Java JDBC
文章目录
- jdbc概述
- 基本介绍
- jdbc原理示意图
- jdbc快速入门
- JDBC程序编写步骤
- 获取数据库连接5种方式
- ResultSet[结果集]
- SQL注入
- Statement
- PreparedStatement
- 预处理好处
- 基本使用
- JDBC API
- JDBCUtils
- 工具类
- 使用工具类
- 事务
- 基本介绍
- 应用实例
- 模拟经典的转帐业务 - 未使用事务
- 模拟经典的转帐业务 - 使用事务
- 批处理
- 基本介绍
- 传统方式
- 批处理方式
- 源码
- 连接池
- 传统获取Connection问题分析
- 数据库连接池基本介绍
- 数据库连接池种类
- DBCP
- C3P0
- Proxool
- BoneCP
- Druid
- Druid工具类
- 使用工具类
- APache-DBUtils
- resultSet问题
- 原生解决方案
- 使用APache-DBUtils
- 基本介绍
- 使用DBUtils+数据连接池(德鲁伊)方式,完成对表的crud
- apache-dbutils+druid完成返回的结果是单行记录(单个对象)的处理
- 演示apache-dbutils+druid完成查询结果是单行单列-返回的就是object
- 演示apache-dbutils+druid完成dml(update,insert,delete)
- 表和JavaBean的类型映射关系
- DAO增删改查-BasicDao
- 问题
- 基本说明
- BasicDAO应用实例
- dao包
jdbc概述
基本介绍
1.JDBC为访问不同的数据库提供了统一的接口,为使用者屏蔽了细节问题。
2.Java程序员使用JDBC,可以连接任何提供了JDBC驱动程序的数据库系统,从而完成对数据库的各种操作。
jdbc原理示意图
jdbc快速入门
JDBC程序编写步骤
1.注册驱动 - 加载Driver类
2.获取连接 - 得到Connection
3.执行增删改查 - 发送SQL给mysql执行
4.释放资源 - 关闭相关连接
import com.mysql.jdbc.Driver;import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;public class Jdbc01 {public static void main(String[] args) throws SQLException {// 注册驱动 - 加载Driver类Driver driver = new Driver();String url = "jdbc:mysql://localhost:3306/hsp_db02";Properties properties = new Properties();properties.setProperty("user", "root");properties.setProperty("password", "hsp");String sql = "insert into actor values(null,'刘德华','男','1970-11-11', '110')";// 获取连接 - 得到ConnectionConnection connection = driver.connect(url, properties);// statement 用于执行静态SQL语句并返回其生成的结果的对象Statement statement = connection.createStatement();int rows = statement.executeUpdate(sql);System.out.println(rows > 0 ? "成功" : "失败");// 关闭连接资源statement.close();connection.close();}
}
获取数据库连接5种方式
方式1 com.hspedu.jdbc.conn
会直接使用com.mysql.jdbc.Driver(),属于静态加载,灵活性差,依赖强
public void connect01() throws SQLException {Driver driver = new Driver();String url = "jdbc:mysql://localhost:3306/hsp_db02";Properties properties = new Properties();properties.setProperty("user", "root");properties.setProperty("password", "hsp");String sql = "insert into actor values(null,'刘德华','男','1970-11-11', '110')";Connection connection = driver.connect(url, properties);Statement statement = connection.createStatement();int rows = statement.executeUpdate(sql);System.out.println(rows > 0 ? "成功" : "失败");statement.close();connection.close();
}
方式2
便用反射加载Driver类,动态加载,更加时灵活,减少依颗性
