1、适配器
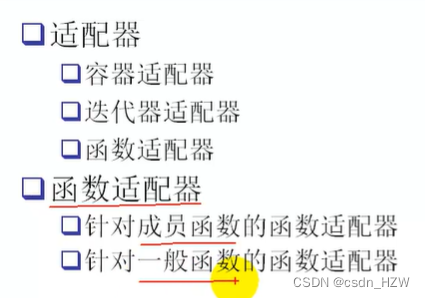
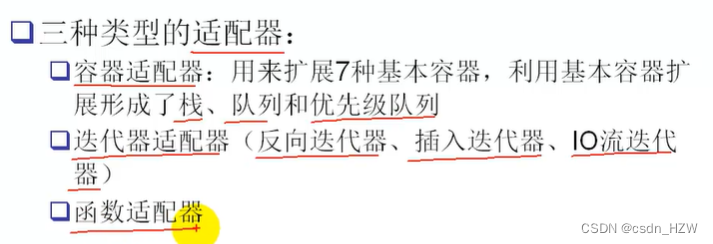
2、函数适配器

#include <iostream>
using namespace std;#include <algorithm>
#include <vector>
#include <functional>bool isOdd(int n)
{return n % 2 == 1;
}
int main() {int a[] = {1, 2, 3, 4, 5};vector <int> v(a, a + 5);cout << count_if(v.begin(), v.end(), isOdd) << endl;// binder2nd将二元函数对象modulus转换为医院函数对象。// binder2nd(op, value)(param)相当于op(param, value)cout << count_if(v.begin(), v.end(), binder2nd(modulus<int>(), 2)) << endl;// binder1st(op, value)(param)相当于op(value, param)cout << count_if(v.begin(), v.end(), binder1st(less<int>(), 4)) << endl;return 0;
}// 输出
3
3
1
3、针对成员函数的函数适配器

#include <iostream>
using namespace std;#include <algorithm>
#include <vector>
#include <functional>
#include <string>class Person
{
public:Person(const string& name) : name_(name){}void print() const{cout << name_ << endl;}void printWithPrefix(string prefix) const{cout << prefix << name_ << endl;}private:string name_;
};void foo(const vector<Person>& v)
{for_each(v.begin(), v.end(), mem_fun_ref(&Person::print));for_each(v.begin(), v.end(), binder2nd(mem_fun_ref(&Person::printWithPrefix), "Person: "));
}void foo2(const vector<Person*>& v)
{for_each(v.begin(), v.end(), mem_fun(&Person::print));for_each(v.begin(), v.end(), binder2nd(mem_fun(&Person::printWithPrefix), "Person: "));
}
int main() {vector<Person> v;v.push_back(Person("tom"));v.push_back(Person("jer"));foo(v);vector<Person*> v2;v2.push_back(new Person("tom"));v2.push_back(new Person("jer"));foo2(v2);return 0;
}// 输出
tom
jer
Person: tom
Person: jer
tom
jer
Person: tom
Person: jer
4、针对一般函数的函数适配器
#include <iostream>
using namespace std;#include <algorithm>
#include <vector>
#include <functional>
#include <string>
#include <cstring>int main() {char* a[] = {"", "BBB", "CCC"};vector<char*> v(a, a + 2);vector<char*>::iterator it;it = find_if(v.begin(), v.end(), binder2nd(ptr_fun(strcmp), ""));if (it != v.end()){cout << *it << endl;}return 0;
}
// 输出
BBB