一、效果图
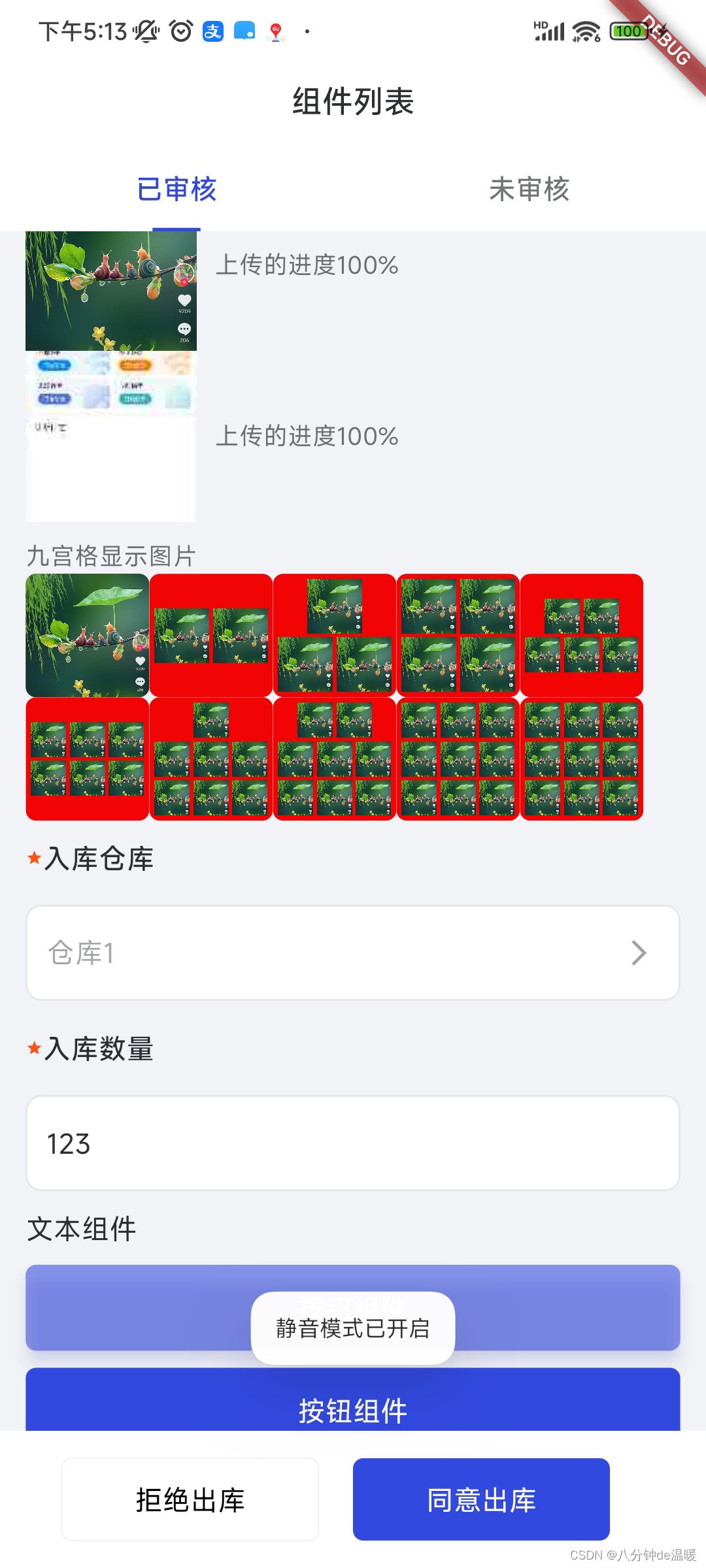
2、主要代码
import 'dart:io';
import 'dart:math';import 'package:cached_network_image/cached_network_image.dart';
import 'package:flutter/material.dart';class ImageGrid extends StatelessWidget {final List<String> imageUrls; final double containerSize = 72.0; final double padding = 3.0; final double spacing = 2.0; ImageGrid({required this.imageUrls});double calculateImageWidth(int count) {double containerWidth = 72;double padding = 3;double gap = 2;if (count <= 4) {return (containerWidth - padding * 2 - gap) / 2;} else {return (containerWidth - padding * 2 - gap * 2) / 3;}}Widget _buildGrid() {double imageWidth = calculateImageWidth(imageUrls.length);int imageCount = imageUrls.length > 9 ? 9 : imageUrls.length;if (imageCount == 1) {return Center(child: Container(width: containerSize,height: containerSize,child: Image.network(imageUrls[0],fit: BoxFit.cover,),),);} else {int rowCount = 1; int firstNumber = 0; int cellCount = 1; if (imageCount == 2) {rowCount = 1;cellCount = 2;} else if (imageCount == 4 || imageCount == 6) {rowCount = 2;cellCount = imageCount == 4 ? 2 : 3;} else if (imageCount == 9) {rowCount = 3;cellCount = 3;} else if (imageCount == 3) {rowCount = 2;firstNumber = 1;cellCount = 2;} else if (imageCount == 5) {rowCount = 2;firstNumber = 2;cellCount = 3;} else if (imageCount == 7) {rowCount = 3;firstNumber = 1;cellCount = 3;} else if (imageCount == 8) {rowCount = 3;firstNumber = 2;cellCount = 3;}return Padding(padding: EdgeInsets.all(spacing),child: Column(mainAxisAlignment: MainAxisAlignment.center,crossAxisAlignment: CrossAxisAlignment.center,children: [if (firstNumber != 0)Row(mainAxisAlignment: MainAxisAlignment.center,children: List.generate(firstNumber, (cellIndex) {return Row(children: [getImageWidget(cellIndex, imageWidth),if (cellIndex != firstNumber - 1)SizedBox(width: spacing),],);}),),if (firstNumber != 0 && rowCount > 1) SizedBox(height: spacing),...List.generate(rowCount - (firstNumber != 0 ? 1 : 0), (rowIndex) {return Column(children: [Row(mainAxisAlignment: MainAxisAlignment.center,children: List.generate(cellCount, (cellIndex) {int index =firstNumber + rowIndex * cellCount + cellIndex;return Row(children: [getImageWidget(index, imageWidth),if (cellIndex != cellCount - 1)SizedBox(width: spacing),],);}),),if (rowIndex != rowCount - (firstNumber != 0 ? 2 : 1))SizedBox(height: spacing),],);}),],),);}}getImageWidget(int index, double width) {if (!imageUrls[index].contains("http")) {return Image.file(File(imageUrls[index]),width: width, height: width, fit: BoxFit.cover);} else {return CachedNetworkImage(imageUrl: imageUrls[index],width: width,height: width,fit: BoxFit.cover,);}}@overrideWidget build(BuildContext context) {return ClipRRect(borderRadius: BorderRadius.circular(6),child: Container(width: containerSize,height: containerSize,decoration: BoxDecoration(color: Color.fromARGB(255, 243, 4, 4),borderRadius: BorderRadius.circular(6),),child: _buildGrid(),),);}
}