@Test
public void connect02() throws ClassNotFoundException, InstantiationException, IllegalAccessException, SQLException {Class<?> aClass = Class.forName("com.mysql.jdbc.Driver");Driver driver = (Driver)aClass.newInstance();String url = "jdbc:mysql://localhost:3306/hsp_db02";Properties properties = new Properties();properties.setProperty("user", "root");properties.setProperty("password", "hsp");Connection connection = driver.connect(url, properties);System.out.println(connection);
}
方式3
使用DriverManager 替代 Diver 进行统一管理
@Test
public void connect03() throws ClassNotFoundException, InstantiationException, IllegalAccessException, SQLException {Class<?> aClass = Class.forName("com.mysql.jdbc.Driver");Driver driver = (Driver)aClass.newInstance();String url = "jdbc:mysql://localhost:3306/hsp_db02";Properties properties = new Properties();properties.setProperty("user", "root");properties.setProperty("password", "hsp");DriverManager.registerDriver(driver);Connection connection = DriverManager.getConnection(url, properties);System.out.println(connection);
}
方式4
使用Class.forName自动完成注册驱动,简化代码
@Test
public void connect04() throws ClassNotFoundException, SQLException {Class.forName("com.mysql.jdbc.Driver");String url = "jdbc:mysql://localhost:3306/hsp_db02";Properties properties = new Properties();properties.setProperty("user", "root");properties.setProperty("password", "hsp");Connection connection = DriverManager.getConnection(url, properties);System.out.println(connection);
}
方式5
方式5,在方式4的基础上改进,增加配置文件,让连接mysql更灵活
@Test
public void connect05() throws ClassNotFoundException, InstantiationException, IllegalAccessException, SQLException, IOException {Properties properties = new Properties();properties.load(new FileInputStream("src\\mysql.properties"));Class.forName(properties.getProperty("driver"));Connection connection = DriverManager.getConnection(properties.getProperty("url"),properties.getProperty("user"),properties.getProperty("password"));System.out.println(connection);
}
ResultSet[结果集]
1.表示数据库结果集的数据表,通常通过执行查询数据库的语句生成
2.ResultSet对象保持一个光标指向其当前的数据行。最初,光标位于第一行之前
3.next方法将光标移动到下一行,并且由于在ResultSeti对象中没有更多行时返回
false,因此可以在while循环中使用循环来遍历结果集
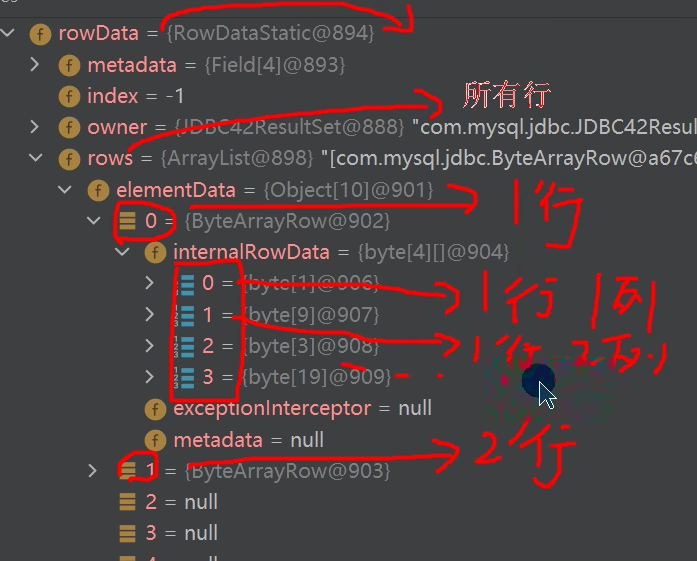
SQL注入
Statement
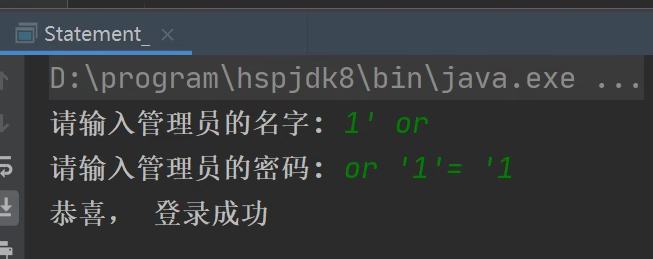
PreparedStatement
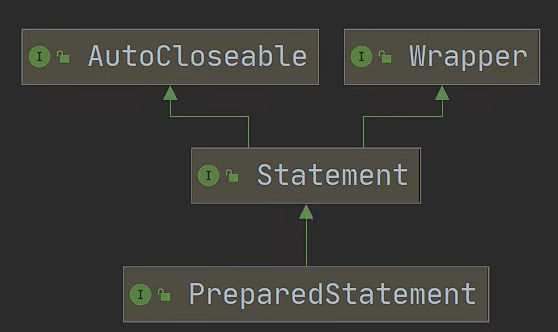
预处理好处
1.不再使用+拼接sql语句,减少语法错误
2.有效的解决了sql注入问题!
