];
}

set

{
ViewState["PageInfo"] = value;
}
}


/**//// <summary>
/// 当前页左右的数字个数(不包括智能的)
/// </summary>
[Category("扩展设置")]
[Description("当前页左右的数字个数(不包括智能的)")]
public int NumCount

{
get

{
if (ViewState["NumCount"] == null)

{
ViewState["NumCount"] = 5;
}
return (int)ViewState["NumCount"];
}

set

{
ViewState["NumCount"] = value;
}
}


/**//// <summary>
/// 是否显示智能数字
/// </summary>
[Category("扩展设置")]
[Description("是否显示智能数字")]
public bool IsShowSmartPage

{
get

{
if (ViewState["IsShowSmartPage"] == null)

{
ViewState["IsShowSmartPage"] = true;
}
return (bool)ViewState["IsShowSmartPage"];
}

set

{
ViewState["IsShowSmartPage"] = value;
}
}


/**//// <summary>
/// 分页信息显示在左边还是在右边
/// </summary>
[Category("扩展设置")]
[Description("是否启用 HighlightColor")]
public bool PageInfoShowLeft

{
get

{
if (ViewState["PageInfoShowLeft"] == null)

{
ViewState["PageInfoShowLeft"] = true;
}
return (bool)ViewState["PageInfoShowLeft"];
}

set

{
ViewState["PageInfoShowLeft"] = value;
}
}


/**//// <summary>
/// 智能导航数字的第一个的倍数
/// </summary>
[Category("扩展设置")]
[Description("智能导航数字的第一个的倍数")]
public int BeiShu

{
get

{
if (ViewState["BeiShu"] == null)

{
ViewState["BeiShu"] = 10;
}
return (int)ViewState["BeiShu"];
}

set

{
ViewState["BeiShu"] = value;
}
}


/**//// <summary>
/// 当前页的颜色
/// </summary>
[Category("扩展设置")]
[Description("当前页的颜色")]
public string CurrentPageColor

{
get

{
if (ViewState["CurrentPageColor"] == null)

{
ViewState["CurrentPageColor"] = "red";
}
return (string)ViewState["CurrentPageColor"];
}

set

{
ViewState["CurrentPageColor"] = value;
}
}


/**//// <summary>
/// 智能数字的颜色
/// </summary>
[Category("扩展设置")]
[Description("智能数字的颜色")]
public string SmartPageColor

{
get

{
if (ViewState["SmartPageColor"] == null)

{
ViewState["SmartPageColor"] = "#00FF00";
}
return (string)ViewState["SmartPageColor"];
}

set

{
ViewState["SmartPageColor"] = value;
}
}

#endregion


/**//// <summary>
/// When using GridView in certain ways the SortDirection and SortExpression
/// properties are sometimes left blank or never changed. When using this control,
/// the Alt properties remedy this situation.
/// </summary>
/// <param name="e"></param>
protected override void OnSorting(GridViewSortEventArgs e)

{
//Handle setting up of sorting info
if (!String.IsNullOrEmpty(this.SortExpression))

{
SortExpressionAlt = e.SortExpression;
SortDirectionAlt = e.SortDirection;
}
else

{
if (SortExpressionAlt == e.SortExpression)

{
if (SortDirectionAlt == SortDirection.Ascending)

{
SortDirectionAlt = SortDirection.Descending;
}
else

{
SortDirectionAlt = SortDirection.Ascending;
}
}
else

{
SortDirectionAlt = SortDirection.Ascending;
}

this.SortExpressionAlt = e.SortExpression;
}

base.OnSorting(e);
}




初始化在分页功能启用时显示的页导航行。#region 初始化在分页功能启用时显示的页导航行。

/**//// <summary>
/// 初始化在分页功能启用时显示的页导航行。
/// </summary>
/// <param name="row">一个 GridViewRow,表示要初始化的页导航行。 </param>
/// <param name="columnSpan">页导航行应跨越的列数</param>
/// <param name="pagedDataSource">一个 PagedDataSource,表示数据源。 </param>
protected override void InitializePager(GridViewRow row, int columnSpan, PagedDataSource pagedDataSource)

{
string pageinfo = this.PageInfo.Replace("{0}", (pagedDataSource.CurrentPageIndex + 1).ToString()).Replace
("{1}", pagedDataSource.PageCount.ToString()).Replace("{2}", pagedDataSource.DataSourceCount.ToString());

if (this.ActivePagerBar)

