122. 买卖股票的最佳时机 II - 力扣(LeetCode)
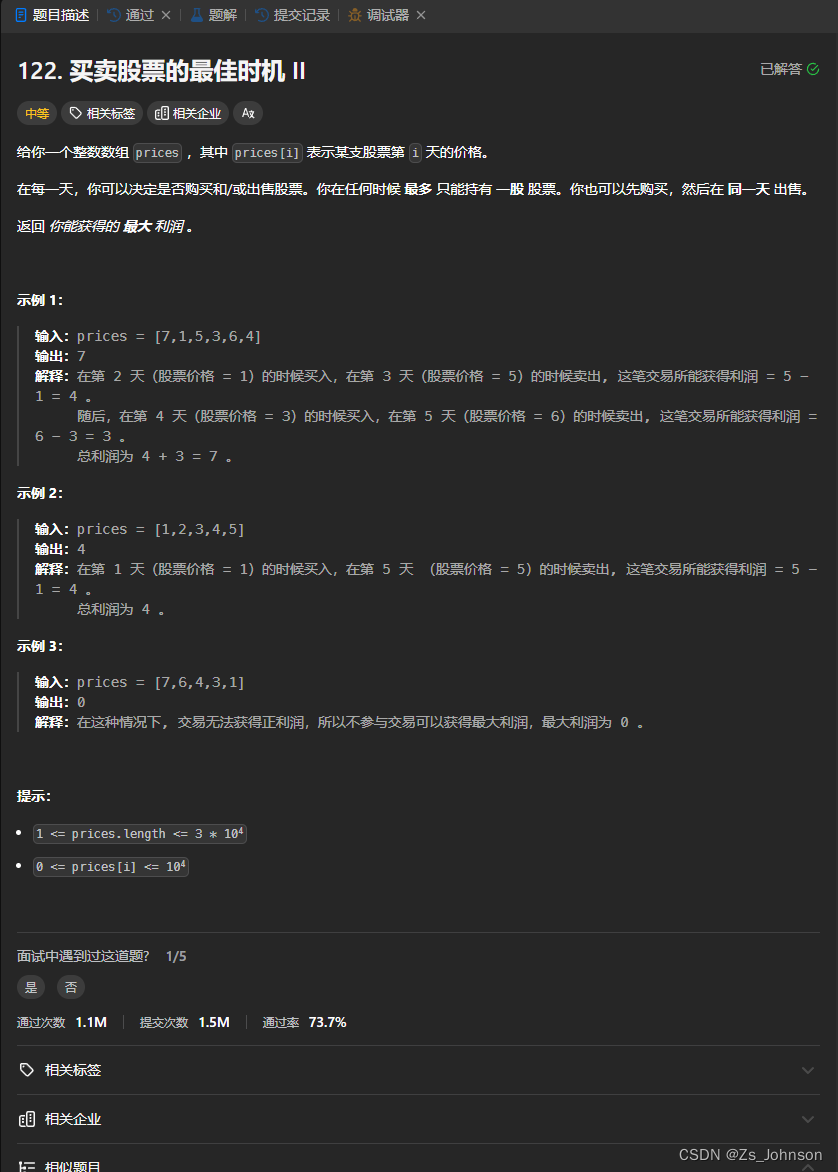
class Solution {public int maxProfit(int[] prices) {if(prices.length == 0){return 0;}int min = prices[0];int result = 0;for(int i=1;i<prices.length;i++){if(prices[i] > min){result += (prices[i] - min);min = prices[i];continue;}if(min > prices[i]){min = prices[i];}}return result;// int buyIndex = -1;// int result = 0;// for (int i = 0; i < prices.length - 1; i++) {// if (prices[i] <= prices[i + 1]) {// if (buyIndex == -1) {// buyIndex = i;// }// continue;// }// if (buyIndex != -1) {// result += prices[i] - prices[buyIndex];// buyIndex = -1;// }// }// if (buyIndex != -1) {// result += prices[prices.length - 1] - prices[buyIndex];// }// return result;}
}
55. 跳跃游戏 - 力扣(LeetCode)
class Solution {public boolean canJump(int[] nums) {if(nums.length <= 1){return true;}int[] canReachPos = new int[nums.length];for(int i=0;i<canReachPos.length;i++){canReachPos[i] = i + nums[i];}int canReachMaxRemotePos = canReachPos[0];for(int i=0;i<=canReachMaxRemotePos;i++){if(canReachPos[i] >= nums.length-1){return true;}if(canReachPos[i] > canReachMaxRemotePos){canReachMaxRemotePos = canReachPos[i];}}return false;}
}
45. 跳跃游戏 II - 力扣(LeetCode)
class Solution {public int jump(int[] nums) {if (nums.length <= 1) {return 0;}int[] dp = new int[nums.length];Arrays.fill(dp, Integer.MAX_VALUE);dp[0] = 0;for (int i = 1; i <= nums[0] && i < nums.length; i++) {dp[i] = 1;}for (int i = 1; i < nums.length; i++) {for (int j = 0; j <= nums[i]; j++) {if (i + j < nums.length && dp[i + j] == Integer.MAX_VALUE) {dp[i + j] = dp[i] + 1;}}if (dp[nums.length - 1] != Integer.MAX_VALUE) {return dp[nums.length - 1];}}return dp[nums.length - 1];}
}