3.大大减少了编译次数,效率较高
基本使用
JDBC API
JDBCUtils
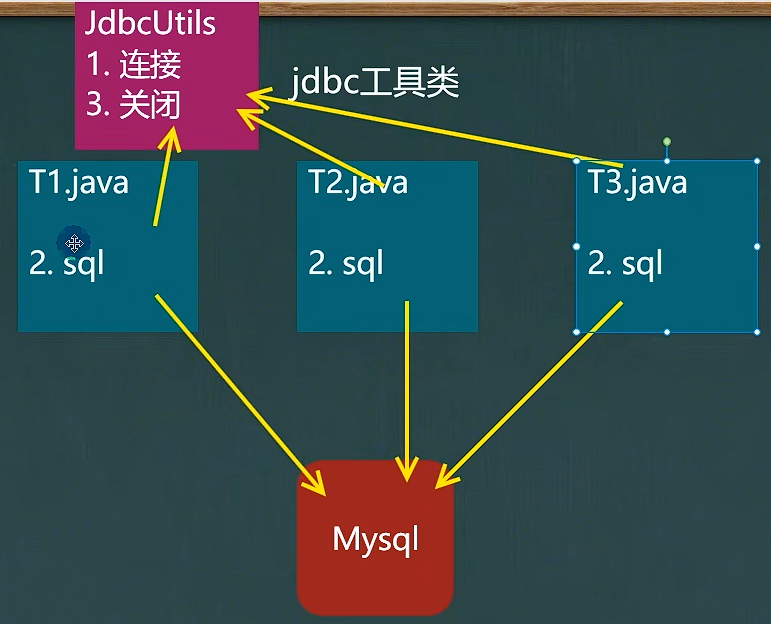
工具类
package com.hspedu.jdbc.utils;import java.io.FileInputStream;
import java.io.IOException;
import java.sql.*;
import java.util.Properties;/*** @program: chapter25* @since: jdk1.8* @description: 这是一个工具类,完成mysql的连接和关闭资源* @author: Administrator* @create: 2024-12-13 16:54**/
public class JDBCUtils {//定义相关属性(4个),因为只需要一份,因此,我们做出staticprivate static String user;private static String password;private static String url;private static String driver;// 在静态代码块去初始化static {Properties properties = new Properties();try {properties.load(new FileInputStream("src\\mysql.properties"));user = properties.getProperty("user");password = properties.getProperty("password");url = properties.getProperty("url");driver = properties.getProperty("driver");} catch (IOException e) {//在实际开发中,我们可以这样处理//1.将编译异常转成运行异常//2.这时调用者,可以选择捕获该异常,也可以选择默认处理该异常,比较方便。throw new RuntimeException(e);}}/*** 获取数据库连接** @return 返回数据库连接对象* @throws RuntimeException 如果发生SQL异常,则抛出运行时异常*/public static Connection getConnection() {try {return DriverManager.getConnection(url, user, password);} catch (SQLException e) {throw new RuntimeException(e);}}/*** 关闭数据库资源** @param rs ResultSet对象,可能为null* @param stmt Statement对象,可能为null* @param conn Connection对象,可能为null* @throws RuntimeException 如果在关闭资源时发生SQL异常,则抛出运行时异常*/public static void close(ResultSet rs, Statement stmt, Connection conn) {try {if (rs != null) {rs.close();}if (stmt != null) {stmt.close();}if (conn != null) {conn.close();}} catch (SQLException e) {throw new RuntimeException(e);}}
}
使用工具类
package com.hspedu.jdbc.utils;import org.junit.jupiter.api.Test;import java.sql.*;/*** @program: chapter25* @since: jdk1.8* @description: 该类演示如何使用JDBCUtils工具类,完成dml和select* @author: Administrator* @create: 2024-12-13 18:00**/
public class JDBCUtils_Use {@Testpublic void testSelect() {Connection connection = null;String sql = "select * from actor where id = ?";PreparedStatement preparedStatement = null;ResultSet resultSet = null;try {connection = JDBCUtils.getConnection();preparedStatement = connection.prepareStatement(sql);preparedStatement.setInt(1, 1);resultSet = preparedStatement.executeQuery();while (resultSet.next()) {int id = resultSet.getInt("id");String name = resultSet.getString("name");String sex = resultSet.getString("sex");Date borndate = resultSet.getDate("borndate");String phone = resultSet.getString("phone");System.out.println(id + "\t" + name + "\t" + sex + "\t" + borndate + "\t" + phone);}} catch (SQLException e) {throw new RuntimeException(e);} finally {// 关闭资源JDBCUtils.close(resultSet, preparedStatement, connection);}}@Testpublic void testDML() {Connection connection = null;String sql = "update actor set name = ? where id = ?";PreparedStatement preparedStatement = null;try {connection = JDBCUtils.getConnection();preparedStatement = connection.prepareStatement(sql);preparedStatement.setString(1, "周星驰");preparedStatement.setInt(2, 1);int i = preparedStatement.executeUpdate();System.out.println(i > 0 ? "成功" : "失败");} catch (SQLException e) {throw new RuntimeException(e);} finally {// 关闭资源JDBCUtils.close(null, preparedStatement, connection);}}
}
事务
基本介绍
应用实例
模拟经典的转帐业务 - 未使用事务

