前言
该系统采用SpringBoot+Vue前后端分离开发,前端是一个单独的项目,后端是一个单独的项目。
技术栈:SpringBoot+Vue+Mybatis+Redis+Mysql
开发工具:IDEA、Vscode
浏览器:Chrome
开发环境:JDK1.8、Maven3.3.9、Node 16.6.0、MySql 5.7
一、功能划分
1、用户功能
2、管理员功能
二、页面展示部分
1、注册登录
2、系统首页
3、商品分类页面
4、购物车页面
5、订单页面
6、个人信息页面
7、后台页面
三、系统源码
1、前端
前端项目结构
部分核心代码
<!--* 前台首页 相关内容** @Author: ShanZhu* @Date: 2023-11-11
-->
<template><div><search @search="handleSearch"></search><div class="main-box"><div class="block" style="margin: 10px auto"><!--商品分类--><div class="good-menu"><ul v-for="(item, index) in icons" :key="index"><li><i class="iconfont" v-html="item.value"></i><!--跳转到商品分类对应列表--><span v-for="(category, index2) in item.categories" :key="index2"><router-link:to="{path: '/goodlist',query: { categoryId: category.id },}"><a href="/person"><span style="color: #3186cb">{{ category.name }}</span></a></router-link><span v-if="index2 != item.categories.length - 1">/</span></span></li></ul></div><!--轮播图--><div><el-carousel height="370px" style="border-radius: 20px; width: 600px"><el-carousel-item v-for="carousel in carousels" :key="carousel.id"><router-link :to="'/goodview/' + carousel.goodId"><img style="height: 370px; width: 600px":src="baseApi + carousel.img"/></router-link></el-carousel-item></el-carousel></div></div><!--推荐商品--><div style="margin-top: 30px"><span style="color: #e75c09">推荐商品</span></div><div style="margin: 20px auto"><el-row :gutter="20"><el-col:span="6"v-for="good in good":key="good.id"style="margin-bottom: 20px"><router-link :to="'goodview/' + good.id"><el-card :body-style="{ padding: '0px', background: '#3472a6' }"><img:src="baseApi + good.imgs"style="width: 100%; height: 300px"/><div style="padding: 5px 10px"><!--商品名称--><span style="font-size: 18px; color: #ffffff">{{ good.name }}</span><br/><!--商品价格--><span style="color: #ffffff; font-size: 15px">¥{{ good.price }}</span></div></el-card></router-link></el-col></el-row></div></div></div>
</template><script>
import search from "../../components/Search";
export default {name: "TopView",//页面初始化数据data() {return {//轮播图carousels: [],//推荐商品good: [],baseApi: this.$store.state.baseApi,//分类icon,每个icon包含id、value、categories对象数组.category:id,nameicons: [],//搜索内容searchText: "",baseApi: this.$store.state.baseApi,};},components: {search,},//页面创建created() {this.request.get("/api/good").then((res) => {if (res.code === "200") {this.good = res.data;} else {this.$message.error(res.msg);}});this.request.get("/api/icon").then((res) => {if (res.code === "200") {this.icons = res.data;if(this.icons.length > 6) {// 截取前六个分类this.icons = this.icons.slice(0, 6);}}});this.request.get("/api/carousel").then((res) => {if (res.code === "200") {this.carousels = res.data;}});},//方法methods: {//搜索按钮触发handleSearch(text) {this.searchText = text;this.$router.push({path: "/goodList",query: { searchText: this.searchText },});},},
};</script><style scoped>
.main-box {background-color: white;border: white 2px solid;border-radius: 40px;padding: 20px 40px;margin: 5px auto;
}
.good-menu {float: left;height: 370px;margin-right: 130px;
}
.good-menu li {list-style: none;overflow: hidden;margin-bottom: 35px;
}
.good-menu li a,
span {font-size: 20px;color: #6c6969;
}
.good-menu a span:hover {color: #00b7ff;
}
</style>
2、后端
项目结构
核心代码
/*** 商品 控制层** @author: ShanZhu* @date: 2023-11-10*/
@RestController