{
base.PagerTemplate = new ExtendGridViewPagerTemplate(
this.PageIndex,
this.PageCount,
this.PagerSettings.FirstPageText,
this.PagerSettings.PreviousPageText,
this.PagerSettings.NextPageText,
this.PagerSettings.LastPageText,
pageinfo,
this.NumCount,
this.IsShowSmartPage,
this.BeiShu,
this.PageInfoShowLeft,
this.SmartPageColor,
this.CurrentPageColor
);
}
base.InitializePager(row, columnSpan, pagedDataSource);
}
#endregion


/**//// <summary>
/// 呈现 GridView 控件之前,必须先为该控件中的每一行创建一个 GridViewRow 对象。
/// 在创建 GridView 控件中的每一行时,将引发 RowCreated 事件。这使您可以提供一个这样的事件处理方法,
/// 即每次发生此事件时都执行一个自定义例程(如在行中添加自定义内容)。
/// </summary>
/// <param name="e"></param>
protected override void OnRowCreated(GridViewRowEventArgs e)

{
//给继承者的说明 在派生类中重写 OnRowCreated 时,一定要调用基类的 OnRowCreated 方法,以便已注册的委托对事件进行接收。
base.OnRowCreated(e);
if (e.Row.RowType == DataControlRowType.DataRow)

{
//If row selection is enabled then add mouse over scripts to enable on client.
if (IsOpenMouseOverColor)

{
e.Row.Attributes.Add("onmouseover", "this.style.backgroundColor = '" + this.MouseOverColor + "';");
e.Row.Attributes.Add("onmouseout", "this.style.backgroundColor = '" + this.MouseOutColor + "';");
}
}
else if (e.Row.RowType == DataControlRowType.Header && IsShowSortDirectionImg)

{
foreach (TableCell headerCell in e.Row.Cells)

{
if (headerCell.HasControls())

{
AddSortImageToHeaderCell(headerCell);
}
}
}
}
//Header中加入排序的图片
private void AddSortImageToHeaderCell(TableCell headerCell)

{
// 查出headerCell中的 linkButton
LinkButton lnk = (LinkButton)headerCell.Controls[0];
if (lnk != null)

{
System.Web.UI.WebControls.Image img = new System.Web.UI.WebControls.Image();
// 设置图片的URL
img.ImageUrl = (this.SortDirectionAlt == SortDirection.Ascending ? this.SortAscImageUrl : this.SortDescImageUrl);
// 如果用户选择了排序,则加入排序图片
if (this.SortExpressionAlt == lnk.CommandArgument)

{
//加入一个空格
headerCell.Controls.Add(new LiteralControl(" "));
headerCell.Controls.Add(img);
}
}
}
}




/**//// <summary>
/// 分页导航条扩展
/// </summary>
class ExtendGridViewPagerTemplate : ITemplate

{

私有成员#region 私有成员
int _pageIndex;//当前页
int _pageNumber

{
get

{
return _pageIndex + 1;
}
set

{
_pageIndex = value - 1;
}
}//当前页 第一页为 1
int _pageCount;//总页数
string _First;
string _Prev;
string _Next;
string _Last;
string _PageInfo;
int _NumCount;
int _Beishu = 3;

int _StartPage;
int _EndPage;
int _MoreStartPage1;
int _MoreStartPage2;
int _MoreStartPage3;
int _MoreEndPage1;
int _MoreEndPage2;
int _MoreEndPage3;
bool _IsShowSmartPage = true;
bool _PageInfoShowLeft = true;
string _CurrentPageColor = "red";
string _SmartPageColor = "#00FF00";
#endregion


构造函数#region 构造函数

/**//// <summary>
/// 构造函数
/// </summary>
/// <param name="pageIndex">当前页(第一页为0)</param>
/// <param name="pageCount">总页数</param>
/// <param name="first">首页</param>
/// <param name="prev">上页</param>
/// <param name="next">下页</param>
/// <param name="last">尾页</param>
/// <param name="pageinfo">分页信息</param>
/// <param name="numcount">当前页数字左右显示的数字个数</param>
/// <param name="isShowSmartPage">是否显示智能导航数字</param>
/// <param name="beiShu">智能导航数字的第一个的倍数</param>
/// <param name="pageInfoShowLeft">是否左边显示分页信息</param>
/// <param name="smartPageColor">智能数字颜色</param>
/// <param name="currentPageColor">当前页颜色</param>
public ExtendGridViewPagerTemplate(
int pageIndex,
int pageCount,
string first,
string prev,
string next,
string last,
string pageinfo,
int numcount,
bool isShowSmartPage,
int beiShu,
bool pageInfoShowLeft,
string smartPageColor,
string currentPageColor
)