public class Transaction_ {@Testpublic void noTransaction() {Connection connection = null;String sql = "update account set balance = balance - 100 where id = 1";String sql2 = "update account set balance = balance + 100 where id = 2";PreparedStatement preparedStatement = null;try {connection = JDBCUtils.getConnection();preparedStatement = connection.prepareStatement(sql);preparedStatement.executeUpdate();int i = 1 / 0;preparedStatement = connection.prepareStatement(sql2);preparedStatement.executeUpdate();} catch (SQLException e) {throw new RuntimeException(e);} finally {// 关闭资源JDBCUtils.close(null, preparedStatement, connection);}}
}
马云的余额减少了马化腾的余额缺没有增加,银行很开心_
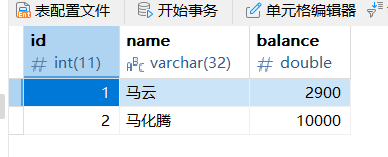
模拟经典的转帐业务 - 使用事务
public void useTransaction() {Connection connection = null;String sql = "update account set balance = balance - 100 where id = 1";String sql2 = "update account set balance = balance + 100 where id = 2";PreparedStatement preparedStatement = null;try {// 默认情况下,connection是默认自动提交connection = JDBCUtils.getConnection();// 将 connection 设置为不自动提交connection.setAutoCommit(false); // 相当于开启了事务preparedStatement = connection.prepareStatement(sql);preparedStatement.executeUpdate();int i = 1 / 0; // 抛出异常preparedStatement = connection.prepareStatement(sql2);preparedStatement.executeUpdate();// 提交事务connection.commit();} catch (Exception e) {System.out.println("执行发生了异常,撤销执行的sql");try {// 回滚到事务开启的地方if (connection != null) {connection.rollback();}} catch (SQLException ex) {ex.printStackTrace();}e.printStackTrace();} finally {// 关闭资源JDBCUtils.close(null, preparedStatement, connection);}
}
批处理
基本介绍
传统方式
public void noBatch() throws SQLException {Connection connection = JDBCUtils.getConnection();String sql = "insert into admin2 values(null, ?, ?)";PreparedStatement preparedStatement = connection.prepareStatement(sql);System.out.println("开始执行");long start = System.currentTimeMillis();for (int i = 0; i < 5000; i++) {preparedStatement.setString(1, "jack" + i);preparedStatement.setString(2, "666");preparedStatement.executeUpdate();}System.out.println("结束执行");long end = System.currentTimeMillis();System.out.println("传统的方式 耗时:" + (end - start) + "ms");JDBCUtils.close(null, preparedStatement, connection);
}
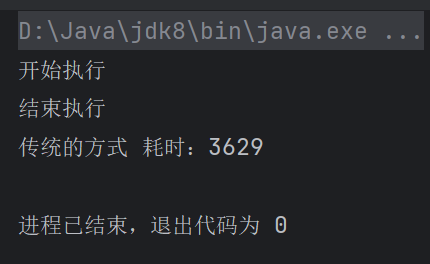
批处理方式
public void batch() throws SQLException {Connection connection = JDBCUtils.getConnection();String sql = "insert into admin2 values(null, ?, ?)";PreparedStatement preparedStatement = connection.prepareStatement(sql);System.out.println("开始执行");long start = System.currentTimeMillis();for (int i = 0; i < 5000; i++) {preparedStatement.setString(1, "jack" + i);preparedStatement.setString(2, "666");preparedStatement.addBatch();// 当有1000条记录时,在批量执行if ((i + 1) % 1000 == 0) {preparedStatement.executeBatch();preparedStatement.clearBatch();}}System.out.println("结束执行");long end = System.currentTimeMillis();System.out.println("批量的方式 耗时:" + (end - start) + "ms");JDBCUtils.close(null, preparedStatement, connection);
}
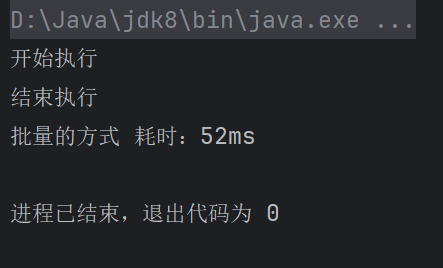
源码
连接池
传统获取Connection问题分析
数据库连接池基本介绍
连接放回去并不是关闭连接,而是不在引用这个连接(引用断开了,但连接对象还在)
数据库连接池种类
JDBC的数据库连接池使用javax.sql.DataSource来表示,DataSource只是一个接口,该接口通常由第三方提供实现
DBCP
DBCP数据库连接池,速度相对C3P0较快,但不稳定
C3P0
C3P0数据库连接池,速度相对较慢,稳定性不错(hibernate,spring)
方式1:相关参数,在程序中指定user,url,password等
