鸿蒙开发小案例(名片管理)
- 1、页面效果
- 1.1 初始页面
- 1.2 点击名片展开
- 1.3 点击收藏
- 1.4 点击编辑按钮
- 2、实现代码
- 2.1 DataModel.ets
- 2.2 RandomUtil.ets
- 2.3 ContactList.ets
1、页面效果
1.1 初始页面
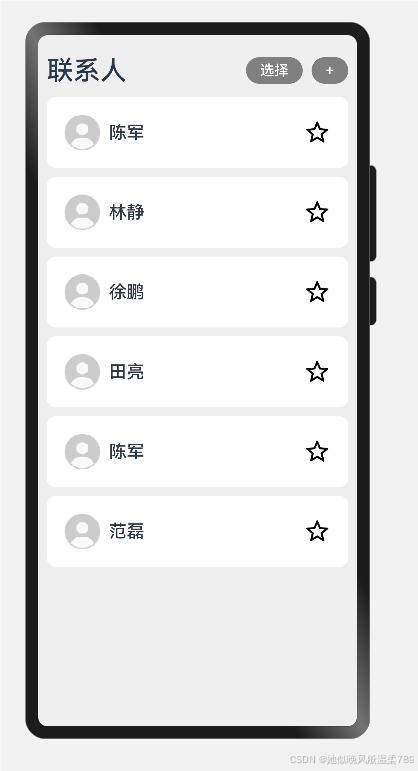
1.2 点击名片展开
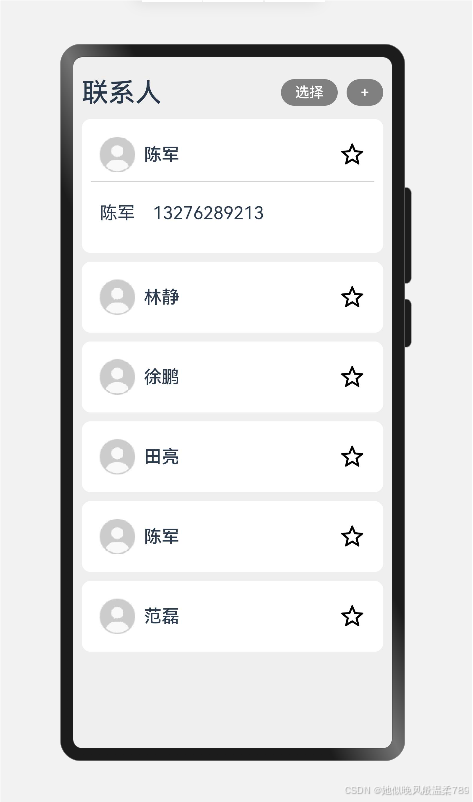
1.3 点击收藏
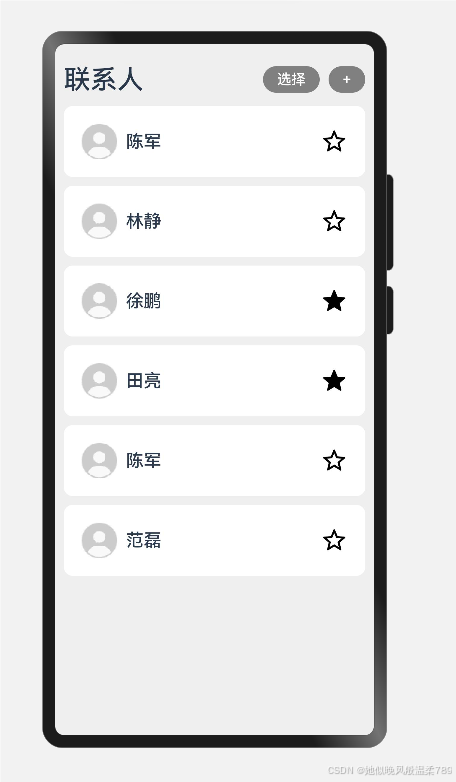
1.4 点击编辑按钮
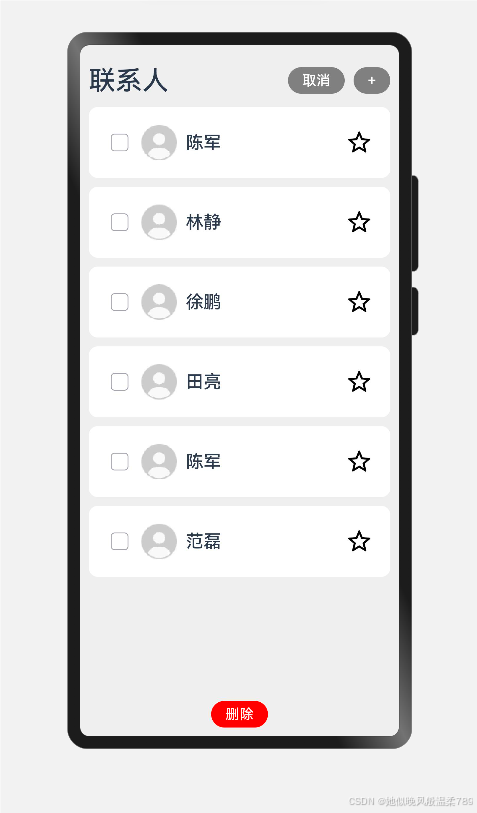
2、实现代码
2.1 DataModel.ets
let nextId = 1;@Observed
export class Person {id: number;name: string;phone: string;isStar: boolean = false;constructor(name, phone) {this.id = nextId++;this.name = name;this.phone = phone;}
}
2.2 RandomUtil.ets
const names = ['张伟','王芳','李娜','刘强','陈军','杨洋','赵丽','黄勇','周雪','吴宇','徐鹏','马丽','孙建','朱敏','郭涛','曹梅','田亮','林静','范磊'
];
export function getRandomName() {let randomIndex = Math.floor(Math.random() * names.length);return names[randomIndex];
}
export function getRandomAge() {return 10 + Math.floor(Math.random() * 30);
}
export function getRandomPhone() {const prefixArray = ['130', '131', '132', '133', '134', '135', '136', '137', '138', '139'];let phone = prefixArray[Math.floor(Math.random() * prefixArray.length)];for (let i = 0; i < 8; i++) {phone += Math.floor(Math.random() * 10);}return phone;
}
2.3 ContactList.ets
import { getRandomName, getRandomPhone } from '../../../../utils/RandomUtil';
import { Person } from './model/DataModel';@Entry
@Component
struct ContactsPage {@State persons: Person[] = [new Person(getRandomName(), getRandomPhone()), new Person(getRandomName(), getRandomPhone())];@State isOpenId: number = -1;@State isShowCheck: boolean = false;@State selectIdList: number[] = [];build() {Column() {Row({ space: 10 }) {Text('联系人').titleStyle()Blank()Button(this.isShowCheck ? '取消' : '选择').buttonStyle(Color.Gray).onClick(() => {this.isShowCheck = !this.isShowCheck})Button('+').buttonStyle(Color.Gray).onClick(() => {this.persons.push(new Person(getRandomName(), getRandomPhone()))})}.width('100%').height(60)List({ space: 10 }) {ForEach(this.persons, (person: Person) => {ListItem() {ContactItem({person: person,isOpenId: $isOpenId,isShowCheck: this.isShowCheck,selectIdList: $selectIdList })}})}.width('100%').layoutWeight(1)if (this.isShowCheck) {Button('删除').buttonStyle(Color.Red).margin({ top: 10 }).onClick(() => {this.persons = this.persons.filter(person => !this.selectIdList.includes(person.id));})}}.width('100%').height('100%').backgroundColor('#EFEFEF').padding(10)}
}@Component
struct ContactItem {@ObjectLink person: Person; @State isShowPhone: boolean = false; @Link @Watch("numberChanger") isOpenId: number; @Prop isShowCheck: boolean; @Link selectIdList: number[] numberChanger() {this.person.id == this.isOpenId ? this.isShowPhone = true : this.isShowPhone = false;}build() {Column() {Row({ space: 10 }) {if (this.isShowCheck) {Toggle({type: ToggleType.Checkbox}).onChange((value) => {value ? this.selectIdList.push(this.person.id) : this.selectIdList.slice(this.selectIdList.indexOf(this.person.id), 1)})}Image($r('app.media.img_user_avatar')).width(40).height(40)Text(this.person.name).fontSize(20).fontWeight(500)Blank()Image(this.person.isStar ? $r('app.media.ic_star_filled') : $r('app.media.ic_star_empty')).width(30).height(30).onClick(() => {this.person.isStar = !this.person.isStar;})}.justifyContent(FlexAlign.SpaceBetween).padding(10).width("100%").onClick(() => {this.isShowPhone = !this.isShowPhone;this.isOpenId = this.person.id;})if (this.isShowPhone) {Divider()Row() {Text(this.person.name).fontSize(20)Text(this.person.phone).fontSize(20).margin({ left: 20 })}.backgroundColor(Color.White).width('100%').height(70).padding(10).borderRadius(10)}}.backgroundColor(Color.White).padding(10).borderRadius(10)}
}@Extend(Text) function titleStyle() {.fontSize(30).fontWeight(500)
}@Extend(Button) function buttonStyle(color: ResourceColor) {.height(30).backgroundColor(color)
}