{
_pageIndex = pageIndex;
_pageCount = pageCount;
_First = first;
_Prev = prev;
_Next = next;
_Last = last;
_PageInfo = pageinfo;
_NumCount = numcount;
_IsShowSmartPage = isShowSmartPage;
_Beishu = beiShu;
_PageInfoShowLeft = pageInfoShowLeft;
_SmartPageColor = smartPageColor;
_CurrentPageColor = currentPageColor;
}
#endregion


/**//// <summary>
/// 对象初始化时调用
/// </summary>
/// <param name="container"></param>
public void InstantiateIn(Control container)

{
if (_PageInfoShowLeft)

{
createPageInfo(container);
createSpacer(container);
}

createFirstLinkButtion(container);//首页
createSpacer(container);//空格
createPrevLinkButton(container);//上页
createSpacer(container);
numericBar(container);//数字导航条
createSpacer(container);
createNextLinkButton(container);//下页
createSpacer(container);
createLastLinkButtion(container);//尾页

if (!_PageInfoShowLeft)

{
createPageInfo(container);
createSpacer(container);
}

}
//成生空格
private void createSpacer(Control container)

{
Literal spacer = new Literal();
spacer = new Literal();
spacer.Text = " ";
container.Controls.Add(spacer);
}
//生成首页按钮
private void createFirstLinkButtion(Control container)

{
if (_pageIndex > 0)

{
LinkButton pageButton;
pageButton = new LinkButton();
pageButton.Text = _First;
pageButton.CommandName = "Page";
pageButton.CommandArgument = "1";
container.Controls.Add(pageButton);
}
else

{
Literal temp = new Literal();
temp.Text = "<span disabled=true>" + _First + "</span>";
container.Controls.Add(temp);
}
}
//生成上一页按钮
private void createPrevLinkButton(Control container)

{
if (_pageIndex > 0)

{
LinkButton prevButton = new LinkButton();
prevButton.CommandName = "Page";
prevButton.CommandArgument = "Prev";
prevButton.Text = _Prev;
container.Controls.Add(prevButton);
}
else

{
Literal temp = new Literal();
temp.Text = "<span disabled=true>" + _Prev + "</span>";
container.Controls.Add(temp);
}
}
//生成下一页按钮
private void createNextLinkButton(Control container)

{
if (_pageIndex < _pageCount - 1 && _pageIndex >= 0)

{
LinkButton prevButton = new LinkButton();
prevButton.CommandName = "Page";
prevButton.CommandArgument = "Next";
prevButton.Text = _Next;
container.Controls.Add(prevButton);
}
else

{
Literal temp = new Literal();
temp.Text = "<span disabled=true>" + _Next + "</span>";
container.Controls.Add(temp);
}
}
//生成尾按钮
private void createLastLinkButtion(Control container)

{
if (_pageIndex < _pageCount - 1 && _pageIndex >= 0)

{
LinkButton pageButton;
pageButton = new LinkButton();
pageButton.Text = _Last;
pageButton.CommandName = "Page";
pageButton.CommandArgument = _pageCount.ToString();
container.Controls.Add(pageButton);
}
else

{
Literal temp = new Literal();
temp.Text = "<span disabled=true>" + _Last + "</span>";
container.Controls.Add(temp);
}

}
//生成数字按钮
private void createNumericPageButton(Control container, int pageIndex, bool isSmatrPage)

{

string _text = (pageIndex + 1).ToString();
if (isSmatrPage)

{
_text = "<font color=\"" + this._SmartPageColor + "\">" + _text + "</font>";
}
if (pageIndex == _pageIndex)

{
_text = "<font color=\"" + this._CurrentPageColor + "\"><b>" + _text + "</b></font>";
}
LinkButton pageButton;
pageButton = new LinkButton();
pageButton.Text = _text;
pageButton.CommandName = "Page";
pageButton.CommandArgument = (pageIndex + 1).ToString();
container.Controls.Add(pageButton);
}

private void numericBar(Control container)

{


跟据 _NumCount 计算出导航条的 起始位置与结束位置#region 跟据 _NumCount 计算出导航条的 起始位置与结束位置
//如果总页数 小于或等于 导航条的数字个数,则把所有页码显示出来
if (_pageCount <= _NumCount * 2 + 1)

{
_StartPage = 1;
_EndPage = _pageCount;
}
else

{
if (_pageNumber <= _NumCount + 1)

