1. 作用
- 异步操作
- 获取执行结果
- 取消任务执行,判断是否取消执行
- 判断任务执行是否完毕
2. demo
public static void main(String[] args) throws Exception {Callable<String> callable= () -> search();FutureTask<String> futureTask=new FutureTask<>(callable);futureTask.run();// 持续阻塞String s = futureTask.get();System.out.println(s);System.out.println("主线程收集了1颗龙珠");}/*** 收集龙珠*/public static String search() {try {TimeUnit.SECONDS.sleep(2);} catch (InterruptedException e) {throw new RuntimeException(e);}return "找到了1颗龙珠";}
3. 原理
3.1 类关系图
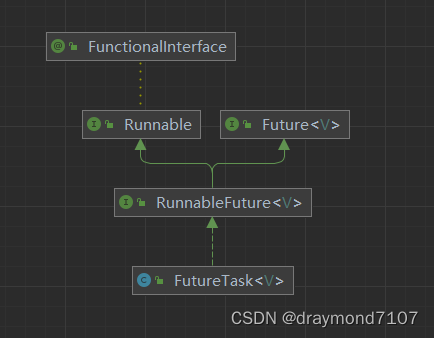
3.2 主要流程
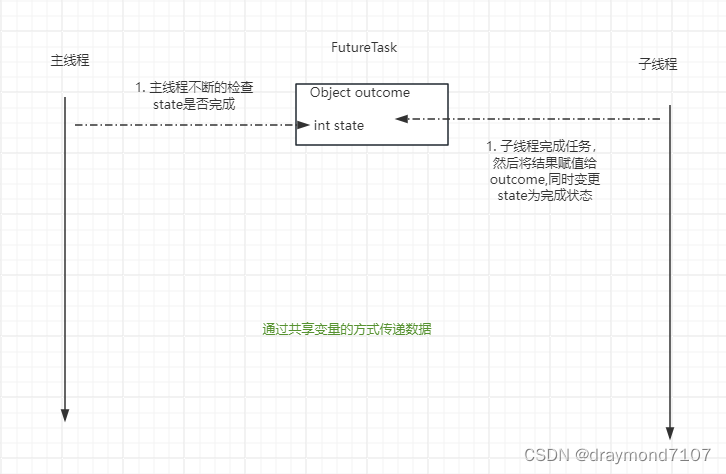
3.3 方法
3.3.1 futureTask.run()
public void run() {if (state != NEW ||!UNSAFE.compareAndSwapObject(this, runnerOffset,null, Thread.currentThread()))return;try {Callable<V> c = callable;if (c != null && state == NEW) {V result;boolean ran;try {// 执行任务result = c.call();ran = true;} catch (Throwable ex) {result = null;ran = false;setException(ex);}if (ran)// 将结果赋值给outcomeset(result);}} finally {runner = null;int s = state;if (s >= INTERRUPTING)handlePossibleCancellationInterrupt(s);}}protected void set(V v) {if (UNSAFE.compareAndSwapInt(this, stateOffset, NEW, COMPLETING)) {outcome = v;UNSAFE.putOrderedInt(this, stateOffset, NORMAL); // final statefinishCompletion();}}
3.3.2 futureTask.get()
public V get() throws InterruptedException, ExecutionException {// 当前状态未完成时,进入自旋等待int s = state;if (s <= COMPLETING)s = awaitDone(false, 0L);// 返回子进程的执行结果return report(s);}private int awaitDone(boolean timed, long nanos)throws InterruptedException {final long deadline = timed ? System.nanoTime() + nanos : 0L;WaitNode q = null;boolean queued = false;// 尝试自旋等待结果for (;;) {if (Thread.interrupted()) {removeWaiter(q);throw new InterruptedException();}int s = state;if (s > COMPLETING) {if (q != null)q.thread = null;return s;}else if (s == COMPLETING) // cannot time out yetThread.yield();else if (q == null)q = new WaitNode();else if (!queued)queued = UNSAFE.compareAndSwapObject(this, waitersOffset,q.next = waiters, q);else if (timed) {nanos = deadline - System.nanoTime();if (nanos <= 0L) {removeWaiter(q);return state;}// 多次等待后park一会(自己会醒)LockSupport.parkNanos(this, nanos);}else// 多次等待后park一会(自己不会醒)LockSupport.park(this);}}// 获取结果private V report(int s) throws ExecutionException {Object x = outcome;if (s == NORMAL)return (V)x;if (s >= CANCELLED)throw new CancellationException();throw new ExecutionException((Throwable)x);}