// 创建一个数据源对象
ComboPooledDataSource comboPooledDataSource = new ComboPooledDataSource();
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String url = properties.getProperty("url");
String driver = properties.getProperty("driver");// 给数据源设置相关的参数
// 注意,连接管理是由 comboPooledDataSource 来管理
comboPooledDataSource.setDriverClass(driver);
comboPooledDataSource.setJdbcUrl(url);
comboPooledDataSource.setUser(user);
comboPooledDataSource.setPassword(password);
// 设置初始化连接数
comboPooledDataSource.setInitialPoolSize(10);
// 最大连接数
comboPooledDataSource.setMaxPoolSize(50);
long start = System.currentTimeMillis();
for (int i = 0; i < 5000; i++) {Connection connection = comboPooledDataSource.getConnection();// System.out.println("连接成功");connection.close();
}
long end = System.currentTimeMillis();
System.out.println("c3p0 5000次耗时" + (end - start) + "ms"); // c3p0 5000次耗时286ms
方式2:使用配置文件模版来完成
1.将c3p0提供的c3p0.config.xml拷贝到src目录下
2.该文件指定了连接数据库和连接池的相关参数
c3p0.config.xml
<?xml version="1.0" encoding="UTF-8"?>
<c3p0-config><default-config><!-- 这些是默认的连接池参数,如果没有在特定数据源中定义,则使用这些参数 --><property name="initialPoolSize">5</property><property name="minPoolSize">5</property><property name="maxPoolSize">20</property><property name="maxIdleTime">300</property> <!-- seconds --><property name="acquireIncrement">1</property><property name="maxStatements">50</property></default-config><!-- 数据源名称代表连接池 --><named-config name="myDataSource"><!-- 特定于 myDataSource 的连接池参数 --><property name="driverClass">com.mysql.jdbc.Driver</property><property name="jdbcUrl">jdbc:mysql://localhost:3306/hsp_db02</property><property name="user">root</property><property name="password">hsp</property><!-- 初始的连接数 --><property name="initialPoolSize">10</property><property name="minPoolSize">5</property><property name="maxPoolSize">50</property><!-- 每次增长的连接数 --><property name="acquireIncrement">5</property><!-- 可连接的最多的命令对象数 --><property name="maxStatements">5</property><!-- 其他可选配置 --><!-- 每个连接对象可连接的最多的命令对象数 --><property name="maxStatementsPerConnection">2</property></named-config>
</c3p0-config>
ComboPooledDataSource comboPooledDataSource = new ComboPooledDataSource("myDataSource");
long start = System.currentTimeMillis();
for (int i = 0; i < 5000; i++) {Connection connection = comboPooledDataSource.getConnection();// System.out.println("连接成功");connection.close();
}
long end = System.currentTimeMillis();
System.out.println("c3p0使用配置文件 连接/断开5000次 耗时:" + (end - start) + "ms"); // c3p0使用配置文件 连接/断开5000次 耗时:285ms
Proxool
Proxool数据库连接池,有监控连接池状态的功能,稳定性较C3P0差一点
BoneCP
BoneCP数据库连接池,速度快
Druid
Druid(德鲁伊)是阿里提供的数据库连接池,集DBCP、C3P0、Proxool优点于一身的数据库连接池
jar包下载地址:https://repo1.maven.org/maven2/com/alibaba/druid/1.1.10/
src/druid.properties
com.mysql.jdbc.Driver# Druid 配置文件模板 (druid.properties)
# 数据源配置
url=jdbc:mysql://localhost:3306/hsp_db02?rewriteBatchedStatements=true
username=root
password=hsp
driverClassName=com.mysql.jdbc.Driver
# 连接池参数配置
# 初始化大小
initialSize=10
# 最小空闲连接数
minIdle=5
# 最大连接数
maxActive=50
# 获取连接最大等待时间(毫秒)
maxWait=5000
Properties properties = new Properties();
properties.load(new FileInputStream("src\\druid.properties"));
// 创建一个指定参数的数据库连接池
DataSource dataSource = DruidDataSourceFactory.createDataSource(properties);
long start = System.currentTimeMillis();