{
_StartPage = 1;
_EndPage = _NumCount * 2 + 1;
}
else

{
if (_pageCount <= _pageNumber + _NumCount)

{
//显示最后 x*2+1页
_StartPage = _pageCount - _NumCount * 2;
_EndPage = _pageCount;
}
else

{
//正常显示
_StartPage = _pageNumber - _NumCount;
_EndPage = _pageNumber + _NumCount;
}
}
}
#endregion


输出前N页#region 输出前N页
if (_IsShowSmartPage)

{

_MoreStartPage1 = _StartPage - _Beishu - 1;
_MoreStartPage2 = _StartPage - _Beishu * 10 - _Beishu - 1;
_MoreStartPage3 = _StartPage - _Beishu * 100 - _Beishu * 10 - _Beishu - 1;
if (_MoreStartPage1 > 0)

{
if (_MoreStartPage3 > 0)

{
createNumericPageButton(container, _MoreStartPage3, true);
createSpacer(container);
}
if (_MoreStartPage2 > _NumCount * 4)

{
createNumericPageButton(container, _MoreStartPage2, true);
createSpacer(container);
}
else

{
if (_MoreStartPage2 > 0)

{
createNumericPageButton(container, _MoreStartPage2, true);
createSpacer(container);
}
}
createNumericPageButton(container, _MoreStartPage1, true);
createSpacer(container);
}

}
#endregion


输出正常数字#region 输出正常数字
for (int i = _StartPage - 1; i < _EndPage; i++)

{
createNumericPageButton(container, i, false);
createSpacer(container);
}
#endregion


输出后N页#region 输出后N页
_MoreEndPage1 = _EndPage + _Beishu - 1;
_MoreEndPage2 = _EndPage + _Beishu * 10 + _Beishu - 1;
_MoreEndPage3 = _EndPage + _Beishu * 100 + _Beishu * 10 + _Beishu - 1;
if (_MoreEndPage1 < _pageCount)

{
createNumericPageButton(container, _MoreEndPage1, true);
createSpacer(container);
if (_MoreEndPage2 < _pageCount - _NumCount * 4)

{
createNumericPageButton(container, _MoreEndPage2, true);
createSpacer(container);
if (_MoreEndPage3 < _pageCount)

{
createNumericPageButton(container, _MoreEndPage3, true);
createSpacer(container);
}
}
else

{
if (_MoreEndPage2 < _pageCount)

{
createNumericPageButton(container, _MoreEndPage1, true);
createSpacer(container);
}
}

}
#endregion
}

private void createPageInfo(Control container)

{
Literal pageinfo = new Literal();
pageinfo = new Literal();
pageinfo.Text = _PageInfo;
container.Controls.Add(pageinfo);
}
}

}

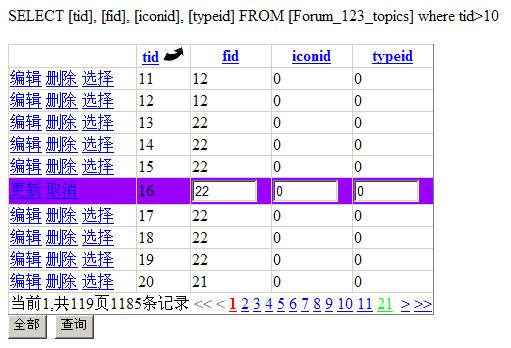
public partial class _Default : System.Web.UI.Page


{
protected void Page_Load(object sender, EventArgs e)

{
if (ViewState["SelectCmd"] != null)

{
SqlDataSource1.SelectCommand = (string)ViewState["SelectCmd"];
MyGridView1.DataBind();
}
}

protected void Button1_Click(object sender, EventArgs e)

{
ViewState["SelectCmd"] = "SELECT [tid], [fid], [iconid], [typeid] FROM [Forum_123_topics] where tid>10";
SqlDataSource1.SelectCommand = (string)ViewState["SelectCmd"];
MyGridView1.DataBind();
Response.Write(SqlDataSource1.SelectCommand);
}
protected void Button2_Click(object sender, EventArgs e)

{
ViewState["SelectCmd"] = "SELECT [tid], [fid], [iconid], [typeid] FROM [Forum_123_topics]";
SqlDataSource1.SelectCommand = (string)ViewState["SelectCmd"];
MyGridView1.DataBind();
Response.Write(SqlDataSource1.SelectCommand);
}
}
转载于:https://www.cnblogs.com/fanfajin/archive/2007/08/21/864566.html