@RequestMapping("/api/good")
@RequiredArgsConstructor
public class GoodController {private final GoodService goodService;private final StandardService standardService;/*** 查询商品** @param id 商品id* @return 商品*/@GetMapping("/{id}")public R<Good> findGood(@PathVariable Long id) {return R.success(goodService.getGoodById(id));}/*** 查询商品规格** @param id 商品规格id* @return 商品规格*/@GetMapping("/standard/{id}")public R<String> getStandard(@PathVariable Integer id) {return R.success(goodService.getStandard(id));}/*** 查询推荐商品,即recommend=1** @return 商品*/@GetMappingpublic R<GoodVo> findFrontGoods() {return R.success(goodService.findFrontGoods());}/*** 商品销售额排行** @return 商品*/@GetMapping("/rank")public R<List<Good>> getSaleRank(@RequestParam int num) {return R.success(goodService.getSaleRank(num));}/*** 保存商品** @param good 商品信息* @return 商品id*/@PostMappingpublic R<Long> save(@RequestBody Good good) {return R.success(goodService.saveOrUpdateGood(good));}/*** 更新商品** @param good 商品信息*/@PutMappingpublic R<Void> update(@RequestBody Good good) {goodService.update(good);return R.success();}/*** 删除商品** @param id 商品id*/@DeleteMapping("/{id}")public R<Void> delete(@PathVariable Long id) {goodService.loginDeleteGood(id);return R.success();}/*** 保存商品规格** @param standards 商品规格列表* @param goodId 商品id*/@PostMapping("/standard")public R<Void> saveStandard(@RequestBody List<Standard> standards,@RequestParam int goodId) {//先删除全部旧记录standardService.deleteAll(goodId);//然后插入新记录for (Standard standard : standards) {standard.setGoodId(goodId);if (!standardService.save(standard)) {return R.error(Status.CODE_500, "商品id: " + goodId + " ,规格保存失败");}}return R.success();}/*** 删除商品规格** @param standard 商品规格列表*/@DeleteMapping("/standard")public R<Void> delStandard(@RequestBody Standard standard) {if (BooleanUtil.isTrue(standardService.delete(standard))) {return R.success();} else {return R.error(Status.CODE_500, "删除失败");}}/*** 修改商品推荐** @param id 商品id* @param isRecommend 是否推荐* @return 结果*/@GetMapping("/recommend")public R<Void> setRecommend(@RequestParam Long id,@RequestParam Boolean isRecommend) {return R.success(goodService.setRecommend(id, isRecommend));}/*** 分页查询商品 - 带查询条件** @param pageNum 页数* @param pageSize 分页大学* @param searchText 查询文本* @param categoryId 分类id* @return 商品列表*/@GetMapping("/page")public R<IPage<GoodVo>> findGoodPage(@RequestParam(required = false, defaultValue = "1") Integer pageNum,@RequestParam(required = false, defaultValue = "10") Integer pageSize,@RequestParam(required = false, defaultValue = "") String searchText,@RequestParam(required = false) Integer categoryId) {return R.success(goodService.findPage(pageNum, pageSize, searchText, categoryId));}/*** 分页查询全部商品** @param pageNum 页数* @param pageSize 分页大学* @param searchText 查询文本* @param categoryId 分类id* @return 商品列表*/@GetMapping("/fullPage")public R<IPage<GoodVo>> findGoodFullPage(@RequestParam(required = false, defaultValue = "1") Integer pageNum,@RequestParam(required = false, defaultValue = "10") Integer pageSize,@RequestParam(required = false, defaultValue = "") String searchText,@RequestParam(required = false) Integer categoryId) {return R.success(goodService.findFullPage(pageNum, pageSize, searchText, categoryId));}}
四、系统源码+答辩PPT
系统源码链接地址:仅供参考学习。
公众号:热爱技术的小郑。
回复:商城系统,即可获取到系统源码。
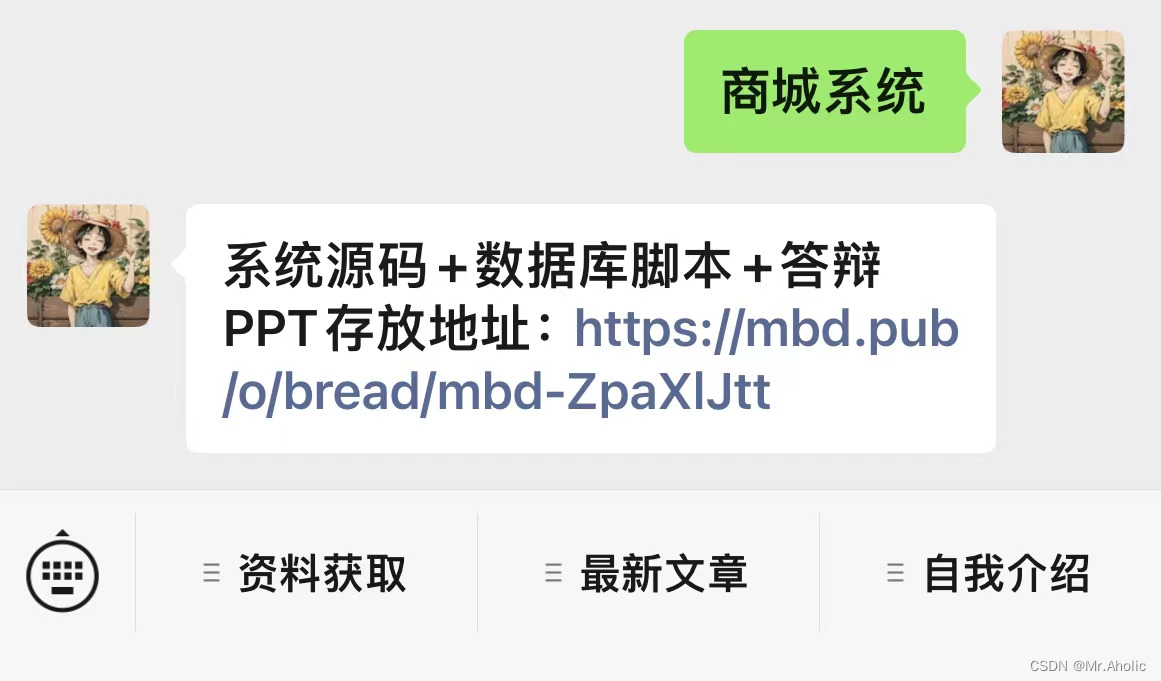