for (int i = 0; i < 5000; i++) {Connection connection = dataSource.getConnection();// System.out.println(connection);connection.close();
}
long end = System.currentTimeMillis();
System.out.println(end - start); // druid连接池 操作500000次 耗时330ms
Druid工具类
package com.hspedu.jdbc.datasource;import com.alibaba.druid.pool.DruidDataSourceFactory;import javax.sql.DataSource;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;/*** @program: chapter25* @since: jdk1.8* @description: 基于druid数据库连接池的工具类* @author: Administrator* @create: 2024-12-14 17:17**/
public class JDBCUtilsByDruid {private static DataSource ds;static {Properties properties = new Properties();try {properties.load(new FileInputStream("src\\druid.properties"));ds = DruidDataSourceFactory.createDataSource(properties);} catch (Exception e) {throw new RuntimeException(e);}}/*** 获取数据库连接** @return 返回数据库连接对象* @throws SQLException 如果获取连接时发生SQL异常,则抛出此异常*/// 编写getConnection方法public static Connection getConnection() throws SQLException {return ds.getConnection();}/*** 关闭数据库连接(把使用的Connection对象放回连接池)、声明和结果集** @param rs 数据库结果集对象* @param stmt 数据库声明对象* @param conn 数据库连接对象* @throws RuntimeException 当关闭过程中发生SQL异常时抛出*/public static void close(ResultSet rs, Statement stmt, Connection conn) {try {if (rs != null) {rs.close();}if (stmt != null) {stmt.close();}if (conn != null) {conn.close(); // 对于Druid连接池,这里的close()实际上是归还连接到连接池}} catch (SQLException e) {throw new RuntimeException("关闭数据库资源时发生异常: " + e.getMessage(), e);}}}
使用工具类
@Test
public void testSelect() {Connection connection = null;String sql = "select * from actor where id = ?";PreparedStatement preparedStatement = null;ResultSet resultSet = null;try {connection = JDBCUtilsByDruid.getConnection();preparedStatement = connection.prepareStatement(sql);preparedStatement.setInt(1, 1);resultSet = preparedStatement.executeQuery();while (resultSet.next()) {int id = resultSet.getInt("id");String name = resultSet.getString("name");String sex = resultSet.getString("sex");Date borndate = resultSet.getDate("borndate");String phone = resultSet.getString("phone");System.out.println(id + "\t" + name + "\t" + sex + "\t" + borndate + "\t" + phone);}} catch (SQLException e) {throw new RuntimeException(e);} finally {// 关闭资源JDBCUtilsByDruid.close(resultSet, preparedStatement, connection);}
}
APache-DBUtils
resultSet问题
1.关闭connection后,resultSet结果集无法使用
2.resultSet不利于数据的管理
3.示意图
原生解决方案
Javabean, POJO, Domain对象
package com.hspedu.jdbc.datasource;import java.util.Date;/*** @program: chapter25* @since: jdk1.8* @description: Actor对象和actor表的记录对应* @author: Administrator* @create: 2024-12-14 19:21**/
public class Actor {private Integer id;private String name;private String sex;private Date borndate;private String phone;public Actor() {}public Actor(Integer id, String name, String sex, Date borndate, String phone) {this.id = id;this.name = name;this.sex = sex;this.borndate = borndate;this.phone = phone;}public Integer getId() {return id;}public void setId(Integer id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public String getSex() {return sex;}public void setSex(String sex) {this.sex = sex;}public Date getBorndate() {return borndate;}public void setBorndate(Date borndate) {this.borndate = borndate;}public String getPhone() {return phone;}public void setPhone(String phone) {this.phone = phone;}@Overridepublic String toString() {return "Actor{" +"id=" + id +", name='" + name + '\'' +", sex='" + sex + '\'' +", borndate=" + borndate +", phone='" + phone + '\'' +'}';}
}
把得到的resultset的记录,封装到Actor对象,放入到list集合
public ArrayList<Actor> testSelectToArrayList() {Connection connection = null;String sql = "select * from actor where id >= ?";PreparedStatement preparedStatement = null;ResultSet resultSet = null;ArrayList<Actor> list = new ArrayList<>();try {connection = JDBCUtilsByDruid.getConnection();preparedStatement = connection.prepareStatement(sql);preparedStatement.setInt(1, 1);resultSet = preparedStatement.executeQuery();while (resultSet.next()) {int id = resultSet.getInt("id");String name = resultSet.getString("name");String sex = resultSet.getString("sex");Date borndate = resultSet.getDate("borndate");String phone = resultSet.getString("phone");// 把得到的resultset的记录,封装到Actor对象,放入到list集合list.add(new Actor(id, name, sex, borndate, phone));}list.forEach(System.out::println);} catch (SQLException e) {throw new RuntimeException(e);} finally {// 关闭资源JDBCUtilsByDruid.close(resultSet, preparedStatement, connection);}return list;
}
使用APache-DBUtils
基本介绍
1.commons-dbutils是Apache组织提供的一个开源JDBC工具类库,它是对JDBC的封装,使用dbutils能极大简化jdbc编码的工作量[真的]。
DbUtils:类
1.QueryRunner类:该类封装了SQL的执行,是线程安全的。可以实现增、删、改、查、批处理
2.使用QueryRunner类实现查询
3.ResultSetHandler接口:该接口用于处理java.sql.ResultSet,将数据按要求转换为另一种形
式,
使用DBUtils+数据连接池(德鲁伊)方式,完成对表的crud
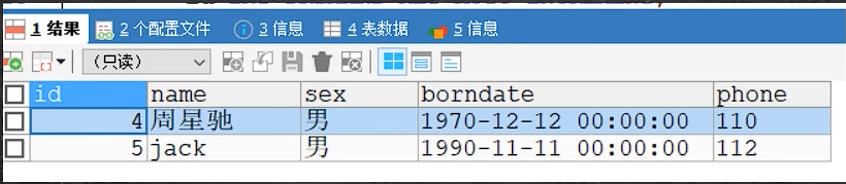
public void testQueryMany() throws SQLException {Connection connection = JDBCUtilsByDruid.getConnection();QueryRunner queryRunner = new QueryRunner();String sql = "select * from actor where id >= ?";// query方法就是执行sqL语句,得到resultset--封装到-->ArrayList集合中// new BeanListHandler<>(Actor.class):在将resultset->Actor对象->封装到ArrayList// 底层使用反射机制去获取Actor类的属性,然后进行封装// 1是给sql语句中的?赋值的// 底层得到的resultset,会在query关闭,还会关闭PreparedStatmentList<Actor> list = queryRunner.query(connection,sql,new BeanListHandler<Actor>(Actor.class),1);for (Actor actor : list) {System.out.println(actor);}JDBCUtilsByDruid.close(null,null, connection);
}
apache-dbutils+druid完成返回的结果是单行记录(单个对象)的处理
public void testQuerySingle() throws SQLException {Connection connection = JDBCUtilsByDruid.getConnection();QueryRunner queryRunner = new QueryRunner();String sql = "select * from actor where id = ?";// 因为我们返回的单行记录<--->单个对象,使用的Hander是BeanHandlerActor actor = queryRunner.query(connection, sql, new BeanHandler<>(Actor.class), 1);System.out.println(actor);JDBCUtilsByDruid.close(null, null, connection);
}
演示apache-dbutils+druid完成查询结果是单行单列-返回的就是object
public void testScalar() throws SQLException {Connection connection = JDBCUtilsByDruid.getConnection();QueryRunner queryRunner = new QueryRunner();String sql = "select name from actor where id = ?";Object object = queryRunner.query(connection, sql, new ScalarHandler<>(), 1);System.out.println(object);JDBCUtilsByDruid.close(null, null, connection);
}
演示apache-dbutils+druid完成dml(update,insert,delete)
public void testDML() throws SQLException {Connection connection = JDBCUtilsByDruid.getConnection();QueryRunner queryRunner = new QueryRunner();String sql = "update actor set name = ? where id = ?";//(I)执行dml操作是queryRunner.update()//(2)返回的值是受影响的行数int affectedRow = queryRunner.update(connection, sql, "张三丰", 1);System.out.println(affectedRow > 0 ? "成功" : "执行没有影响到表");JDBCUtilsByDruid.close(null, null, connection);
}
表和JavaBean的类型映射关系
DAO增删改查-BasicDao
问题
apache-dbutils+Druid简化了JDBC开发,但还有不足:
1.SQL语句是固定,不能通过参数传入,通用性不好,需要进行改进,更方便执行增删改查
2.对于select操作,如果有返回值,返回类型不能固定,需要使用泛型
3.将来的表很多,业务需求复杂,不可能只靠一个Java类完成
4.引出=》BasicDAO画出示意图
基本说明
1.DAO:data access object数据访问对象
2.这样的通用类,称为BasicDao,是专门和数据库交互的,即完成对数据库(表)的crud操作。
3.在BaiscDao的基础上,实现一张表对应一个Dao,更好的完成功能,比如Customer表
Customer.java(javabean)-CustomerDao.java
BasicDAO应用实例
dao包
BasicDAO
package com.hspedu.dao_.dao;import com.hspedu.jdbc.datasource.JDBCUtilsByDruid;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;/*** @program: chapter25* @since: jdk1.8* @description: 开发BasicDAO,是其他DAO的父类* @author: Administrator* @create: 2024-12-15 15:12**/
public class BasicDAO<T> { // 泛型指定具体类型private QueryRunner qr = new QueryRunner();/*** 开发一个通用的DML(数据操作语言)方法,用于执行更新、插入或删除操作,适用于任意表。** @param sql 要执行的SQL语句,通常为UPDATE、INSERT或DELETE语句。* @param parameters SQL语句中的参数值,可变参数,允许传入多个参数。* @return 返回受影响的行数,表示有多少行数据被更新、插入或删除。* @throws RuntimeException 如果在执行SQL语句过程中发生SQL异常,则抛出此运行时异常。*/public int update(String sql, Object... parameters) {Connection connection = null;try {connection = JDBCUtilsByDruid.getConnection();int update = qr.update(connection, sql, parameters);return update;} catch (SQLException e) {throw new RuntimeException(e);} finally {JDBCUtilsByDruid.close(null, null, connection);}}/*** 执行SQL查询并返回多个对象(即查询结果是多行),适用于任意表。** @param sql 要执行的SQL查询语句。* @param clazz 查询结果对象的目标类,必须是一个JavaBean类。* @param parameters SQL查询中的参数值,可变参数,支持传入多个参数。* @return 返回一个包含查询结果的列表,列表中的每个元素都是clazz类型的对象。* 如果查询结果为空,则返回一个空列表。* @throws RuntimeException 如果在查询过程中发生SQL异常,则抛出此运行时异常。*/// 返回多个对象(即查询的结果是多行),针对任意表public List<T> queryMulti(String sql, Class<T> clazz, Object... parameters) {Connection connection = null;try {connection = JDBCUtilsByDruid.getConnection();List<T> list = qr.query(connection, sql, new BeanListHandler<T>(clazz), parameters);return list;} catch (SQLException e) {throw new RuntimeException(e);} finally {JDBCUtilsByDruid.close(null, null, connection);}}/*** 执行SQL查询并返回单个结果对象** @param sql 要执行的SQL查询语句* @param clazz 结果对象的目标类,必须是一个JavaBean类* @param parameters SQL查询中的参数值,可变参数,可以传入多个参数* @return 查询到的单个结果对象,若查询结果为空则返回null* @throws RuntimeException 如果在查询过程中发生SQL异常,则抛出此运行时异常*/public T querySingle(String sql, Class<T> clazz, Object... parameters) {Connection connection = null;try {connection = JDBCUtilsByDruid.getConnection();return qr.query(connection, sql, new BeanHandler<T>(clazz), parameters);} catch (SQLException e) {throw new RuntimeException(e);} finally {JDBCUtilsByDruid.close(null, null, connection);}}/*** 执行SQL查询并返回查询结果的第一行第一列的值。** @param sql 要执行的SQL查询语句。* @param parameters SQL查询中的参数值,可变参数,支持传入多个参数。* @return 返回查询结果的第一行第一列的值,如果查询结果为空,则返回null。* @throws RuntimeException 如果在查询过程中发生SQL异常,则抛出此运行时异常。*/public Object queryScalar(String sql, Object... parameters) {Connection connection = null;try {connection = JDBCUtilsByDruid.getConnection();return qr.query(connection, sql, new ScalarHandler<>(), parameters);} catch (SQLException e) {throw new RuntimeException(e);} finally {JDBCUtilsByDruid.close(null, null, connection);}}
}